
PHP
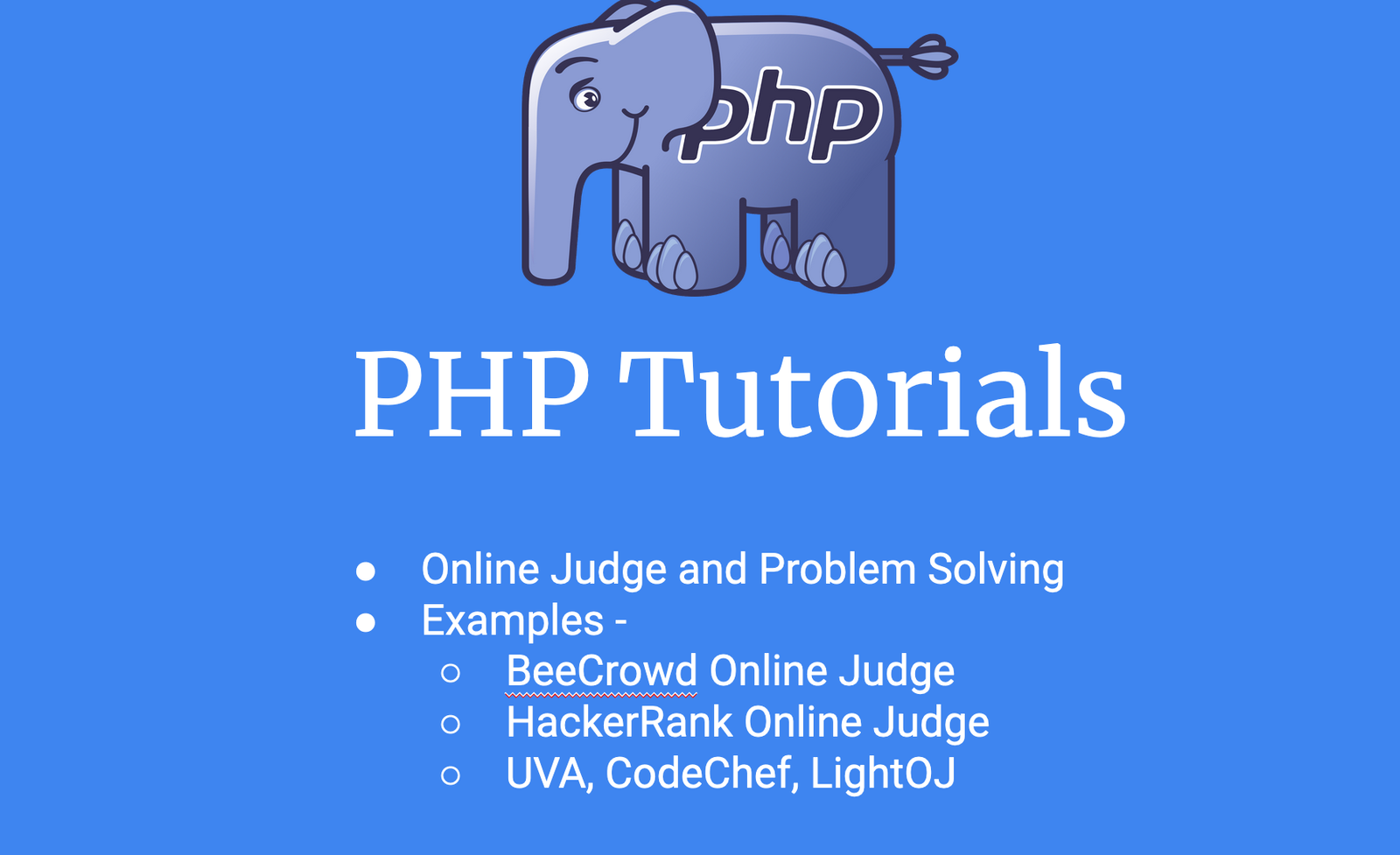
PHP Bonus - Online Judge Problem Solving Using PHP
¶Problem Solving
Todays, lecture is totally different from PHP programming language aspects. This is for both PHP and all general level programming languages. Problem Solving is a very important parts to becoming a very good Developer. In our practical life, we’ll face lots of different and new problems every day when we starts our real project. If we have proper problem solving skills, then we can easily solve any problem easily. Let’s try solving some of the problems in popular online judge programming platform.
¶Popular online problem solving platforms -
- BeeCrowd - https://www.beecrowd.com.br/judge/en
- UVA Online Judge - https://onlinejudge.org
- HackerRank Online Judge - https://www.hackerrank.com
- CodeForce Online Judge - https://codeforces.com
- LightOJ - https://lightoj.com
And there is so many online programming problem solving websites out there. We’ll start using the easiest one BeeCrowd or previously URI online judge.
¶BeeCrowd Online Judge
BeeCrowd is one of the most popular online judge problem solving platform. It’s suitable for both beginner, intermediate and advanced level users.
¶How we’ll solve the problems ?
We’ll read the problems very carefully and solve those problems using PHP language. You can try with other language also if you’re comfortable. We’ll solve some Beginner Level problems - https://www.beecrowd.com.br/judge/en/problems/index/1
¶Test in Online PHP Code -
https://onecompiler.com/php/3y5ak79p9
¶Problem 1: Hello World
https://www.beecrowd.com.br/judge/en/problems/view/1000
¶Problem Details
Hello World!
Jean Bez, beecrowd Brasil
Welcome to beecrowd!
Your first program in any programming language is usually "Hello World!". In this first problem all you have to do is print this message on the screen.
Input
This problem has no input.
Output
You must print the message Hello World! and then the endline as shown below.
Input Sample | Output Sample |
|
Hello World! |
¶Solution in PHP
<?php
echo "Hello World!\n";
?>
¶Problem 2: Extremely Basic
https://www.beecrowd.com.br/judge/en/problems/view/1001
¶Solution in PHP
<?php
$input1 = intval(fgets(STDIN));
$input2 = intval(fgets(STDIN));
$summation = $input1 + $input2;
echo "X = $summation\n";
?>
¶Problem 3 - Multiples
https://www.beecrowd.com.br/judge/en/problems/view/1044
¶Solution in PHP
<?php
$numbers = fgets(STDIN);
$a = intval(explode(" ", $numbers)[0]);
$b = intval(explode(" ", $numbers)[1]);
if (($b % $a == 0) || ($a % $b == 0)) {
echo "Sao Multiplos\n";
} else {
echo "Nao sao Multiplos\n";
}
?>
¶Problem 4 - Upto Odd Numbers
https://www.beecrowd.com.br/judge/en/problems/view/1067
¶Solution in PHP
<?php
$x = intval(fgets(STDIN));
for($i = 1; $i <= $x; $i += 2) {
echo $i . "\n";
}
?>
PHP Array Part 2 - Array Built in PHP Functions and Some More Examples
PHP - Functions in PHP - How to create and how to use
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
13
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development