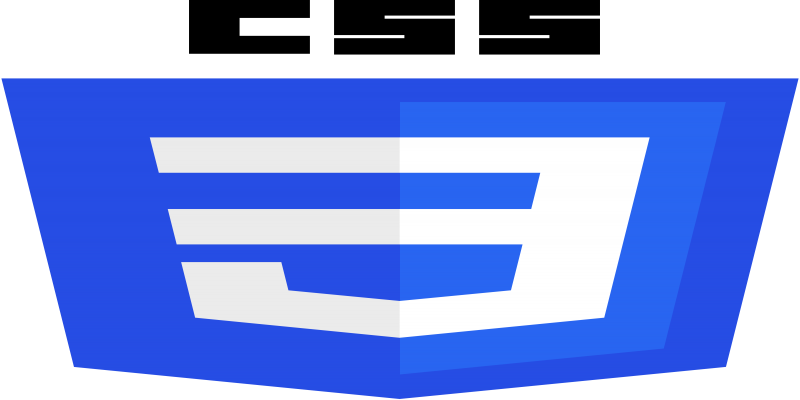
CSS
Crafting Responsive Designs: Images, Fonts, Width, and Height
Responsive design is an approach to web design that makes web pages render well on a variety of devices and window or screen sizes. Here’s an example using HTML and CSS to make an image, font, and container responsive:
¶Responsive Image:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
img {
max-width: 100%;
height: auto;
display: block;
margin: 0 auto;
}
</style>
<title>Responsive Example</title>
</head>
<body>
<img src="your-image.jpg" alt="Responsive Image">
</body>
</html>
Explanation:
1. max-width: 100%;
ensures that the image does not exceed the width of its container.
2. height: auto;
maintains the aspect ratio of the image.
3. display: block;
and margin: 0 auto; center the image within its container.
¶Responsive Font:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
body {
font-size: 16px;
}
@media (max-width: 600px) {
body {
font-size: 14px;
}
}
</style>
<title>Responsive Example</title>
</head>
<body>
<p>This is some text.</p>
</body>
</html>
¶Output (dekstop and mobile screen)


Explanation:
-
font-size: 16px;
sets the default font size. -
@media (max-width: 600px)
applies the styles inside the block only when the screen width is 600 pixels or less.
¶Responsive Container (Width):
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
.container {
max-width: 1200px;
margin: 0 auto;
padding: 20px;
}
</style>
<title>Responsive Example</title>
</head>
<body>
<div class="container">
<!-- Your content goes here -->
</div>
</body>
</html>
Explanation:
-
max-width: 1200px;
sets the maximum width of the container. -
margin: 0 auto;
centers the container on the page.
¶height as well:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
body {
font-family: 'Arial', sans-serif;
line-height: 1.6;
margin: 0;
padding: 0;
}
.container {
max-width: 1200px;
margin: 0 auto;
padding: 20px;
overflow: hidden; /* Clearfix to contain floated elements */
}
img {
max-width: 100%;
height: auto;
display: block;
margin: 0 auto;
}
@media (max-width: 600px) {
body {
font-size: 14px;
}
.container {
padding: 10px;
}
}
</style>
<title>Responsive Example</title>
</head>
<body>
<div class="container">
<h1>Responsive Example</h1>
<img src="your-image.jpg" alt="Responsive Image">
<p>This is some text. It will adjust its font size on smaller screens, and the image will be responsive as well. The container will adapt its padding for better spacing.</p>
</div>
</body>
</html>
In this example:
- The body’s font-family, line-height, margin, and padding are set to provide a clean and consistent base.
- The
overflow: hidden;
property in the container is a clearfix technique to contain floated elements. - Inside the media query (
@media (max-width: 600px)
), the font size of the body is reduced, and the container’s padding is adjusted to provide a better layout on smaller screens. Feel free to replace the placeholder image (your-image.jpg
) and customize the content according to your needs. This example demonstrates a simple and responsive webpage that adjusts its layout based on the screen size.
Unlocking Responsive Design: CSS Strategies and Media Techniques
Elevate Your Layouts with Flex-Box and flex-direction: Row, Column
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development