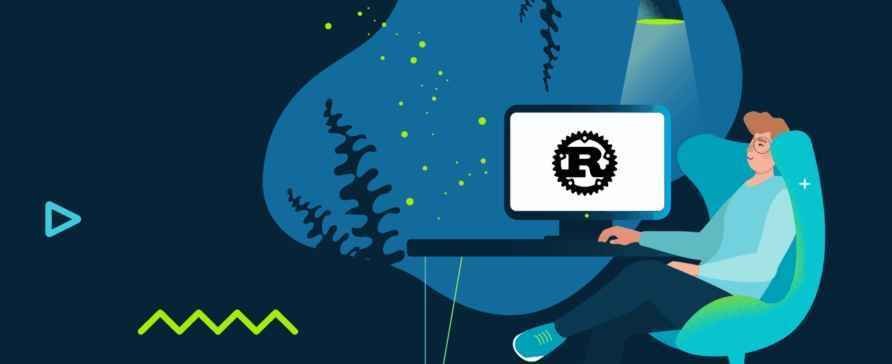
Guide to become a RUST developer - Learn RUST programming language with Examples
- What is RUST Programming Language
- How to Setup RUST environment locally
- Common Syntax of RUST Programming language
-
Syntax of Rust at a Glance
- 1. Variables and Data Types
- 2. Functions
- 3. Ownership and Borrowing
- 4. Structs
- 5. Enums
- 6. Pattern Matching
- 7. Vectors
- 8. Error Handling
- 9. Concurrency
- 10. Comments
-
Example of Some RUST Programming Example
- 1. Rust Hello World Program
- 2. Rust Program to Print Your Own Name
- 3. Rust Program to Print an Integer Entered By the User
- 4. Rust Program to Add Two Numbers
- 5. Rust Program to Check Whether a Number is Prime or Not
- 6. Rust Program to Multiply Two Floating-Point Numbers
- 7. Rust Program to Print the ASCII Value of a Character
- 8. Rust Program to Swap Two Numbers
- 9. Rust Program to Calculate Fahrenheit to Celsius
- 10. Rust Program to Find the Size of int, float, double, and char
- 11. Rust Program to Add Two Complex Numbers
- 12. Rust Program to Print Prime Numbers From 1 to N
- 13. Rust Program to Find Simple Interest
- 14. Rust Program to Find Compound Interest
- 15. Rust Program for Area And Perimeter Of Rectangle
- 16. Rust Program to Check Whether a Number is Positive, Negative, or Zero
- 17. Rust Program to Check Whether Number is Even or Odd
- 18. Rust Program to Check Whether a Character is Vowel or Consonant
- 19. Rust Program to Find Largest Number Among Three Numbers
- 20. Rust Program to Calculate Sum of Natural Numbers
- 21. Rust Program to Print Alphabets From A to Z Using Loop
- 22. Rust Program to Check Leap Year
- 23. Rust Program to Find Factorial of a Number
- 24. Rust Program to Make a Simple Calculator
- 25. Rust Program to Generate Multiplication Table
- 26. Rust Program to Print Fibonacci Series
- 27. Rust Program to Find LCM of Two Numbers
- 28. Rust Program to Check Armstrong Number
- 29. Rust Program to Display Armstrong Numbers Between 1 to 1000
- 30. Rust Program to Display Armstrong Number Between Two Intervals
- 31. Rust Program to Reverse a Number
- 32. Rust Program to Check Whether a Number is a Palindrome or Not
- 34. Rust Program to Display Prime Numbers Between Intervals
- 35. Rust Program to Check whether the input number is a Neon Number
- 36. Rust Program to Find All Factors of a Natural Number
- 37. Rust Program to Sum of Fibonacci Numbers at Even Indexes up to N Terms
- 38. File I/O Read and write to files.
¶What is RUST Programming Language
RUST is a modern, systems-level programming language known for its focus on safety, performance, and concurrency. Developed by Mozilla, Rust was designed to address common programming pitfalls, particularly those related to memory management and thread safety, while still delivering high-performance code. Here’s a short introduction to some of the key features and characteristics that define Rust:
-
Memory Safety: Rust’s most prominent feature is its ownership system, which enforces strict rules about how memory is allocated, accessed, and deallocated. This prevents common issues like null pointer dereferences and buffer overflows, making Rust code safe from memory-related vulnerabilities.
