
PHP
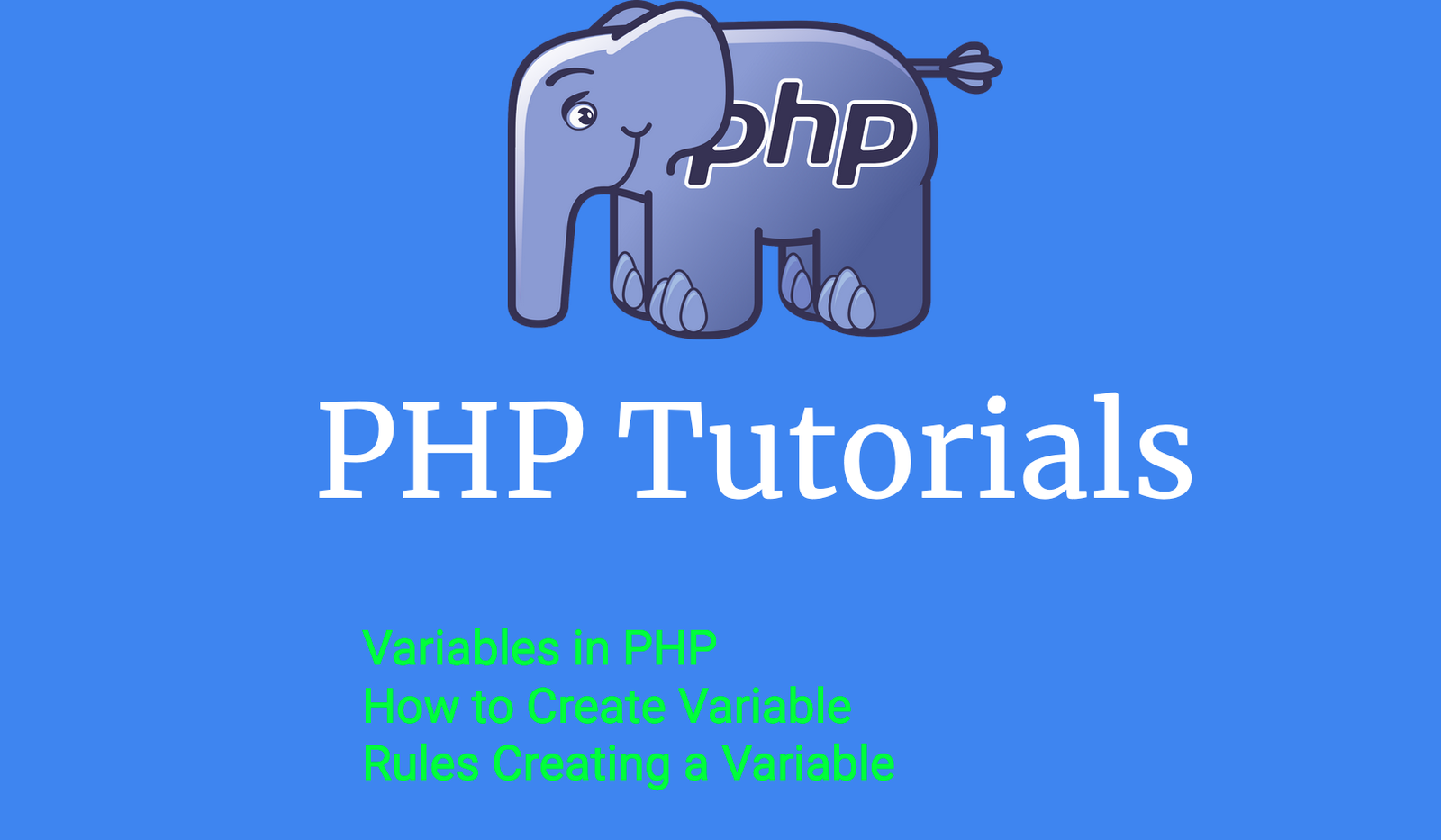
Variables in PHP - How to Create, Rules and Examples
- What is Variable in PHP
- How to declare a Variable in PHP
- Rules to Create a Variable name in PHP
- Invalid Variable Name Example in PHP
- Example of Some Valid Variable Name in PHP
¶What is Variable in PHP
Variable is like a Jar or Container, which stores information.
Not only in PHP, for any programming language, to process any data, we need to store something in somewhere and then, we process that data in later.
You can think it like, to calculate the summation of two numbers, we can store the summation to a jar and name it result
. Then anywhere of our application, we can use that result
. And in programming language, this result
is actually the Variable
.
¶How to declare a Variable in PHP
Variable Name Formula: Dollar sign ($) + name of the variable.
Example: $name
$age
$userName
$name1
$name2
$_is_available
$avatar_url
¶Assign a value to variable:
After getting the idea of a variable name, let’s assign a value or store a value to variable.
$name = "Jhon Doe";
$age = 28;
$avatar_url = "jhondoe@example.com";
$product_price = 1,200.50
$_is_available = true;
Summation value store example with variable:
$number1 = 20;
$number2 = 30;
$summation = $number1 + $number2;
¶Rules to Create a Variable name in PHP
- Variable name is case sensitive -
$age
and$AGE
is not same variable. - Variable starts with letter(a, b, … z) or underscore(_) eg:
$name
,$_age
- After first letter or underscore, then any letter, number or underscore
- eg: $user, $user1, $age, $user1_age, $user2_age
- Variable name should be meaningful (Not rule, but a very good convention) Like:
$user_n
is not good for a variable name for user name, we should rather name that like$user_name
or$userName
.
¶Invalid Variable Name Example in PHP
-
$123
is not a valid variable - Variable should start with a-z or A-Z or with underscore. But here starts with Number. -
$ age
is not a valid variable - Cause, after the$
sign, there is a space( -
$name 1
is not a valid variable but$name1
is - Inside variable name, there should not be any space.
¶Example of Some Valid Variable Name in PHP
-
$name
-
$age
-
$userName
-
$name1
-
$name2
-
$_is_available
-
$avatar_url
-
$NAME
-
$USER_ADDRESS
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development