
PHP
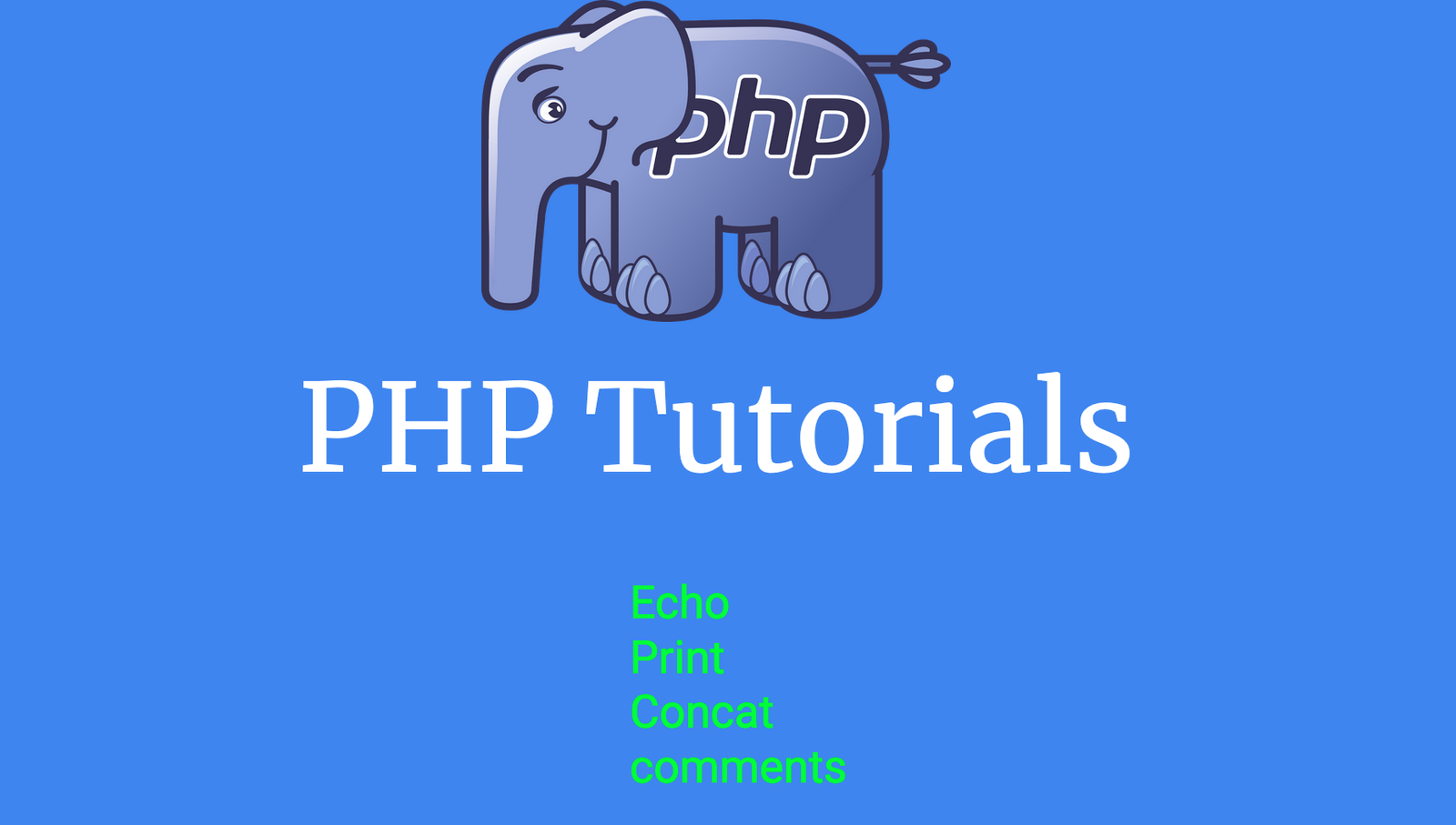
Echo, PHP Statement, Print, Concat and Comments in PHP
In Previous chapter, we’ve learned about variable and it’s data types. Today, we’ll learn also some important thing in PHP programming language.
- echo
- print &
- commenting in PHP code
¶echo
In PHP, to output something to display/console/browser, we can use echo
or print
. So, echo is used to print / output something in browser.
Let’s we want to show Welcome to learn PHP
, so that’s simple -
<?php
echo "Welcome to learn PHP";
?>
Output:
¶Echo/Print Variable in PHP
¶Example 1:
Suppose, we’ve a $price
variable and it’s value is 10 and we want to print that price -
<?php
$price = 10;
echo $price;
?>
Output:
¶Example 2:
Suppose, we’ve a $name
variable and it’s value is Jhon
and we want to print that name with Hello Jhon
-
<?php
$name = "Jhon";
echo "Hello " . $name;
?>
Output:
Note: In the above example, we’ve added a dot (.)
sign after "Hello "
string. This is called Concat
or adding in the aspect of PHP programming language.
In our, next examples, we’ll use this echo and concat a lot for our every example.
¶Example 3:
Suppose, we’ve two variables $number1
and $number2
and we want to print the summation after keeping those in a $summation
variable -
<?php
$number1 = 10;
$number2 = 20;
$summation = $number1 + $number2;
echo "Summation = " . $summation;
?>
Output:
Wow, great, we’ve made a simple summation calculator using this kind of PHP knowledge. So, we can print - text, variable, concat two strings or variables and many more.
¶Example 4: echo()
We can also use echo()
instead of echo
. But echo
is preferred.
<?php
$number1 = 10;
$number2 = 20;
$summation = $number1 + $number2;
echo ("Summation = " . $summation);
?>
Output:
¶print & Difference between echo and print
print
and echo
are almost same. print
is also used for output in PHP programming. Only difference between them is -
-
echo
has no return value -
print
has a return value of1
So, what we’ve done using echo
, we can do that using print
. Let’s do the first example with print
-
<?php
print "Welcome to learn PHP";
?>
Output:
And rest of the examples are also valid for print
in PHP.
¶comment
In our HTML class, we seen how to comment in HTML. That is like -
<!-- HTML Comment & it'll not be visible in Browser -->
In PHP, there is also a commenting system in Code. There are some approach for commenting in PHP -
- Inline commenting -
// Comment after this Hash sign
. Use//
sign and after that add our comment. - Multiline commenting -
/* Comment inside here */
¶1. Inline commenting - // Comment
Let’s add a comment in our first example of what it’ll do -
<?php
print "Welcome to learn PHP"; // This will print Welcome to learn PHP
?>
¶2. Multi-line commenting - /* Comment */
<?php
$number1 = 10; // Assign number1 to 10
$number2 = 20; // Assign number2 to 20
/*
* Let's make summation of those two number.
* By adding those two number.
* Summation = number 1 + number 2;
*/
$summation = $number1 + $number2;
echo ("Summation = " . $summation);
?>
Output:
¶PHP Statement
I think, you’ve already looked, we use semicolon ;
after every line in our above examples. In PHP, to terminate any statement, we use semicolon - ;
. So, after any print
or echo
we must have to use semicolon as, the statement finishes after an echo or print.
<?php
$number1 = 10;
$number2 = 20;
$summation = $number1 + $number2;
echo "Number1 = " . $number1 . "<br>";
echo "Number2 = " . $number2. "<br>";
echo "Summation = " . $summation;
?>
Output:
Number2 = 20
Summation = 30
Data Types in PHP - Variable Types in PHP
PHP Operators - Arithmetic operator, Assignment Operator, Increment-decrement Operator
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development