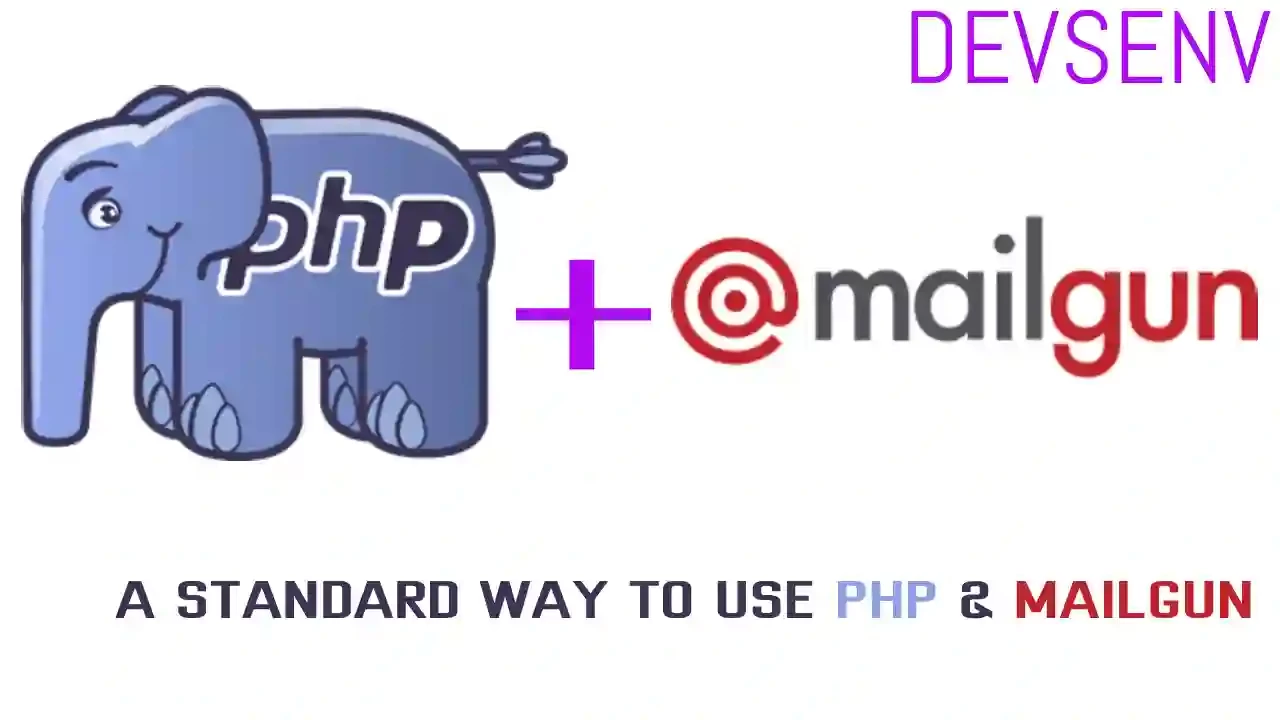
Mailgun-PHP Setup and Standard Class to Use easily Mailgun with PHP
Mailgun is an awesome Outgoing or Incoming Email Gateway. And with the help of PHP, we can use this very easily to our scalable project architecture.
Let's integrate Mailgun into our PHP application easily.
Create a Mailgun Account:
Create a mailgun account first - https://www.mailgun.com
Get API & Other Credentials
After Login, Go to Dashboard - https://app.mailgun.com/app/dashboard
You'll be able to find these staff -
- Mailgun sending domains - https://app.mailgun.com/app/dashboard
- Domain Look like -
sandboxce25c035e9664f82a05ee6e1380a9821.mailgun.org
- Domain Look like -
- Mailgun Private API Keys - https://app.mailgun.com/app/account/security/api_keys
- API Keys look like this -
key-e991f0b2d4f882e7acf457ccaedc52caa
- API Keys look like this -
Note: The above data is a demo, you'll find your data.
Don't make also Whitelist IP. If you do that, read docs first. It can give invalid credential error.
