
PHP
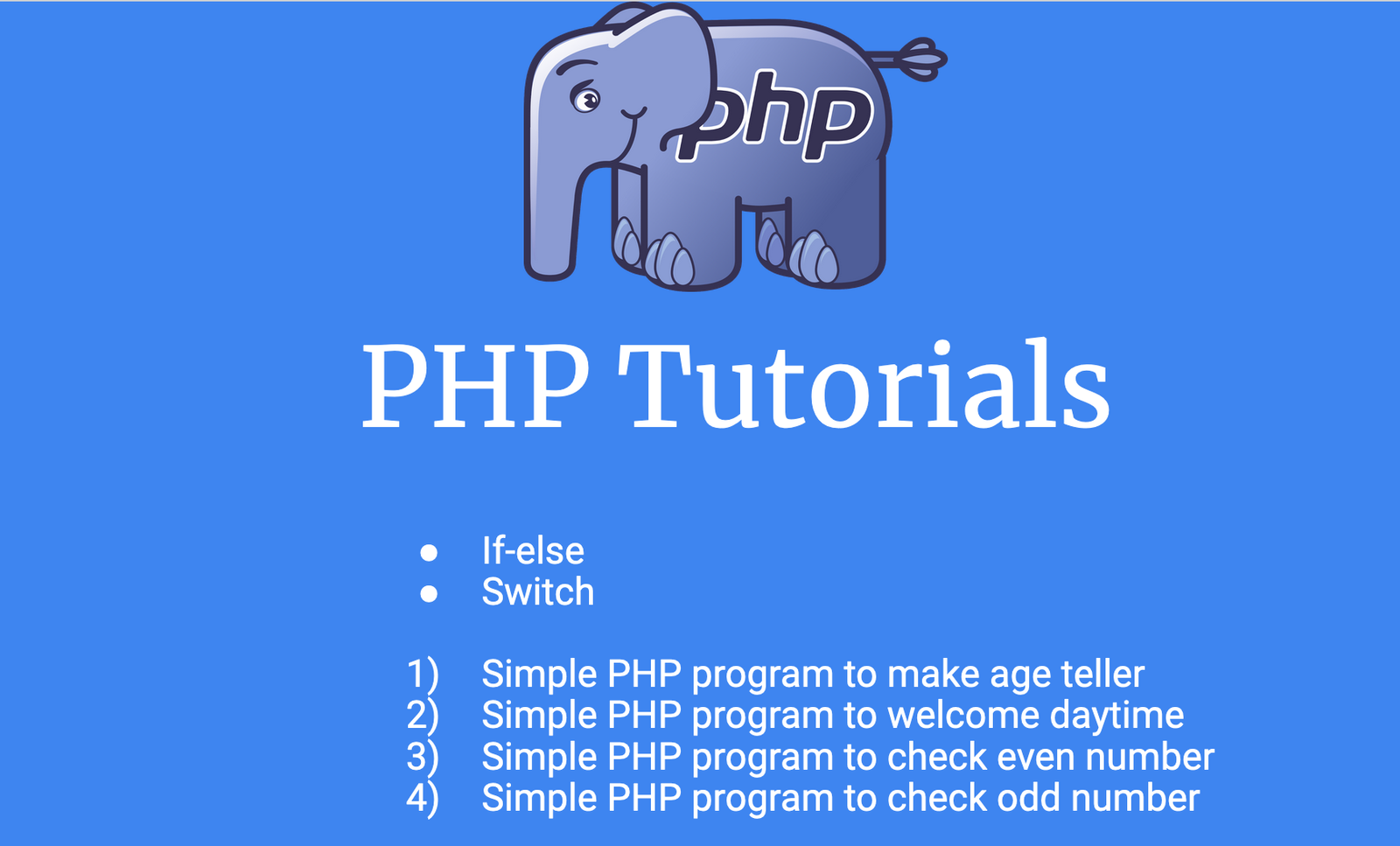
PHP If-else-elseif and Switch-case
¶What is if
in programming language.
if
is a very important feature of any programming language. It’s used to execute conditional statement based on any condition. In PHP, here is the structure of an if-statement.
if (condition or any expression that returns boolean(true or 1 or false or 0)) {
// This will be executed, if condition equal to true
}
¶Example of an if-statement:
<?php
$age = 28;
if ($age > 18) {
echo "You are an Adult !";
}
?>
Output:
¶if-else
If we want to do something if the first condition does not met, then we can use else
like so -
if (condition) {
// This will be executed, if condition equal to true
} else {
// This will be executed, if condition is false
}
¶Example of an if-else statement:
<?php
$age = 15;
if ($age > 18) {
echo "You are an Adult !";
} else {
echo "You are a child !";
}
?>
Output:
¶if-elseif-else
If we want to check multiple condition, not only a single condition check, then we can use elseif
like so -
if (condition) {
// This will be executed, if condition equal to true
} elseif (other_condition) {
// This will be executed, if first condition is false and this condition is true
} else {
// This will be executed, if all above condition is false
}
¶Example of an if-elseif-else
statement:
<?php
$age = 25;
if ($age >= 50) {
echo "You are Old !";
} elseif ($age >= 18) {
echo "You are an Adult !";
} else {
echo "You are a child !";
}
?>
Output:
¶Switch-case
In PHP or any programming language, we can use switch-case
instead of if-else
. It can do same work like if-else
. Main benifits and purpose of switch-case is we can do operations on same variable multiple times.
Let’s see how switch-case
works -
switch (variable) {
case value1:
// execute when variable=value1;
break;
case value2:
// execute when variable=value2;
break;
case value3:
// execute when variable=value3;
break;
default:
// execute when nothing matches
break;
}
If there is multiple case for one condition, then we can write also like this -
case value1:
case value2:
// execute when variable=value1 and variable=value2;
break;
¶Example of switch-case
: Check if a character is Vowel or consonent with the help of switch-case and if-else.
<?php
$character = 'a';
$vowel = false;
switch($character) {
case 'a':
case 'A':
$vowel = true;
break;
case 'e':
case 'E':
$vowel = true;
break;
case 'i':
case 'I':
$vowel = true;
break;
case 'o':
case 'O':
$vowel = true;
break;
case 'u':
case 'U':
$vowel = true;
break;
default:
$vowel = false;
break;
}
if ($vowel === true) {
echo "The character is a Vowel";
} else {
echo "The character is a consonent";
}
Output:
¶More example related to if-else
- Simple PHP program to make age teller
- Simple PHP program to welcome daytime
- Simple PHP program to check even number
- Simple PHP program to check odd number
PHP Operators Part 2 - Comparison Operator, Logical Operator, Conditional Operator
PHP Math Functions - pi, abs, sqrt, min, max, rand, round, floor, ceil, pow, is_nan, is_numeric
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development