
PHP
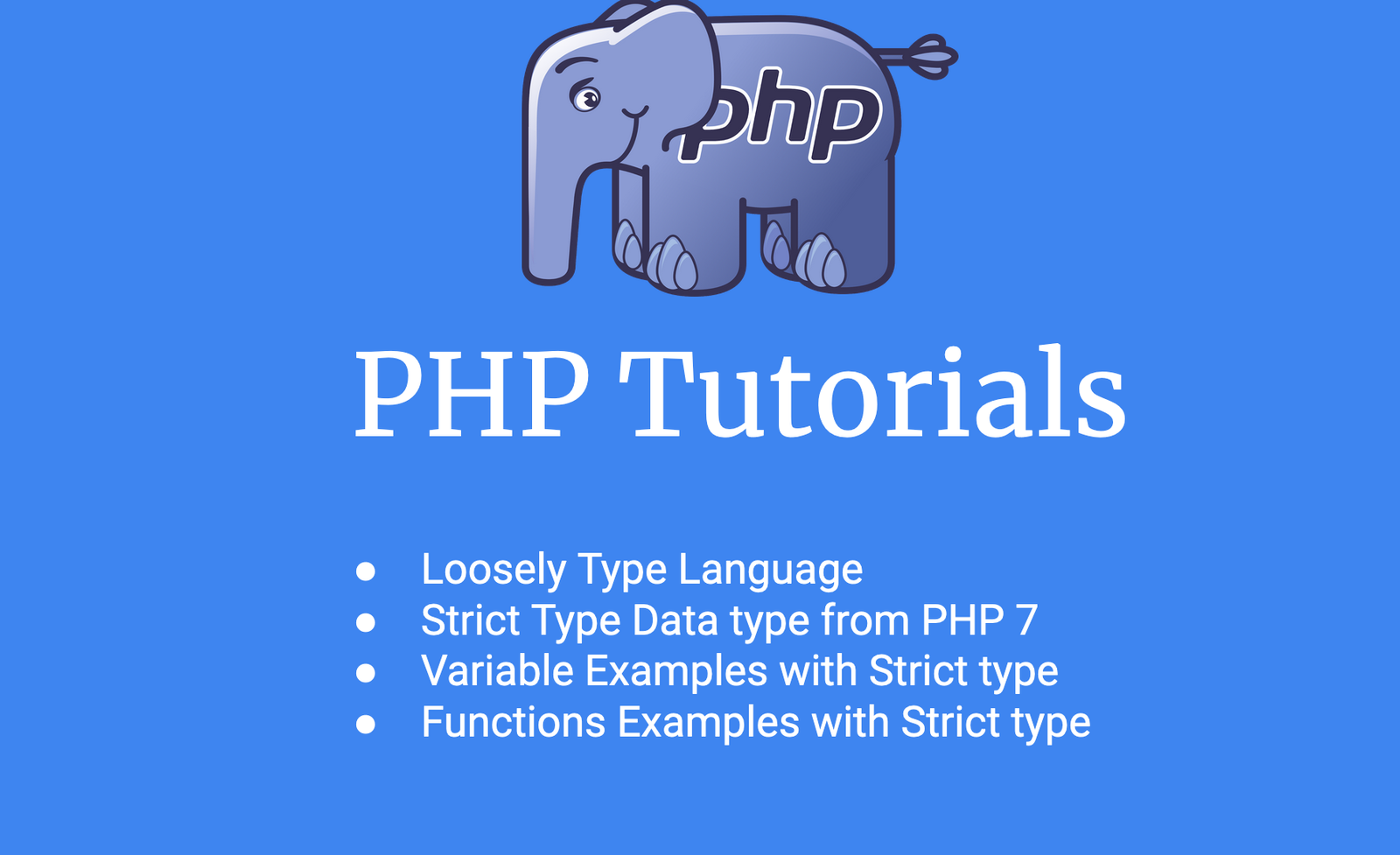
PHP Loosely Type Language - Strict type from PHP 7
¶Loosely Typed Language - PHP
PHP is a Loosely typed language, that means we don’t need to define the Data type of variable in PHP while we declare it. PHP automatically assume a data type for that variable, based on the value of that variable. Suppose, Let’s assign some variable and test it’s data type -
$name = "Jhon";
var_dump($name); // string(4) "Jhon"
$age = 20;
var_dump($age); // int(20)
$price = 800.50;
var_dump($price); // float(800.5)
In the above examples, we don’t define the data type of any variable. PHP automatically associates that data type for those variables. And we can then update the variables with another data type. Like so -
$name = "Jhon";
var_dump($name); // string(4) "Jhon"
$name = 50.5;
var_dump($name); // float(50.5)
At first, we assign Jhon String type data to variable $name
.
And then again, assign 50.5 or float type data to it. In other programming languages, if we want to assign another data type value to that variable, then it would create a fatal error or exception. But, php doesn’t create any type of exception.
That’s why PHP is called Loosely type language.
¶Strict type example -
From PHP Version 7, PHP added support for strict type data type. Like, if we assign a string value to any variable, we can not assign then integer or float values to that variable. PHP will create a warning or exception like other language. We would call that Strict type.
Let’s get an example of that -
Before strict implementation is ON, we have to add declare(strict_types=1);
at very first line of the PHP code.
Let’s implement the previous chapter getAge()
method with strict type in PHP.
<?php
declare(strict_types=1);
function getAge(int $age): void
{
if ($age >= 0 && $age < 18) {
echo "You are a Child";
} elseif ($age >= 18 && $age < 50) {
echo "You are an Adult";
} elseif ($age >= 50) {
echo "You are Old";
} else {
echo "Age is not valid.";
}
}
echo getAge(40.5); // Fatal Error
Output:
As if getAge()
is expecting integer int $age
, and when calling if we pass float
value, it will be given a fatal error that we can not assign float
value to int
type argument.
¶Strict type in Variable in PHP
We’ll discuss this in our OOP chapter, as we can use strict data type for a variable in PHP in our PHP class. Let’s check a demo,
<?php
declare(strict_types=1);
class Student
{
public string $name;
public int $age;
public float $gpa;
public function getGpA(): float
{
return $this->gpa;
}
public function setGpA(float $gpa): void
{
$this->gpa = $gpa;
}
}
Don’t worry, we’ll discuss this type of Strict use in our PHP OOP chapter.
PHP - Functions in PHP - How to create and how to use
PHP Superglobal Variables and It's Practical Examples - Get, Post, Session, Cookie, Request, Server
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development