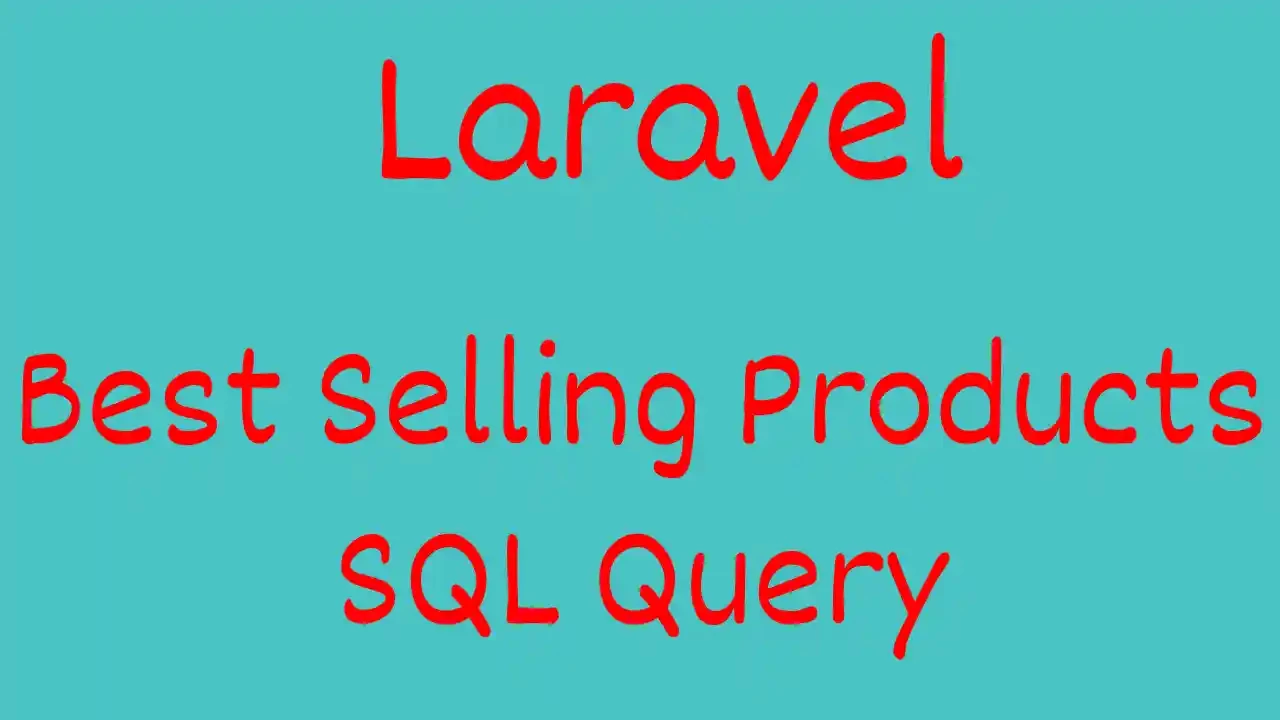
Get Best Selling Products Efficient Query in Laravel
Get Best selling products for your Ecommerce Store in Laravel -
So, assume we've a transaction_sell_lines
table which is connected to TransactionSellLine
eloquent model.
Item table - items
Transaction Sell Items table - transaction_sell_lines
Laravel Code snippet -
public function getBestSellingProducts($limit = 10)
{
$bestSellingProducts = TransactionSellLine::select(
'item_id',
'items.name as item_name',
'items.sku as item_sku',
DB::raw('SUM((quantity * unit_price_inc_tax) - discount_amount - item_tax) as total_sale')
)
->leftJoin('items', 'items.id', '=', 'transaction_sell_lines.item_id')
->groupBy('item_id')
->orderBy('total_sale', 'desc')
->limit($limit)
->get();
return $bestSellingProducts;
}
We've actually used the DB::raw
query inside select.
DB::raw('SUM((quantity * unit_price_inc_tax) - discount_amount - item_tax) as total_sale')
Then, use descending order -
->orderBy('total_sale', 'desc')
Very simple to implement best selling products in laravel, right ?
PHP If-else-elseif and Switch-case
PHP String Functions - All necessary String functions in PHP to manage strings better.
Popular Tutorials
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development