
PHP
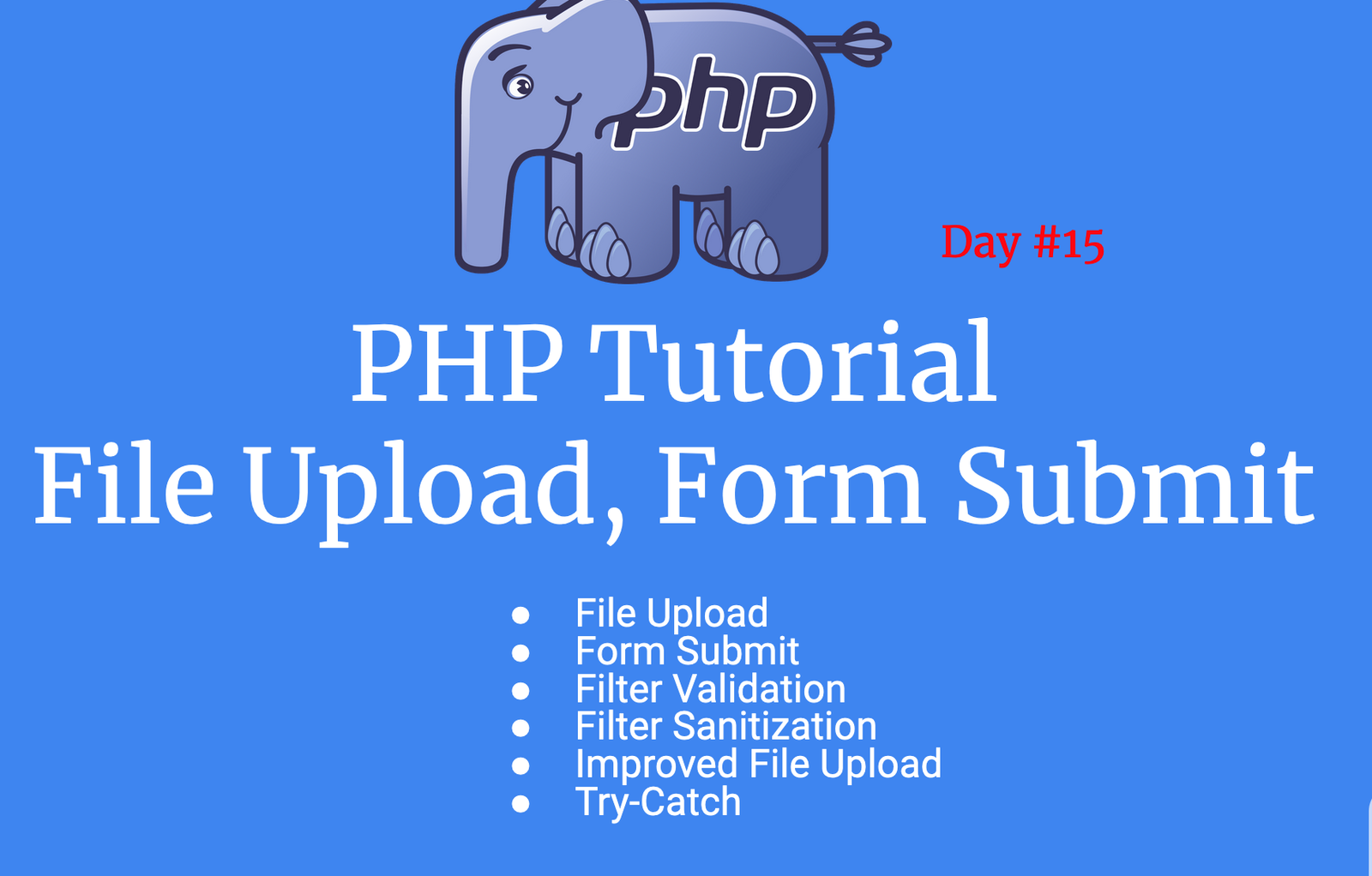
Form Submission and File Upload in PHP - Basic to Advance Level Form Submission in PHP
- Basic Form Submission
- Input Validation in PHP
- Form Submission with Global Errors variable Managed
- File Upload in PHP -
- Final Form Submission with Validation, Security, File Upload -
Form submission in PHP is a very important thing. In every phase, when we’ll work with database, we must use a form and make some save/checking with database. So form is a very important thing for Web Development. Today we’ll learn a complete form submission using PHP.
