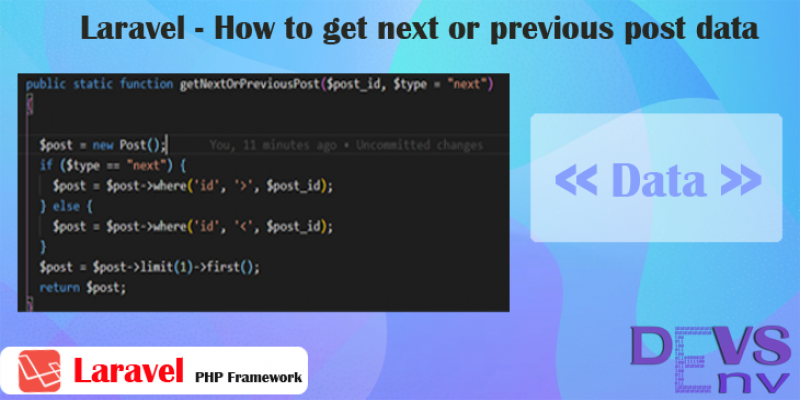
Laravel - How to get next or previous post data
In this article, we'll learn how to get the next and previous post data for a post or an article modal or any types of modal.
/**
* getNextOrPreviousPost
*
* @param integer $post_id Post/Article/Modal ID
* @param string $type Type of post, next/previous
* @return object The previous or next post according to any post
*/
public static function getNextOrPreviousPost($post_id, $type = "next")
{
$post = new Post();
if ($type == "next") {
$post = $post->where('id', '>', $post_id);
} else {
$post = $post->where('id', '<', $post_id);
}
$post = $post->limit(1)->first();
return $post;
}
Or, If we want to make it in Laravel DB Query Builder:
public static function getNextOrPreviousPost($post_id, $type = "next")
{
if ($type == "next") {
$post = DB::table('posts')->where('id', '>', $post_id);
} else {
$post = DB::table('posts')->where('id', '<', $post_id);
}
$post = $post->limit(1)->first();
return $post;
}
Details:
params 1:
In first parameter, we've get the post_id or model's primary_id.
params 2:
In second parameter, we've passed the type of getting data
return:
returns the object of the required post/model's data
Logic Bihind How to get next or previous post data:
- First check the type
- If type is next, get the greater than post of this post
- If type is previous, get the less than post of this post
- Then fetch as limit 1 and get the first data
PHP If-else-elseif and Switch-case
PHP String Functions - All necessary String functions in PHP to manage strings better.
Popular Tutorials
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development