
PHP
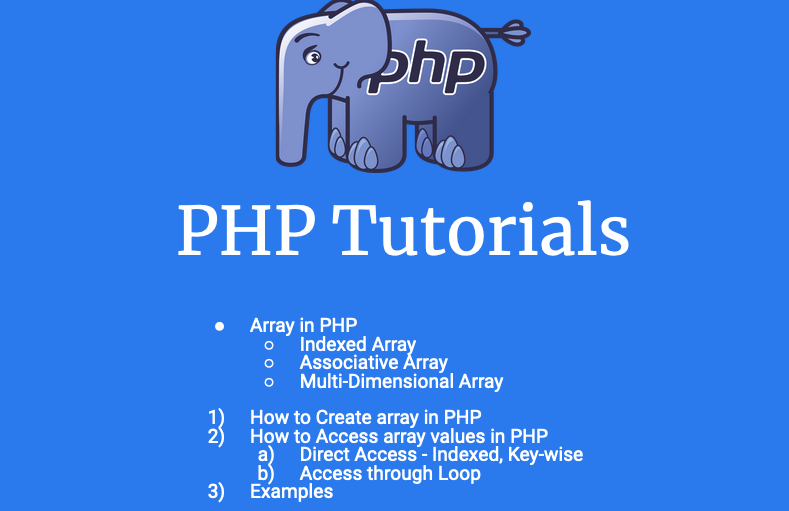
PHP Array and all it's Examples
- What is Array in PHP
- How to create an array in PHP
- Types of Array in PHP
- How to access array property
¶What is Array in PHP
In our Data Types tutorial, we’ve shown, array is one kind of data type for almost any programming language. PHP also has that. Array is a kind of Data-Structure which allows to store multiple values in a single variable. We can store collection of data or similar types of multiple data inside array.
¶Practical Example 1:
In a class, there could be 40 students, or collection of students. Which students have almost same type of data but different. Like, one age, class roll no, father mother etc. So, every students have this kind of similar data. So, array is a good fit for this kind of data processing.
¶Practical Example 2:
In road there are three type of traffic lights. Red, Green and Yellow. List of traffic lights with a different meaning. and there could be a lots of use-case and examples of array.
¶How to create an array in PHP
array() is the keyword which is used to create array in PHP.
array()
There is another short form to create an array - third bracket []
.
Inside the parenthesis of the array, we can put as many value as need by comma(,)
separated. Like the below -
// Create an empty array of marks.
$marks = array();
// Update that array with some marks
$marks = array(80, 90, 100);
**Using [] as array create - **
// Create an empty array of marks.
$marks = [];
// Update that array with some marks
$marks = [80, 90, 100];
¶Types of Array in PHP
PHP has three types of array. There are -
- Indexed Array
- Associative Array
- Multi-dimensional Array
¶Indexed Array:
The array, which is used by there indexed.
¶Indexed Array Example:
// Create an empty array of marks.
$marks = array();
// Update that array with some marks
$marks = array(80, 90, 100);
¶Associative Array:
The array, which is used by key-value
pair. Here is the structure -
array(
key => value
)
¶Associative Array Example:
$student_data = array(
"name" => "Jhon",
"age" => 20,
"result" => 3.8
);
¶Multi-dimensional Array:
The array, which is used by multiple array inside one array with comma separation. Here is the structure -
array(
array(
key => value
),
array(
key => value
),
)
¶Multi-dimensional Array Example:
$students = array(
array(
"name" => "Jhon",
"age" => 20,
"result" => 3.8
),
array(
"name" => "Akash",
"age" => 26,
"result" => 2.5
),
)
¶How to access array property
We can access array property by their index or key. Let’s get the structure -
- For index - Array index starts from
0
. - For Key - just
key name
.
¶Access by Index
$marks = array(10, 30, 50);
Get the First mark or 0th
index mark -
echo $marks[0]; // 10
Get the Second mark or 1st
index mark -
echo $marks[1]; // 30
¶Access by Key name
Let’s access our top student data array values. Structure -
Array variable + Third bracket Open + quotation(single or double) + Key name + quotation(single or double) + Third bracket Close
$array['key_name']
Example:
$student_data = array(
"name" => "Jhon",
"age" => 20,
"result" => 3.8
);
// Get student name
echo $student_data['name']; // Jhon
// we can use
echo $student_data["age"]; // 20
¶Access by Loop
<?php
$students = array(
array(
"name" => "Jhon",
"age" => 20,
"result" => 3.8
),
array(
"name" => "Akash",
"age" => 26,
"result" => 2.5
),
);
// Access single item by a foreach loop
foreach($students as $student) {
echo "<h2>Name: " . $student['name'] . "</h2>";
echo "<p>Age: " . $student['age'] . "</p>";
echo "<hr>";
}
Output:
Name: Jhon
Age: 20
Name: Akash
Age: 26
Loops in PHP - for, while, foreach, do-while
PHP Array Part 2 - Array Built in PHP Functions and Some More Examples
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
13
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development