Mailgun Official Doc - https://github.com/mailgun/mailgun-php
Install Mailgun composer:
To integrate mailgun with PHP, we have to install mailgun-php
sdk and a http-client
.
// Mailgun PHP SDK
composer require mailgun/mailgun-php
// HTTP Client
composer require php-http/zend-adapter
Now an easy way to send email inside PHP -
require 'vendor/autoload.php';
use Mailgun\Mailgun;
# Instantiate the client.
$mgClient = new Mailgun('key-e991f0b2d4f882e7acf457ccaedc52caa'); // 'YOUR_API_KEY'
$domain = "sandboxce25c035e9664f82a05ee6e1380a9821.mailgun.org"; // "YOUR_DOMAIN_NAME"
$from_email = "manirujjamanakash@gmail.com";
$to_email = "afia@gmail.com";
$subject = "This is a mail using Mailgun";
$message = "Testing some Mailgun awesomness!";
# Make the call to the client.
$result = $mgClient->sendMessage($domain, array(
'from' => $from_email,
'to' => $to_email,
'subject' => $subject,
'text' => $message
));
Done, you can now check your email and it's received.
Another Approach for PRO Developers:
Make a Global Usable Helper Class for Mailgun Email:
Now, I'll create an awesome dynamic class to use mailgun easily in our project.
Class Email_Mailgun
:
<?php
// namespace App; // If you use namespace, then use,otherwise just comment it out
use Exception;
use Mailgun\Mailgun;
use Mailgun\Message\MessageBuilder;
/**
* Mailgun Email Class
*
* Send Outgoing Emai for WP ERP
*
* @since 1.0.0
*/
class Email_Mailgun {
/**
* Private API Key
*
* @var $private_api_key
*/
private $private_api_key;
/**
* Region Host Address
*
* @var $region
*/
public $region;
/**
* Domain Name
*
* @var $domain
*/
public $domain;
/**
* Mailgun Instance
*
* @var $mailgun instance
*/
protected $mailgun;
/**
* Message Builder Instance
*
* @var $builder instance
*/
protected $builder;
/**
* Class contsructor
*
* @param string $private_api_key key-xxx
* @param string $region api.mailgun.net
* @param string $domain sandboxxxx.mailgun.org
*/
public function __construct( $private_api_key, $region, $domain ) {
$this->private_api_key = $private_api_key;
$this->region = $region;
$this->domain = $domain;
$this->init();
}
/**
* Init Mailgun Instance
*
* @since 1.0.0
*
* @return void
*/
public function init() {
$this->mailgun = Mailgun::create( $this->private_api_key, "https://$this->region" );
$this->builder = new MessageBuilder();
}
/**
* Set Email Subject
*
* @since 1.0.0
*
* @param string $subject
*
* @return void
*/
public function set_subject( $subject ) {
if ( ! empty( $subject ) ) {
$subject = sanitize_text_field( wp_unslash( $subject ) );
$this->builder->setSubject( $subject );
}
}
/**
* Set Email Message
*
* @since 1.0.0
*
* @param string $message
*
* @return void
*/
public function set_message( $message ) {
if ( ! empty( $message ) ) {
$message = sanitize_text_field( wp_unslash( $message ) );
$this->builder->setTextBody( $message );
}
}
/**
* Set Email From Address
*
* @since 1.0.0
*
* @param array $from address_array example; `[ 'email' => 'test@example.com', 'name' => 'Jhon Doe' ]`
*
* @return void
*/
public function set_from_address( $from = [] ) {
if ( ! empty( $from['email'] ) ) {
$this->builder->setFromAddress(
$from['email'],
[
'first' => $from['name']
]
);
}
}
/**
* Set Email To Address
*
* @since 1.0.0
*
* @param array $to address_array example; `[ 'email' => 'test@example.com', 'name' => 'Jhon Doe' ]`
*
* @return void
*/
public function set_to_address( $to = [] ) {
if ( ! empty( $to['email'] ) ) {
$this->builder->addToRecipient(
$to['email'],
[
'first' => $to['name']
]
);
}
}
/**
* Set Email CC Address
*
* @since 1.0.0
*
* @param array $cc address_array example; `[ 'email' => 'test@example.com', 'name' => 'Jhon Doe' ]`
*
* @return void
*/
public function set_cc_address( $cc = [] ) {
