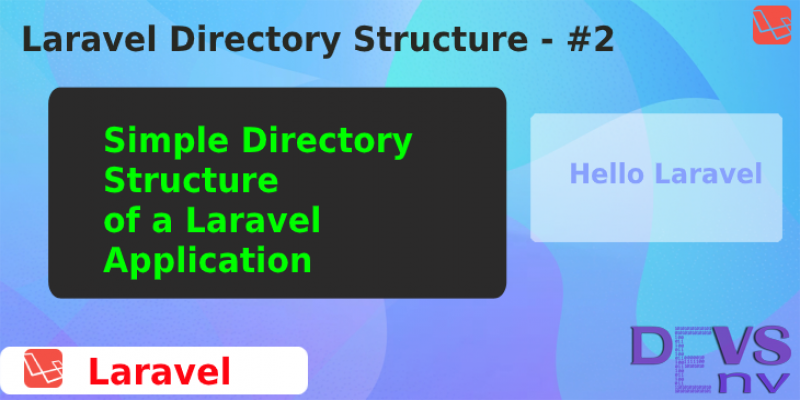
#2 Directory Structure of Laravel Application
Directory Structure
Directory structure is important to understand the basic structure of any framework and it's data flow.
We'll understand the basic directory structure of Laravel in this article.
Here is the simple directory structure of Laravel Application after it's first installation.
So, focus on every directory/files of a laravel project, one by one.
#app
app folder is the mother of a laravel application. All of the Models and Controller logic goes in this folder. Here is a full details of app folder with every file and folder's demonstration -
See the above image and description in right side to clearify and here I'm demonstrating the most important ones.
app/Http/Controller
In the Controllers folder under Http
folder, all of the Controllers are present, which is for MVC's Controller.
There is a basic Controller clss, called Controller.php
and all of the other controllers extend thia class's propertiesand methods. We write our logic here.
To send data from controller to view, we've to use this controller. We assign here any data, variables and send them to laravel views with some of the laravel built in functions with()
, compact()
etc.
app/User.php
Inside the app folder root, by default, we'll find a User.php file which consist a basic Model for User.Where a basic model structure is defined. In here, inside a User model, we'll see it's extend the Base Model class of Laravel. Any basic modfies, custom database table name, hidden attributes, relationships are defined in this Model class.
So, we come to the concept of Model of MVC Framework here. User is that model.
However, we can change our models to a specific Models folder also, which makes our code more cleaner and beautiful. A Basic User Class is like this -
<?php
namespace App;
use Illuminate\Contracts\Auth\MustVerifyEmail;
use Illuminate\Foundation\Auth\User as Authenticatable;
use Illuminate\Notifications\Notifiable;
class User extends Authenticatable
{
use Notifiable;
/**
* The attributes that are mass assignable.
*
* @var array
*/
protected $fillable = [
'name', 'email', 'password',
];
/**
* The attributes that should be hidden for arrays.
*
* @var array
*/
protected $hidden = [
'password', 'remember_token',
];
/**
* The attributes that should be cast to native types.
*
* @var array
*/
protected $casts = [
'email_verified_at' => 'datetime',
];
}
#bootstrap
Bootstrap folder is responsible to initialize/bootstrap the application.
There is a file called app.php
, which will do the tasks.
To develop a project, we don't have to put code here initially.
The app file starts like this -
<?php
/*
|--------------------------------------------------------------------------
| Create The Application
|--------------------------------------------------------------------------
|
| The first thing we will do is create a new Laravel application instance
| which serves as the "glue" for all the components of Laravel, and is
| the IoC container for the system binding all of the various parts.
|
*/
$app = new Illuminate\Foundation\Application(
$_ENV['APP_BASE_PATH'] ?? dirname(__DIR__)
);
// ... More
#config
Config Folder is resnponsible all of the configurations like, survice, app classes, database, constants, mail, session, auth, view, cache, cors. All of the configuration are in the specific file name, so there name is self descriptive.