-
Concurrency: Rust has built-in support for concurrent programming with features like threads and asynchronous programming. The ownership system ensures that data races and other concurrency bugs are caught at compile time, reducing the risk of hard-to-debug issues.
-
Zero-Cost Abstractions: Rust allows developers to write high-level code with abstractions like structs, enums, and traits while ensuring that these abstractions have no runtime overhead. This makes Rust code efficient and performant.
-
C and C++ Interoperability: Rust can easily interface with C and C++ code, making it suitable for systems programming and integration with existing libraries and systems.
-
Borrowing and Lifetimes: Rust introduces concepts like borrowing and lifetimes to manage references to data. These mechanisms prevent data races and enable the safe sharing of data between different parts of a program.
-
Cargo: Rust’s package manager, Cargo, simplifies dependency management, project setup, and building. It also provides extensive testing and documentation tools, enhancing the development experience.
-
Community and Ecosystem: Rust has a growing and active community of developers. Its ecosystem includes a rich collection of libraries and frameworks, making it suitable for a wide range of applications, from systems programming to web development and more.
-
Cross-Platform: Rust is designed to be cross-platform, enabling developers to write code that can run on various operating systems without modification.
-
Open Source: Rust is an open-source language with a permissive license, encouraging contributions and fostering a collaborative development environment.
In summary, Rust offers a compelling combination of memory safety, concurrency, performance, and modern tooling, making it an excellent choice for developers seeking to build reliable and efficient software, particularly in areas where system-level control and safety are essential.
¶How to Setup RUST environment locally
Setting up a Rust development environment locally on Windows, macOS, or Linux involves a few common steps. Here’s a brief guide on how to do it for each of these operating systems:
¶Windows
-
Install Rust:
- Visit the official Rust website at https://www.rust-lang.org/ and click on the “Install” button.
- Download the Rust installer for Windows.
- Run the installer and follow the on-screen instructions.
- During installation, you’ll be prompted to customize the installation. It’s recommended to enable the “Add to PATH environment variable” option for easier command-line access to Rust tools.
-
Verify Installation:
Open a Command Prompt or PowerShell window and type:
rustc --version
This should display the installed Rust version, confirming that Rust is properly installed.
¶macOS
-
Install Rust:
-
You can use the Rust installation script. Open a Terminal and run:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
-
Follow the on-screen instructions to complete the installation. By default, Rustup will add Rust to your PATH.
-
-
Verify Installation:
In the Terminal, type:
rustc --version
You should see the installed Rust version displayed, confirming a successful installation.
¶Linux
-
Install Rust:
-
Open a Terminal and run the following command to download and run the Rust installation script:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
-
Follow the on-screen instructions. Rustup will add Rust to your PATH.
-
-
Verify Installation:
In the Terminal, type:
rustc --version
This should display the installed Rust version, confirming that Rust is correctly installed.
¶Common Steps for All Operating Systems
-
Install a Text Editor or IDE:
Choose a code editor or integrated development environment (IDE) for writing Rust code. Popular options include Visual Studio Code with the Rust extension, JetBrains’ IntelliJ IDEA with the Rust plugin, or Sublime Text with Rust packages.
-
Create Your First Rust Project:
Open a terminal or command prompt and navigate to the directory where you want to create your Rust project. Use the following command to create a new Rust project:
cargo new my_rust_project
This command will generate a basic Rust project structure.
-
Build and Run Your Project:
Inside your project directory, run the following command to build and run your Rust program:
cd my_rust_project cargo run
This will compile and execute your Rust code.
With these steps, you’ll have a local Rust development environment set up on your Windows, macOS, or Linux machine, and you’ll be ready to start writing and running Rust programs.
¶Common Syntax of RUST Programming language
¶Syntax of Rust at a Glance
Rust is a modern and powerful systems programming language known for its safety, performance, and expressive syntax. In this brief article, we’ll take a quick look at the key elements of Rust’s syntax.
