
PHP
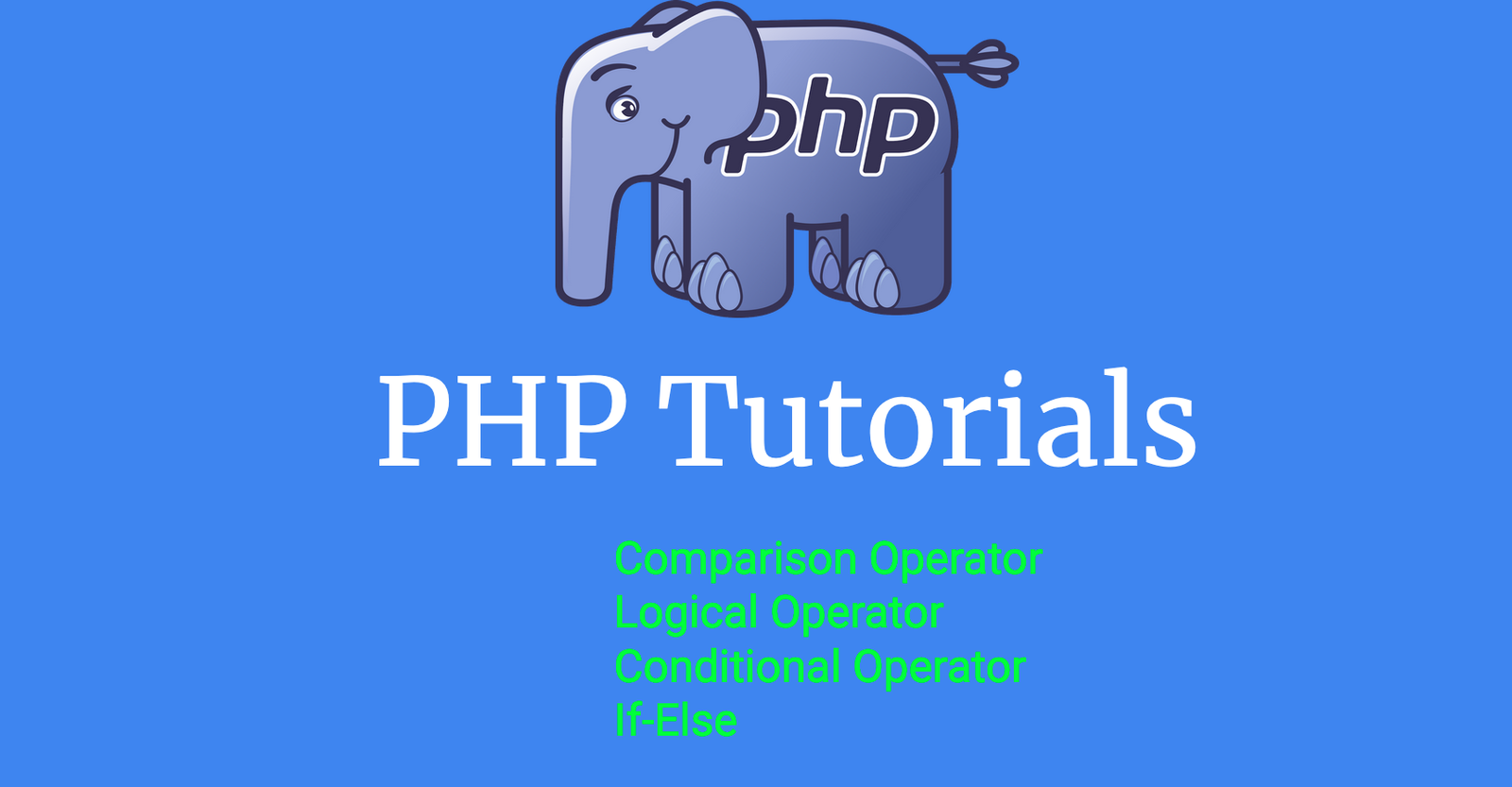
PHP Operators Part 2 - Comparison Operator, Logical Operator, Conditional Operator
Today, we’ll learn learn some of the operators which was not discussed in previous lecture. This will be helpful for our next if-else-elseif
lecture.
