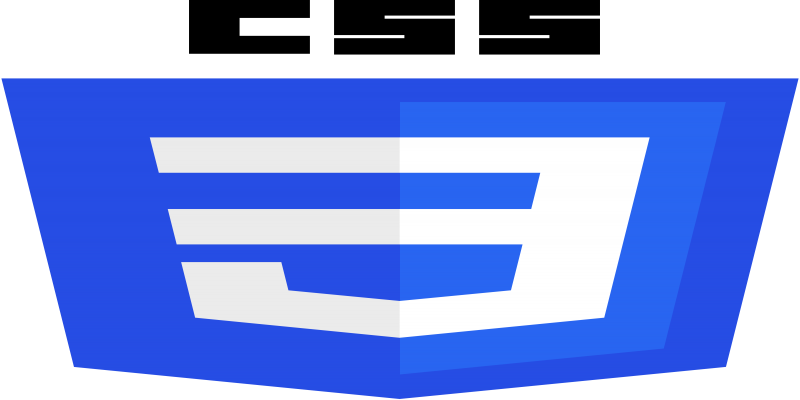
CSS
CSS Grid Properties - Grid-auto-rows with Practical examples
¶Property details - grid-auto-rows
The grid-auto-rows
property in CSS is used in a grid container to set the size of implicit rows (rows that are created by the grid but not explicitly defined using the grid-template-rows
property). The minmax()
function allows you to define a size range for the rows.
Let’s break down grid-auto-rows: minmax(100px, auto):
minmax(100px, auto)
: This function sets a size range for the rows. It specifies that the minimum size of the row is 100px
, and the maximum size is set to auto
, meaning the row can grow to accommodate its content.
Now, let’s see an example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<style>
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
grid-gap: 10px;
grid-auto-rows: minmax(100px, auto);
}
.grid-item {
background-color: #3498db;
color: #fff;
padding: 10px;
text-align: center;
}
</style>
</head>
<body>
<div class="grid-container">
<div class="grid-item">Item 1</div>
<div class="grid-item">Item 2</div>
<div class="grid-item">Item 3</div>
<div class="grid-item">Item 4</div>
<div class="grid-item">Item 5</div>
<div class="grid-item">Item 6</div>
</div>
</body>
</html>
¶Output:

The .grid-container
is a grid container with three columns (grid-template-columns: repeat(3, 1fr)) and a grid gap of 10px.
The .grid-auto-rows: minmax(100px, auto)
sets the minimum row height to 100px and allows the rows to expand as needed (auto).
The items inside the grid will adjust their size based on the content, and if the content is larger than 100px, the row will expand accordingly. This is especially useful when you want rows to be a minimum height but still be able to grow based on content.
CSS Grid Properties - Gap, Grid Column Gap, Grid Template Columns
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
13
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development