¶1. Variables and Data Types
In Rust, you declare variables using the let
keyword, and the type can often be inferred by the compiler:
let x = 5; // Integer
let name = "Alice"; // String (str)
let is_rust_cool = true; // Boolean
¶2. Functions
Function definitions start with the fn
keyword. Rust uses snake_case for function and variable names:
fn greet(name: &str) {
println!("Hello, {}!", name);
}
¶3. Ownership and Borrowing
Rust’s ownership system ensures memory safety. Variables “own” their data, and you can transfer ownership using move
or borrow with &
:
fn main() {
let s1 = String::from("Hello");
let s2 = s1; // Moves ownership to s2
// println!("{}", s1); // This would result in a compilation error
println!("{}", s2); // s2 is valid here
}
¶4. Structs
You can define custom data structures using structs:
struct Person {
name: String,
age: u32,
}
let alice = Person {
name: String::from("Alice"),
age: 30,
};
¶5. Enums
Enums define a type with a fixed set of values:
enum Color {
Red,
Green,
Blue,
}
let favorite = Color::Blue;
¶6. Pattern Matching
Rust’s match
expression allows for powerful pattern matching:
match favorite {
Color::Red => println!("It's red!"),
Color::Green | Color::Blue => println!("It's green or blue!"),
}
¶7. Vectors
Vectors are dynamic arrays:
let numbers = vec![1, 2, 3];
for num in &numbers {
println!("Number: {}", num);
}
¶8. Error Handling
Rust uses the Result
type for error handling:
fn divide(x: f64, y: f64) -> Result<f64, &'static str> {
if y == 0.0 {
Err("Division by zero")
} else {
Ok(x / y)
}
}
match divide(10.0, 2.0) {
Ok(result) => println!("Result: {}", result),
Err(err) => println!("Error: {}", err),
}
¶9. Concurrency
Rust supports concurrent programming with threads:
use std::thread;
let handle = thread::spawn(|| {
for i in 1..=5 {
println!("Thread: {}", i);
}
});
for i in 1..=3 {
println!("Main: {}", i);
}
handle.join().unwrap();
¶10. Comments
Rust supports both single-line and multi-line comments:
// This is a single-line comment
/*
This is a
multi-line comment
*/
¶Example of Some RUST Programming Example
Here are some beginner-level programming examples using Rust. Which could be a very good start for a beginner level RUST programmer.
¶1. Rust Hello World Program
fn main() {
println!("Hello, World!");
}
¶2. Rust Program to Print Your Own Name
fn main() {
println!("My name is YourName");
}
¶3. Rust Program to Print an Integer Entered By the User
use std::io;
fn main() {
let mut input = String::new();
println!("Enter an integer: ");
io::stdin().read_line(&mut input).expect("Failed to read line");
let num: i32 = input.trim().parse().expect("Invalid input");
println!("You entered: {}", num);
}
¶4. Rust Program to Add Two Numbers
use std::io;
fn main() {
let mut input1 = String::new();
let mut input2 = String::new();
println!("Enter the first number: ");
io::stdin().read_line(&mut input1).expect("Failed to read line");
println!("Enter the second number: ");
io::stdin().read_line(&mut input2).expect("Failed to read line");
let num1: f64 = input1.trim().parse().expect("Invalid input");
let num2: f64 = input2.trim().parse().expect("Invalid input");
let sum = num1 + num2;
println!("Sum: {}", sum);
}
¶5. Rust Program to Check Whether a Number is Prime or Not
use std::io;
fn is_prime(num: u32) -> bool {
if num <= 1 {
return false;
}
for i in 2..=(num / 2) {
if num % i == 0 {
return false;
}
}
true
}
fn main() {
let mut input = String::new();
println!("Enter a number: ");
io::stdin().read_line(&mut input).expect("Failed to read line");
let num: u32 = input.trim().parse().expect("Invalid input");
if is_prime(num) {
println!("{} is prime.", num);
} else {
println!("{} is not prime.", num);
}
}
¶6. Rust Program to Multiply Two Floating-Point Numbers
use std::io;
fn main() {
let mut input1 = String::new();
let mut input2 = String::new();
println!("Enter the first number: ");
io::stdin().read_line(&mut input1).expect("Failed to read line");
println!("Enter the second number: ");
io::stdin().read_line(&mut input2).expect("Failed to read line");
let num1: f64 = input1.trim().parse().expect("Invalid input");
