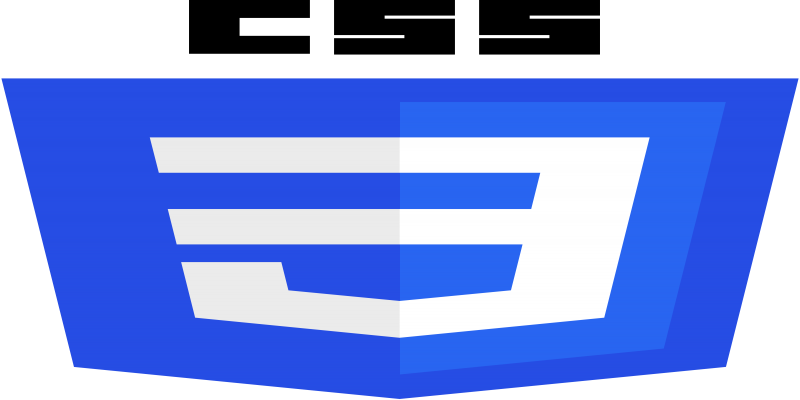
CSS
Mastering Flex-wrap, No-wrap, and Flex-flow for Dynamic CSS Layouts
flex-wrap
is a property in CSS that is used with Flexbox to control the wrapping of flex items within a flex container. The property can take three values: nowrap
, wrap
, and wrap-reverse
.
Here are the three values explained:
-
nowrap
: This is the default value. It means that all flex items will be forced into a single line, and the container will not wrap them onto multiple lines. -
wrap
: This value allows flex items to wrap onto multiple lines. The items will be placed in as many rows as necessary to accommodate all items. The order of the items is preserved. -
wrap-reverse
: Similar towrap
, but the wrapping occurs in the opposite direction. The first line is filled from the end to the beginning, and subsequent lines are filled in the reverse order.
Let’s look at an example to illustrate these values:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
.flex-container {
display: flex;
flex-wrap: nowrap; /* or wrap, or wrap-reverse */
}
.flex-item {
width: 100px;
height: 100px;
margin: 5px;
background-color: lightblue;
display: flex;
align-items: center;
justify-content: center;
font-weight: bold;
font-size: 16px;
}
</style>
</head>
<body>
<h2>flex-wrap: nowrap (default)</h2>
<div class="flex-container">
<div class="flex-item">1</div>
<div class="flex-item">2</div>
<div class="flex-item">3</div>
<div class="flex-item">4</div>
<div class="flex-item">5</div>
</div>
<h2>flex-wrap: wrap</h2>
<div class="flex-container" style="flex-wrap: wrap;">
<div class="flex-item">1</div>
<div class="flex-item">2</div>
<div class="flex-item">3</div>
<div class="flex-item">4</div>
<div class="flex-item">5</div>
</div>
<h2>flex-wrap: wrap-reverse</h2>
<div class="flex-container" style="flex-wrap: wrap-reverse;">
<div class="flex-item">1</div>
<div class="flex-item">2</div>
<div class="flex-item">3</div>
<div class="flex-item">4</div>
<div class="flex-item">5</div>
</div>
</body>
</html>
¶Output:

In this example, the .flex-container class represents a flex container, and each .flex-item represents a flex item. You can experiment with the flex-wrap property by changing its value in the CSS to see how it affects the layout of the flex items.
flex-flow
property in CSS is a shorthand property that combines the flex-direction
and flex-wrap
properties. It’s used with Flexbox to set the direction of the flexible items and whether the items should wrap or not.
Here is the syntax for flex-flow
:
css
flex-flow: flex-direction flex-wrap;
-
flex-direction
: This property defines the direction of the main axis, which determines the direction in which the flex items are placed. It can take values likerow
,row-reverse
,column
, orcolumn-reverse
. -
flex-wrap
: This property defines whether the flex items should wrap onto multiple lines or not. It can take values likenowrap
(default),wrap
, orwrap-reverse
.
Here’s an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
.flex-container {
display: flex;
border: 1px solid #ccc;
padding: 10px;
}
.flex-item {
width: 100px;
height: 100px;
background-color: lightblue;
margin: 5px;
display: flex;
align-items: center;
justify-content: center;
font-weight: bold;
}
</style>
</head>
<body>
<div class="flex-container" style="flex-flow: row nowrap;">
<div class="flex-item">1</div>
<div class="flex-item">2</div>
<div class="flex-item">3</div>
</div>
<div class="flex-container" style="flex-flow: column wrap;">
<div class="flex-item">A</div>
<div class="flex-item">B</div>
<div class="flex-item">C</div>
<div class="flex-item">D</div>
</div>
</body>
</html>
¶Output:

The first flex container has a row direction and nowrap value for flex-flow
, meaning the items will be in a single row and won’t wrap.
The second flex container has a column direction and wrap value for flex-flow
, meaning the items will be in a column and will wrap to the next line if necessary.
You can modify the values of flex-direction and flex-wrap
to see how the layout changes.
Elevate Your Layouts with Flex-Box and flex-direction: Row, Column
Fine-Tuning Flex-shrink and Flex-basis for Responsive CSS Layouts
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development