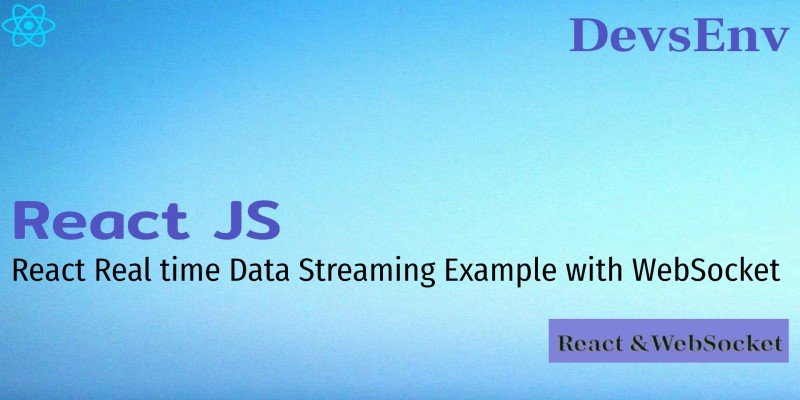
Connect your React app with WebSocket to get real time Data
Today we'll start how to create a react app with live data from a websocket server.
Start a basic react project:
Create a basic react project names websocketdemo
. That means we'll use basic Create CRA App.
npx create-react-app websocketdemo
Then go to that folder using cd websocketdemo
.
Open that project using your favorite Text Editor. In my case, that is Visual Studio Code.
Run Basic React Project First:
Run your project by giving command npm start
. Then it will start in your localhost 3000 port. It's the default running port of any react application.
Run this URL in browser - http://localhost:3000/
and you'll see a fresh react project set up and it's time to get Websocket in our React App.
Make Design For a Chat Application (Just Demo):
Now create a component inside src/components folder named WebSocketComponent.
Full path will be src/components/WebSocketComponent.js
The Component full source code is:
import React, { Component } from "react";
class WebSocketComponent extends Component {
state = {
messageList: [
{ message: "Hello Akash" },
{ message: "Hi Man, what about you ?" },
{ message: "Yes, what about you too ?" }
]
};
render() {
return (
<div className="message-area">
<ul className="list">
{this.state.messageList.map((item, index) => (
<li
className={index % 2 === 0 ? "list-item-left" : "list-item-right"}
key={index}
>
{item.message}
</li>
))}
</ul>
</div>
);
}
}
export default WebSocketComponent;
What have done:
We've used React class based component. in state, we've defined a messageList
array. Which contains some dummy messages.
messageList: [
{ message: "Hello Akash" },
{ message: "Hi Man, what about you ?" },
{ message: "Yes, what about you too ?" }
]
And in render()
, we've just print it using map()
function of JavaScript. and we've also added a logic if the message is even, we make it list-item-left
class or list-item-right
class if it is in the right.
<ul className="list">
{this.state.messageList.map((item, index) => (
<li className={index % 2 === 0 ? "list-item-left" : "list-item-right"} key={index}>
{item.message}
</li>
))}
</ul>
Ok, now clear src/app.js
file and add that component in src/app.js
file.
import React from "react";
import logo from "./logo.svg";
import "./App.css";
import WebSocketComponent from "./components/WebSocketComponent";
function App() {
return (
<div className="App">
<img src={logo} alt="React Websocket" width={100} />
<WebSocketComponent />
</div>
);
}
export default App;
Let's give it some styles.
add chat-list.css in src/components
folder.
.list {
list-style: none;
margin: 20px;
}
.list-item-left {
background: #1552fac2;
padding: 10px;
border-radius: 10px;
border-bottom: 1px solid;
color: #FFF!important;
text-align: left!important;
margin-bottom: 3px;
}
.list-item-right {
background: #eee;
padding: 10px;
border-radius: 10px;
border-bottom: 1px solid;
text-align: right!important;
margin-bottom: 3px;
}
add that css in the src/components/WebSocketComponent.js
file
import "./chat-list.css";
Now, see the output again:
Ok, now import react-websocket from https://www.npmjs.com/package/react-websocket
npm i react-websocket
And then Update our WebSocketComponent file to listen websocket stream data using like this:
//import at top
import Websocket from "react-websocket";
// Inside render()
<Websocket
url="ws://127.0.0.1:9999/?topic=test&consumerGroup=group1&offset=1"
onMessage={() => {
console.log("hello from websocket data");
}}
/>
Where url=ws://127.0.0.1:9999/?topic=test&consumerGroup=group1&offset=1
is the websocket url, in your case, that will be your websocket url.
So, now whenever any message comes from your websocket live streaming URL, you'll get a console text of "hello from websocket data".
Ok, Now make a function to handle when some data arrives and push the data to messageList array of state.
/**
* handleData
* Manage data when data received from websocket
*/
handleData = messageData => {
const data = JSON.parse(messageData);
let messageList = this.state.messageList;
if (data.length > 0) {
messageList.push(data[0]);
this.setState({
messageList
});
}
};
and add it in view:
<Websocket
url="ws://127.0.0.1:9999/?topic=test&consumerGroup=group1&offset=1"
onMessage={this.handleData}
/>
So, now, whenever a new message arrived from the websocket url, a message will be got and that will be parsed and pushed to the messageList
array. Here is the Full Source Code of WebSocketComponent
.
import React, { Component } from "react";
import Websocket from "react-websocket";
import "./chat-list.css";
class WebSocketComponent extends Component {
state = {
messageList: [
{ message: "Hello Akash" },
{ message: "Hi Man, what about you ?" },
{ message: "Yes, what about you too ?" }
]
};
/**
* handleData
* Manage data when data received from websocket
*/
handleData = messageData => {
const data = JSON.parse(messageData);
let messageList = this.state.messageList;
if (data.length > 0) {
messageList.push(data[0]);
this.setState({
messageList
});
}
};
render() {
return (
<div className="message-area">
<ul className="list">
{this.state.messageList.map((item, index) => (
<li
className={index % 2 === 0 ? "list-item-left" : "list-item-right"}
key={index}
>
{item.message}
</li>
))}
</ul>
<Websocket
url="ws://127.0.0.1:9999/?topic=test&consumerGroup=group1&offset=1"
onMessage={this.handleData}
/>
</div>
);
}
}
export default WebSocketComponent;
Final View after message from websocket:
Download or clone Full Project from Gitlab - Download/Clone Now
PHP If-else-elseif and Switch-case
PHP String Functions - All necessary String functions in PHP to manage strings better.
Popular Tutorials
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
14
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development