let num2: f64 = input2.trim().parse().expect("Invalid input");
let product = num1 * num2;
println!("Product: {}", product);
}
¶7. Rust Program to Print the ASCII Value of a Character
use std::io;
fn main() {
let mut input = String::new();
println!("Enter a character: ");
io::stdin().read_line(&mut input).expect("Failed to read line");
let character = input.chars().next().expect("No character entered");
let ascii_value = character as u32;
println!("ASCII value of {} is: {}", character, ascii_value);
}
¶8. Rust Program to Swap Two Numbers
fn main() {
let mut num1 = 5;
let mut num2 = 10;
println!("Before swapping: num1 = {}, num2 = {}", num1, num2);
let temp = num1;
num1 = num2;
num2 = temp;
println!("After swapping: num1 = {}, num2 = {}", num1, num2);
}
Certainly! Here are more Rust programs for the tasks you’ve mentioned:
¶9. Rust Program to Calculate Fahrenheit to Celsius
use std::io;
fn main() {
let mut input = String::new();
println!("Enter temperature in Fahrenheit: ");
io::stdin().read_line(&mut input).expect("Failed to read line");
let fahrenheit: f64 = input.trim().parse().expect("Invalid input");
let celsius = (fahrenheit - 32.0) * 5.0 / 9.0;
println!("Temperature in Celsius: {:.2}", celsius);
}
¶10. Rust Program to Find the Size of int, float, double, and char
fn main() {
println!("Size of i32: {} bytes", std::mem::size_of::<i32>());
println!("Size of f64: {} bytes", std::mem::size_of::<f64>());
println!("Size of f64: {} bytes", std::mem::size_of::<f64>());
println!("Size of char: {} bytes", std::mem::size_of::<char>());
}
¶11. Rust Program to Add Two Complex Numbers
struct Complex {
real: f64,
imaginary: f64,
}
fn add_complex(c1: Complex, c2: Complex) -> Complex {
Complex {
real: c1.real + c2.real,
imaginary: c1.imaginary + c2.imaginary,
}
}
fn main() {
let c1 = Complex { real: 3.0, imaginary: 2.0 };
let c2 = Complex { real: 1.5, imaginary: 4.5 };
let result = add_complex(c1, c2);
println!("Sum: {} + {}i", result.real, result.imaginary);
}
¶12. Rust Program to Print Prime Numbers From 1 to N
use std::io;
fn is_prime(num: u32) -> bool {
if num <= 1 {
return false;
}
for i in 2..=(num / 2) {
if num % i == 0 {
return false;
}
}
true
}
fn main() {
let mut input = String::new();
println!("Enter a number (N): ");
io::stdin().read_line(&mut input).expect("Failed to read line");
let n: u32 = input.trim().parse().expect("Invalid input");
println!("Prime numbers from 1 to {}:", n);
for i in 1..=n {
if is_prime(i) {
print!("{} ", i);
}
}
println!();
}
¶13. Rust Program to Find Simple Interest
use std::io;
fn main() {
let mut principal = String::new();
let mut rate = String::new();
let mut time = String::new();
println!("Enter principal amount: ");
io::stdin().read_line(&mut principal).expect("Failed to read line");
println!("Enter rate of interest: ");
io::stdin().read_line(&mut rate).expect("Failed to read line");
println!("Enter time (in years): ");
io::stdin().read_line(&mut time).expect("Failed to read line");
let principal: f64 = principal.trim().parse().expect("Invalid input");
let rate: f64 = rate.trim().parse().expect("Invalid input");
let time: f64 = time.trim().parse().expect("Invalid input");
let simple_interest = (principal * rate * time) / 100.0;
println!("Simple Interest: {}", simple_interest);
}
¶14. Rust Program to Find Compound Interest
use std::io;
fn main() {
let mut principal = String::new();
let mut rate = String::new();
let mut time = String::new();
println!("Enter principal amount: ");
io::stdin().read_line(&mut principal).expect("Failed to read line");
println!("Enter rate of interest: ");
io::stdin().read_line(&mut rate).expect("Failed to read line");
println!("Enter time (in years): ");
io::stdin().read_line(&mut time).expect("Failed to read line");
let principal: f64 = principal.trim().parse().expect("Invalid input");
let rate: f64 = rate.trim().parse().expect("Invalid input");
let time: f64 = time.trim().parse().expect("Invalid input");
let compound_interest = principal * (1.0 + rate / 100.0).powf(time) - principal;
println!("Compound Interest: {}", compound_interest);