To develop a project, we don't have to put code here initially.
#database
Database folder is very important for a laravel project and it's actually needed to create, update database tables, insert dummy data.
database/migrations:
To create a new table or any indexing, or any query execution, we've to put that in database/migrations
folder in Laravel.
Suppose, we want a basic table called posts
in database, then
- We've to create a migration first, and that will store in this folder by this command -
php artisan make:migration create_posts_table
. - It'll create a migrations file like this - 2020_08_23_123020_create_posts_table.php , where the date and time is dependent on your time period.
- Then when we run
php artisan migrate
command, this migrations file logic will store in the migrations folder.
database/seeders:
To create dummy data in database, we've to use this seeder. By using seeder, we can insert/update dummy data in database.
If there is no initialize of empty data insert, there is no use of this seeder.
#public
Public folder is where we put our Stylesheets and Javascripts. And then, from view we've to link that. We don't have to addition work here. index.php
will handle autoloading the data here.
So, whenever, we hit an application, it will first comes here, index.php
in laravel.
#resources
Applications views, components, languages files are stored here.
resources/view/
Application every views are stored here. It'll fullfill the concept of Laravel's MVC(Model, View, Controller) pattern. By default, there is a file welcome.blade.php
exists there to give us the initial page view.
resources/lang/
In this folder, to manage multi language of our site, we could put different folder here like, en, as, in, then store our known variable in an array and use these in our application. It is helpful for multi language site.
#routes
Applications URL, routes are defined here.
resources/web.php
Web.php file manage the initial application's route management. A basic router is already initilized in the pre built web.php.
<?php
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
Which will just return a basic home page url, and return a view welcom.blade.ph for this.
#storage
Application encrypted or secured data will stored through here.
It is not necessary for a basic web application
#test
All test case will be declared here for our Laravel application
It is not necessary for a basic web application
#vendor
Application system files are stored here
It is not necessary to do anything here while development any project.
.env
All of the sites configurations are stored here. Like database credentials, mail credentials, pusher credentials, broadcasting credentials and so on. A Basic .env file is like this -
APP_NAME=Laravel
APP_ENV=local
APP_KEY=base64:SEjGcAu686ZZQORkIEodR2WdcPpqBBKjMxfIbEFoTrA=
APP_DEBUG=true
APP_URL=http://localhost
LOG_CHANNEL=stack
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel
DB_USERNAME=root
DB_PASSWORD=
BROADCAST_DRIVER=log
CACHE_DRIVER=file
QUEUE_CONNECTION=sync
SESSION_DRIVER=file
SESSION_LIFETIME=120
REDIS_HOST=127.0.0.1
REDIS_PASSWORD=null
REDIS_PORT=6379
MAIL_MAILER=smtp
MAIL_HOST=smtp.mailtrap.io
MAIL_PORT=2525
MAIL_USERNAME=null
MAIL_PASSWORD=null
MAIL_ENCRYPTION=null
MAIL_FROM_ADDRESS=null
MAIL_FROM_NAME="${APP_NAME}"
AWS_ACCESS_KEY_ID=
AWS_SECRET_ACCESS_KEY=
AWS_DEFAULT_REGION=us-east-1
AWS_BUCKET=
PUSHER_APP_ID=
PUSHER_APP_KEY=
PUSHER_APP_SECRET=
PUSHER_APP_CLUSTER=mt1
MIX_PUSHER_APP_KEY="${PUSHER_APP_KEY}"
MIX_PUSHER_APP_CLUSTER="${PUSHER_APP_CLUSTER}"
It is already well descriptive, so we already understood I think.
composer.json
All of the packages installed in Laravel project are added here. Whenever we run composer update
command, it will update the laravel vendor
folder with this packages.
server.php
Application to use with built in laravel server, this file is necessary and we dont't have to add anything here. We just run php artisan serve
and it'll create a server for us.
Official Documentation of Laravel about Directory Structure - https://laravel.com/docs/7.x/structure
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
14
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development