if ( ! empty( $cc['email'] ) ) {
$this->builder->addCcRecipient(
$cc['email'],
[
'first' => $cc['name']
]
);
}
}
/**
* Set Email Custom Headers
*
* @since 1.0.0
*
* @param array $header address_array example; `[ 'key' => 'Custom-ID', 'name' => '123456' ]`
*
* @return void
*/
public function set_custom_headers( $header = [] ) {
if ( ! empty( $header['key'] ) && ! empty( $header['value'] ) ) {
$this->builder->addCustomHeader( $header['key'], $header['value'] );
}
}
/**
* Set Email Attachment
*
* @since 1.0.0
*
* @param string attachment file
*
* @return void
*/
public function set_attachment( $attachment ) {
if ( ! empty( $attachment ) ) {
$this->builder->addAttachment( $attachment );
}
}
/**
* Make Message
*
* Build a mailgun message with the data
*
* @since 1.0.0
*
* @param array $args
*
* @todo Add all supported features of mailgun if missed any
*
* @return void
*/
public function make_message( $args = [] ) {
$default = [
'subject' => '',
'from_address' => ['email' => '', 'name' => ''],
'to_address' => ['email' => '', 'name' => ''],
'cc_address' => ['email' => '', 'name' => ''],
'customer_header' => ['key' => '', 'value' => ''],
'attachment' => '',
'message' => ''
];
$data = wp_parse_args( $args, $default );
$this->set_subject( $data['subject'] );
$this->set_from_address( $data['from_address'] );
$this->set_to_address( $data['to_address'] );
$this->set_cc_address( $data['cc_address'] );
$this->set_custom_headers( $data['customer_header'] );
$this->set_attachment( $data['attachment'] );
$this->set_message( $data['message'] );
}
/**
* Send a Mailgun Message
*
* @since 1.0.0
*
* @param array $data A complete dataset of the message
*
* @return mixed
*/
public function send_email( $data = [] ) {
try {
$this->make_message( $data );
return $this->mailgun->messages()->send(
$this->domain,
$this->builder->getMessage()
);
} catch ( Exception $e ) {
throw new Exception( $e->getMessage() );
}
}
}
Now send through any of your PHP Code -
use App\Email_Mailgun; // It can be your path
try {
$private_api_key = "key-xxxx"; // Your Key
$region = "api.mailgun.net"; // For US - api.mailgun.net, For EU - api.eu.mailgun.net
$domain = "sandbox-xxx.mailgun.org"; // Domain Name
$mailgun = new Email_Mailgun( $private_api_key, $region, $domain );
$from_email = "manirujjamanakash@gmail.com";
$to_email = "afia@gmail.com";
$subject = "This is a mail using Mailgun";
$message = "Testing some Mailgun awesomeness!";
$data = [
'subject' => $subject,
'from_address' => ['email' => $from_email, 'name' => ''],
'to_address' => ['email' => $to_email, 'name' => ''],
'message' => $message
];
$mailgun->send_email( $data );
echo "Email sent successfully !";
} catch ( \Exception $e ) {
echo $e->getMessage();
}
That's it. You've sent the mail. You can also use many optional parameters, here, like so -
$data = [
'subject' => $subject,
'from_address' => ['email' => $from_email, 'name' => ''],
'to_address' => ['email' => $to_email, 'name' => ''],
'cc_address' => ['email' => $to_email, 'name' => ''],
'custom_header'=> ['key' => "Customer-ID", 'value' => '123'],
'attachment'. => "path file url",
'message' => $message
];
I hope, it's enough to do work with Mailgun and PHP. Thanks for staying with devsEnv.
Reference Links -
- Mailgun PHP - https://github.com/mailgun/mailgun-php
- Full Docs - https://documentation.mailgun.com/en/latest/api-intro.html#introduction
- Custom Message Builder - https://github.com/mailgun/mailgun-php/blob/master/src/Message/README.md
PHP If-else-elseif and Switch-case
PHP String Functions - All necessary String functions in PHP to manage strings better.
Popular Tutorials
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development