}
¶15. Rust Program for Area And Perimeter Of Rectangle
use std::io;
fn main() {
let mut length = String::new();
let mut width = String::new();
println!("Enter length of the rectangle: ");
io::stdin().read_line(&mut length).expect("Failed to read line");
println!("Enter width of the rectangle: ");
io::stdin().read_line(&mut width).expect("Failed to read line");
let length: f64 = length.trim().parse().expect("Invalid input");
let width: f64 = width.trim().parse().expect("Invalid input");
let area = length * width;
let perimeter = 2.0 * (length + width);
println!("Area: {}", area);
println!("Perimeter: {}", perimeter);
}
¶16. Rust Program to Check Whether a Number is Positive, Negative, or Zero
use std::io;
fn main() {
let mut num = String::new();
println!("Enter a number: ");
io::stdin().read_line(&mut num).expect("Failed to read line");
let num: f64 = num.trim().parse().expect("Invalid input");
if num > 0.0 {
println!("The number is positive.");
} else if num < 0.0 {
println!("The number is negative.");
} else {
println!("The number is zero.");
}
}
¶17. Rust Program to Check Whether Number is Even or Odd
use std::io;
fn main() {
let mut num = String::new();
println!("Enter a number: ");
io::stdin().read_line(&mut num).expect("Failed to read line");
let num: i32 = num.trim().parse().expect("Invalid input");
if num % 2 == 0 {
println!("The number is even.");
} else {
println!("The number is odd.");
}
}
¶18. Rust Program to Check Whether a Character is Vowel or Consonant
use std::io;
fn main() {
let mut character = String::new();
println!("Enter a character: ");
io::stdin().read_line(&mut character).expect("Failed to read line");
let character = character.trim().to_lowercase().chars().next().expect("No character entered");
match character {
'a' | 'e' | 'i' | 'o' | 'u' => println!("The character is a vowel."),
_ => println!("The character is a consonant."),
}
}
¶19. Rust Program to Find Largest Number Among Three Numbers
use std::io;
fn main() {
let mut num1 = String::new();
let mut num2 = String::new();
let mut num3 = String::new();
println!("Enter the first number: ");
io::stdin().read_line(&mut num1).expect("Failed to read line");
println!("Enter the second number: ");
io::stdin().read_line(&mut num2).expect("Failed to read line");
println!("Enter the third number: ");
io::stdin().read_line(&mut num3).expect("Failed to read line");
let num1: f64 = num1.trim().parse().expect("Invalid input");
let num2: f64 = num2.trim().parse().expect("Invalid input");
let num3: f64 = num3.trim().parse().expect("Invalid input");
let max = num1.max(num2).max(num3);
println!("The largest number is: {}", max);
}
¶20. Rust Program to Calculate Sum of Natural Numbers
use std::io;
fn main() {
let mut n = String::new();
println!("Enter a positive integer (n): ");
io::stdin().read_line(&mut n).expect("Failed to read line");
let n: u32 = n.trim().parse().expect("Invalid input");
let sum = (n * (n + 1)) / 2;
println!("Sum of natural numbers from 1 to {} is: {}", n, sum);
}
¶21. Rust Program to Print Alphabets From A to Z Using Loop
fn main() {
for ch in 'a'..'z' {
print!("{} ", ch);
}
println!();
}
¶22. Rust Program to Check Leap Year
use std::io;
fn is_leap_year(year: i32) -> bool {
(year % 4 == 0 && year % 100 != 0) || (year % 400 == 0)
}
fn main() {
let mut year = String::new();
println!("Enter a year: ");
io::stdin().read_line(&mut year).expect("Failed to read line");
let year: i32 = year.trim().parse().expect("Invalid input");
if is_leap_year(year) {
println!("{} is a leap year.", year);
} else {
println!("{} is not a leap year.", year);
}
}
¶23. Rust Program to Find Factorial of a Number
use std::io;
fn factorial(n: u64) -> u64 {
if n <= 1 {
return 1;
}
n * factorial(n - 1)
}
fn main() {
let mut num = String::new();
println!("Enter a non-negative integer: ");
io::stdin().read_line(&mut num).expect("Failed to read line");
let num: u64 = num.trim().parse().expect("Invalid input");
let result = factorial(num);
println!("Factorial of {} is: {}", num, result);
}
¶24. Rust Program to Make a Simple Calculator