- Comparison Operator
- Logical Operator
- Conditional Operator
¶Comparison Operator
Comparison Operator are used to compare two values. Let’s get the list of Comparison operators -
Operator | Name | Example | Result |
---|---|---|---|
== | Equal | $a == $b |
Return true if $a and $b 's value are equal. |
=== | Identical (Triple Equal) | $a === $b |
Return true if $a and $b are identical with case sensativity |
!= | Not Equal | $a != $b |
Return true if $a and $b 's value are not equal. |
!== | Not Identical (Not Triple Equal) | $a !== $b |
Return true if $a and $b are not identical with case sensativity |
<> | Not Equal | $a <> $b |
Return true if $a and $b 's value are not equal. |
< | Less than | $a < $b |
Return true if $a is less than $b |
> | Greater than | $a > $b |
Return true if $a is greater than $b |
<= | Less than or Equal | $a <= $b |
Return true if $a is less than or equal to $b |
>= | Greater than or Equal | $a >= $b |
Return true if $a is greater than or equal to $b |
<=> | Spaceship or Three way comparison operator | $a <=> $b |
Three values => -1, 0, 1 . Return 0 if both equal, 1 if Left is greater, -1 if right is greater. |
¶Examples of Comparison Operator
<?php
$number1 = 10;
$number2 = 20;
var_dump($number1 > $number2); // bool(false)
$number1 = 10;
$number2 = 10;
var_dump($number1 >= $number2); // bool(true)
$number1 = 10;
$number2 = 5;
var_dump($number1 < $number2); // bool(false)
$number1 = 10;
$number2 = 5;
var_dump($number1 <=> $number2); // int(1)
$number1 = 10;
$number2 = 10;
var_dump($number1 <=> $number2); // int(0)
$number1 = 10;
$number2 = 50;
var_dump($number1 <=> $number2); // int(-1)
?>
¶Logical Operator
It’s some logical operations operator or Circuits operators. This only works on boolean (1, 0) values and return type will also be boolean. Let’s get the list of Logical operators -
Operator | Name | Example | Result |
---|---|---|---|
and |
And Operator | $a and $b |
Return true if $a and $b both are true |
or |
Or Operator | $a or $b |
Return true if $a or $b any of them are true |
xor |
Or Operator | $a xor $b |
Return true either $a or $b is true, but not both. |
! |
Not Operator | !$a |
Return true if $a is false. |
&& |
And Operator | $a && $b |
Return true if $a and $b both are true |
|| |
Or Operator | $a && $b |
Return true if $a or $b any of them are true |
¶Example of Logical Operator
<?php
$a = true;
$b = false;
var_dump($a and $b); // bool(false)
var_dump($a or $b); // bool(true)
var_dump($a xor $b); // bool(true)
var_dump($a and $b); // bool(false)
var_dump(!$b); // bool(true)
var_dump($a && $b); // bool(false)
var_dump($a || $b); // bool(true)
?>
¶Conditional Operator
Conditional Operator is for checking some condition -
Operator | Name | Example | Result |
---|---|---|---|
?: |
Ternary Operator | $a = condition ? result1 : result2 |
Return condition is true, return $result1 else return $result2 |
?? [Supported from PHP7.x] |
Null coalescing Operator | $a = result1 ?? result2 |
Return $result1 if it is exist and not null, otherwise return $result2 |
¶Example of Conditional Operator
<?php
$a = true;
$result = $a ? "A is true" : "A is false";
echo $result . '<br>'; // A is true
$val = null;
echo $val ?? "Value is not set"; // Value is not set
echo '<br>';
$val = "Hi, Nice val.";
echo $val ?? "Value is not set"; // Hi, Nice val.
?>
PHP Operators - Arithmetic operator, Assignment Operator, Increment-decrement Operator
PHP If-else-elseif and Switch-case
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development