¶Basic Form Submission
Let’s make a basic form submission in PHP -
<h2>Contact Us</h2>
<?php
// We'll handle our form submission logic here. Let's just make Form Submitted. text if form is submitted.
if (isset($_POST['contact_form'])) {
echo "Form Submitted.";
}
?>
<form method="POST" action="<?php echo $_SERVER['PHP_SELF']; ?>">
<label for="name">Name</label><br>
<input type="text" id="name" name="name"> <br>
<br>
<label for="email">Email</label><br>
<input type="email" id="email" name="email"> <br>
<span style="color: red"><?php echo !empty($errors['email']) ? $errors['email'] : '' ?></span>
<br>
<label for="subject">Subject</label><br>
<input type="text" id="subject" name="subject"> <br>
<span style="color: red"><?php echo !empty($errors['subject']) ? $errors['subject'] : '' ?></span>
<br>
<input type="submit" value="Send" name="contact_form" />
</form>
Look in php first line -
if (isset($_POST['contact_form'])) {
echo "Form Submitted.";
}
isset($_POST['contact_form']) == true
if someone submit the form.
here, we’re first checking if someone click on submit button of contact_form
. For this, one must have to provide a name="contact_form"
value in input.
like so -
<input type="submit" value="Send" name="contact_form" />
And, we’ld make our method="post"
as we’ll handle everything in POST request. We can also do that in GET request. But POST request is more secured than GET request. Like so in form -
<form method="POST" action="<?php echo $_SERVER['PHP_SELF']; ?>">
¶Input Validation in PHP
Let’s now make a simple form validation in PHP by just checking if an input is empty or not -
<h2>Contact Us</h2>
<?php
if (isset($_POST['contact_form'])) {
if (empty($_POST['name'])) {
echo 'Please give a valid name.<br>';
}
if (empty($_POST['email'])) {
echo 'Please give an email address.<br>';
}
if (empty($_POST['subject'])) {
echo 'Please give a subject.<br>';
}
}
?>
<form method="POST" action="<?php echo $_SERVER['PHP_SELF']; ?>">
<label for="name">Name</label><br>
<input type="text" id="name" name="name"> <br>
<br>
<label for="email">Email</label><br>
<input type="email" id="email" name="email"> <br>
<br>
<label for="subject">Subject</label><br>
<input type="text" id="subject" name="subject"> <br>
<br>
<input type="submit" value="Send" name="contact_form" />
</form>
So, Output would be like this after empty form submission -
Output:

¶Form Submission with Global Errors variable Managed
Let’s now store our errors in $errors[]
and then process from this -
<h2>Contact Us</h2>
<?php
$errors = [];
if (isset($_POST['contact_form'])) {
if (empty($_POST['name'])) {
$errors['name'] = "Please give a valid name.";
}
if (empty($_POST['email'])) {
$errors['email'] = "Please give an email address.";
}
if (empty($_POST['subject'])) {
$errors['subject'] = "Please give a subject.";
}
}
?>
<?php if (count($errors)) : ?>
<div style='border: 1px solid red; padding: 10px; margin: 10px;'>
<?php foreach ($errors as $error) : ?>
<p style="color: red;"><?php echo $error; ?></p>
<?php endforeach; ?>
</div>
<?php endif; ?>
<form method="POST" action="<?php echo $_SERVER['PHP_SELF']; ?>">
<label for="name">Name</label><br>
<input type="text" id="name" name="name"> <br>
<br>
<label for="email">Email</label><br>
<input type="email" id="email" name="email"> <br>
<br>
<label for="subject">Subject</label><br>
<input type="text" id="subject" name="subject"> <br>
<br>
<input type="submit" value="Send" name="contact_form" />
</form>
So, it’s now little improved, right. After getting any error, we’re adding that with an index in $errors
variable.
if (empty($_POST['name'])) {
$errors['name'] = "Please give a valid name.";
}
Then show all of the errors one by one using a foreach loop
-
<?php if (count($errors)) : ?>
<div style='border: 1px solid red; padding: 10px; margin: 10px;'>
<?php foreach ($errors as $error) : ?>
<p style="color: red;"><?php echo $error; ?></p>
<?php endforeach; ?>
</div>
<?php endif; ?>
¶File Upload in PHP -
move_uploaded_file()
is used to upload any file in php - https://www.php.net/manual/en/function.move-uploaded-file.php
move_uploaded_file(string $from, string $to): bool
** So, this function takes **
- a file name as first parameter and
- File location to store in second parameter.
**Returns - ** Boolean(true) if file is uploaded successfully.
¶Simple File Upload Example -
Create a uploads
folder in root, so that uploaded image could be moved in this folder.
<?php
if (isset($_POST['contact_form'])) {
// Upload file if any file is provided
if (!empty($_FILES['attachment']['name'])) {
if (move_uploaded_file($_FILES['attachment']['tmp_name'], "uploads/" . $_FILES['attachment']['name'])) {
echo "File Uploaded.";
} else {
echo "File Upload Failed.";
}
}
}
?>
<form method="POST" action="<?php echo $_SERVER['PHP_SELF']; ?>" enctype="multipart/form-data">
<label for="attachment">Attachment (PDF or Image) (optional)</label><br>
<input type="file" id="attachment" name="attachment"> <br>
<span style="color: red"><?php echo !empty($errors['attachment']) ? $errors['attachment'] : '' ?></span>
<br>
<input type="submit" value="Send" name="contact_form" />
</form>
Great, after clicking send you’ll check a file has been uploaded. Forgettable Requirements in File Upload
- In form, there would be enctype property value set to
enctype="multipart/form-data"
. Without this, no image would be uploaded. -
move_uploaded_file($_FILES['attachment']['tmp_name'], "uploads/" . $_FILES['attachment']['name'])
- We must need to check if file is given like so -
if (!empty($_FILES['attachment']['name'])) {
¶Final Form Submission with Validation, Security, File Upload -
<h2>Contact Us</h2>
<?php
$errors = [];
try {
if (isset($_POST['contact_form'])) {
// Validation
if (empty($_POST['name'])) {
$errors['name'] = "Please give a valid name.";
// throw new Exception("Please give a valid name.", 1);
}
if (empty($_POST['email'])) {
$errors['email'] = "Please give an email address.";
}
if(!filter_var($_POST['email'], FILTER_VALIDATE_EMAIL)) {
$errors['email'] = "Please give a valid email.";
}
if (empty($_POST['subject'])) {
$errors['subject'] = "Please give a valid subject.";
}
if (empty($_POST['temp_password'])) {
$errors['temp_password'] = "Please give a valid temporary password.";
}
// Upload file
$file_name = null;
if (!empty($_FILES['attachment']['name'])) {
// Undefined | Multiple Files | $_FILES Corruption Attack
// If this request falls under any of them, treat it invalid.