use std::io;
fn main() {
let mut num1 = String::new();
let mut num2 = String::new();
let mut operator = String::new();
println!("Enter the first number: ");
io::stdin().read_line(&mut num1).expect("Failed to read line");
println!("Enter an operator (+, -, *, /): ");
io::stdin().read_line(&mut operator).expect("Failed to read line");
println!("Enter the second number: ");
io::stdin().read_line(&mut num2).expect("Failed to read line");
let num1: f64 = num1.trim().parse().expect("Invalid input");
let num2: f64 = num2.trim().parse().expect("Invalid input");
let result = match operator.trim() {
"+" => num1 + num2,
"-" => num1 - num2,
"*" => num1 * num2,
"/" => {
if num2 == 0.0 {
println!("Division by zero is not allowed.");
return;
}
num1 / num2
}
_ => {
println!("Invalid operator.");
return;
}
};
println!("Result: {}", result);
}
¶25. Rust Program to Generate Multiplication Table
use std::io;
fn main() {
let mut num = String::new();
println!("Enter a number to generate its multiplication table: ");
io::stdin().read_line(&mut num).expect("Failed to read line");
let num: i32 = num.trim().parse().expect("Invalid input");
for i in 1..=10 {
let result = num * i;
println!("{} x {} = {}", num, i, result);
}
}
¶26. Rust Program to Print Fibonacci Series
use std::io;
fn main() {
let mut n = String::new();
println!("Enter the number of terms for the Fibonacci series: ");
io::stdin().read_line(&mut n).expect("Failed to read line");
let n: u32 = n.trim().parse().expect("Invalid input");
let mut first = 0;
let mut second = 1;
for _ in 0..n {
print!("{} ", first);
let next = first + second;
first = second;
second = next;
}
println!();
}
¶27. Rust Program to Find LCM of Two Numbers
use std::io;
fn gcd(mut a: u32, mut b: u32) -> u32 {
while b != 0 {
let temp = b;
b = a % b;
a = temp;
}
a
}
fn lcm(a: u32, b: u32) -> u32 {
(a * b) / gcd(a, b)
}
fn main() {
let mut num1 = String::new();
let mut num2 = String::new();
println!("Enter the first number: ");
io::stdin().read_line(&mut num1).expect("Failed to read line");
println!("Enter the second number: ");
io::stdin().read_line(&mut num2).expect("Failed to read line");
let num1: u32 = num1.trim().parse().expect("Invalid input");
let num2: u32 = num2.trim().parse().expect("Invalid input");
let result = lcm(num1, num2);
println!("LCM of {} and {} is: {}", num1, num2, result);
}
¶28. Rust Program to Check Armstrong Number
use std::io;
fn is_armstrong(num: u32) -> bool {
let mut n = num;
let mut sum = 0;
let order = (num as f64).log10() as u32 + 1;
while n > 0 {
let digit = n % 10;
sum += digit.pow(order);
n /= 10;
}
sum == num
}
fn main() {
let mut num = String::new();
println!("Enter a number: ");
io::stdin().read_line(&mut num).expect("Failed to read line");
let num: u32 = num.trim().parse().expect("Invalid input");
if is_armstrong(num) {
println!("{} is an Armstrong number.", num);
} else {
println!("{} is not an Armstrong number.", num);
}
}
¶29. Rust Program to Display Armstrong Numbers Between 1 to 1000
fn is_armstrong(num: u32) -> bool {
let mut n = num;
let mut sum = 0;
let order = (num as f64).log10() as u32 + 1;
while n > 0 {
let digit = n % 10;
sum += digit.pow(order);
n /= 10;
}
sum == num
}
fn main() {
println!("Armstrong numbers between 1 and 1000:");
for num in 1..=1000 {
if is_armstrong(num) {
print!("{} ", num);
}
}
println!();
}
¶30. Rust Program to Display Armstrong Number Between Two Intervals
fn is_armstrong(num: u32) -> bool {
let mut n = num;
let mut sum = 0;
let order = (num as f64).log10() as u32 + 1;
while n > 0 {
let digit = n % 10;
sum += digit.pow(order);
n /= 10;
}
sum == num
}
fn main() {
let mut lower = String::new();
let mut upper = String::new();
println!("Enter the lower interval: ");
io::stdin().read_line(&mut lower).expect("Failed to read line");
println!("Enter the upper interval: ");
io::stdin().read_line(&mut upper
).expect("Failed to read line");
let lower: u32 = lower.trim().parse().expect("Invalid input");
let upper: u32 = upper.trim().parse().expect("Invalid input");
println!("Armstrong numbers between {} and {}:", lower, upper);
for num in lower..=upper {
if is_armstrong(num) {
print!("{} ", num);
}
}
println!();
}
¶31. Rust Program to Reverse a Number
use std::io;
fn main() {
let mut num = String::new();
println!("Enter a number: ");
io::stdin().read_line(&mut num).expect("Failed to read line");
let mut num: i32 = num.trim().parse().expect("Invalid input");
let mut reversed = 0;
while num != 0 {
let digit = num % 10;
reversed = reversed * 10 + digit;
num /= 10;
}
println!("Reversed number: {}", reversed);
}
¶32. Rust Program to Check Whether a Number is a Palindrome or Not
use std::io;
fn is_palindrome(num: i32) -> bool {
let mut n = num;
let mut reversed = 0;
let original = num;
while n != 0 {
let digit = n % 10;
reversed = reversed * 10 + digit;
n /= 10;
}
reversed == original
}
fn main() {
let mut num = String::new();
println!("Enter a number: ");
io::stdin().read_line(&mut num).expect("Failed to read line");
let num: i32 = num.trim().parse().expect("Invalid input");
if is_palindrome(num) {
println!("{} is a palindrome.", num);
} else {
println!("{} is not a palindrome.", num);
}
}
¶34. Rust Program to Display Prime Numbers Between Intervals
use std::io;
fn is_prime(num: u32) -> bool {
if num <= 1 {
return false;
}
for i in 2..=(num / 2) {
if num % i == 0 {
return false;
}
}
true
}
fn main() {
let mut lower = String::new();
let mut upper = String::new();
println!("Enter the lower interval: ");
io::stdin().read_line(&mut lower).expect("Failed to read line");
println!("Enter the upper interval: ");
io::stdin().read_line(&mut upper).expect("Failed to read line");
let lower: u32 = lower.trim().parse().expect("Invalid input");
let upper: u32 = upper.trim().parse().expect("Invalid input");
println!("Prime numbers between {} and {}:", lower, upper);
for num in lower..=upper {
if is_prime(num) {
print!("{} ", num);
}
}
println!();
}
¶35. Rust Program to Check whether the input number is a Neon Number
use std::io;
fn is_neon_number(num: u32) -> bool {
let square = num * num;
let mut sum = 0;
let mut n = square;
while n != 0 {
sum += n % 10;
n /= 10;
}
sum == num
}
fn main() {
let mut num = String::new();
println!("Enter a number: ");
io::stdin().read_line(&mut num).expect("Failed to read line");
let num: u32 = num.trim().parse().expect("Invalid input");
if is_neon_number(num) {
println!("{} is a neon number.", num);
} else {
println!("{} is not a neon number.", num);
}
}
¶36. Rust Program to Find All Factors of a Natural Number
use std::io;
fn main() {
let mut num = String::new();
println!("Enter a natural number: ");
io::stdin().read_line(&mut num).expect("Failed to read line");
let num: u32 = num.trim().parse().expect("Invalid input");
println!("Factors of {} are:", num);
for i in 1..=num {
if num % i == 0 {
println!("{}", i);
}
}
}
¶37. Rust Program to Sum of Fibonacci Numbers at Even Indexes up to N Terms
use std::io;
fn main() {
let mut n = String::new();
println!("Enter the number of Fibonacci terms (N): ");
io::stdin().read_line(&mut n).expect("Failed to read line");
let n: u32 = n.trim().parse().expect("Invalid input");
let mut sum = 0;
let mut fib_prev = 0;
let mut fib_curr = 1;
for i in 1..=n {
if i % 2 == 0 {
sum += fib_curr;
}
let fib_next = fib_prev + fib_curr;
fib_prev = fib_curr;
fib_curr = fib_next;
}
println!("Sum of Fibonacci numbers at even indexes: {}", sum);
}
¶38. File I/O Read and write to files.
use std::fs::File;
use std::io::{self, Read, Write};
fn main() -> io::Result<()> {
let mut file = File::create("example.txt")?;
file.write_all(b"Hello, Rust!")?;
let mut content = String::new();
File::open("example.txt")?.read_to_string(&mut content)?;
println!("File content: {}", content);
Ok(())
}
These examples cover a range of basic concepts in Rust programming. As you progress, you can explore more advanced topics like ownership, lifetimes, traits, and libraries in Rust to build more complex applications.
PHP If-else-elseif and Switch-case
PHP String Functions - All necessary String functions in PHP to manage strings better.
Popular Tutorials
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development