if (
!isset($_FILES['attachment']['error']) ||
is_array($_FILES['attachment']['error'])
) {
throw new RuntimeException('Invalid parameters.');
}
// Check file size exceeded or not
$file_size = !empty($_FILES['attachment']['size']) ? intval($_FILES['attachment']['size']) : 0;
$upto_supported_size = 5 * 1024 * 1000; // 5MB
if ($file_size > $upto_supported_size) {
$errors['attachment'] = "File size exceeded. Max upload limit is 5MB";
throw new Exception($errors['attachment'], 1);
}
// Mime type of file
$finfo = new finfo(FILEINFO_MIME_TYPE);
$file_ext = array_search(
$finfo->file($_FILES['attachment']['tmp_name']),
array(
'jpg' => 'image/jpg',
'jpg' => 'image/jpeg',
'png' => 'image/png',
'gif' => 'image/gif',
'gif' => 'image/bnf',
'pdf' => 'application/pdf'
),
true
);
if (false === $file_ext) {
$errors['attachment'] = "File should be a valid image or PDF file";
throw new Exception($errors['attachment'], 1);
}
// Sanitize file name and make a new name
$file_name = 'devsenv-' . time() . '.' . $file_ext;
// Upload file
if (!move_uploaded_file($_FILES['attachment']['tmp_name'], "uploads/" . $file_name)) {
$errors['attachment'] = "File uploads fail, please try again.";
throw new Exception($errors['attachment']);
}
}
}
} catch (\Exception $e) {
$errors['global'] = $e->getMessage();
}
if (count($errors) > 0) {
$err_global = "Failed to submit form. Please try again.";
if (isset($errors['global'])) {
$err_global .= ' Error: ' .$errors['global'];
}
$errors['global'] = $err_global;
} else {
if (isset($_POST['contact_form'])) {
// Sanitization
$insert_data = [
'name' => htmlspecialchars($_POST['name']),
'email' => filter_var($_POST['email'], FILTER_VALIDATE_EMAIL),
'subject' => htmlspecialchars($_POST['subject']),
'temp_password' => htmlspecialchars($_POST['temp_password']),
'attachment' => $file_name
];
var_dump($insert_data);
// Insert into Database
// DB::insert('contact_us', $insert_data);
}
}
?>
<?php
if (!empty($errors['global'])) {
echo "<p style='color:red; border: 1px solid #ccc; padding: 10px;'>" . $errors['global'] . "</p>";
} elseif (isset($_POST['contact_form'])) {
echo "<p style='color:green'>Your form is submitted !</p>";
}
?>
<form method="POST" action="<?php echo $_SERVER['PHP_SELF']; ?>" enctype="multipart/form-data">
<label for="name">Name</label><br>
<input type="text" id="name" name="name"> <br>
<span style="color: red"><?php echo !empty($errors['name']) ? $errors['name'] : '' ?></span>
<br>
<label for="email">Email</label><br>
<input type="email" id="email" name="email"> <br>
<span style="color: red"><?php echo !empty($errors['email']) ? $errors['email'] : '' ?></span>
<br>
<label for="subject">Subject</label><br>
<input type="text" id="subject" name="subject"> <br>
<span style="color: red"><?php echo !empty($errors['subject']) ? $errors['subject'] : '' ?></span>
<br>
<label for="temp_password">Temp Password</label><br>
<input type="password" id="temp_password" name="temp_password"> <br>
<span style="color: red"><?php echo !empty($errors['temp_password']) ? $errors['temp_password'] : '' ?></span>
<br>
<label for="attachment">Attachment (PDF or Image) (optional)</label><br>
<input type="file" id="attachment" name="attachment"> <br>
<span style="color: red"><?php echo !empty($errors['attachment']) ? $errors['attachment'] : '' ?></span>
<br>
<input type="submit" value="Send" name="contact_form" />
</form>

PHP Superglobal Variables and It's Practical Examples - Get, Post, Session, Cookie, Request, Server
PHP OOP - Object Oriented Programming Introduction
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development