
React Js
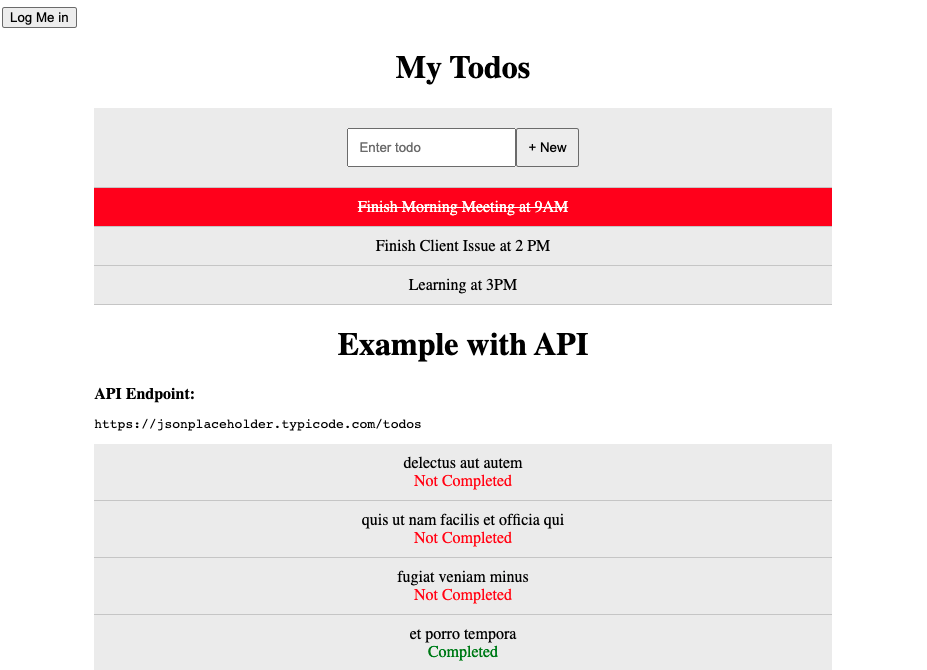
React Context API - An Advanced Introduction and Complete Examples
Hi, Today I'll be going to show you an Advanced Introduction of React New Context API with most of the topics. Let's start to Learn -
Who is eligible to Start
I assume you are very much familiar with React component. You know how the component works and for which problem to solve the React Context API arrives.
In a short - React Context API arrives to solve the Data Passing Redundancy problem to nth level child or parent component. So, it removes the unnecessary props drilling in React JS.
What has been Added
We've added/implemented the following things -
- React Context API
- Multiple Reducer
- AuthReducer - Manage Authentication Parts
- TodoReducer - Manage Todo Parts
- PostReducer - Manage Post Parts
- RootReducer - Combine All of the 3 Reducers into one. and it's scalable at much as reducer needs.
- Good File Structure for Context Provider Setup
- Good File Structure for Component Setup
- Manage all values from a single Store
- Follow Single Source of Truth.
Let's Begin to start the context API implementation on our own and really advanced one.
Start a React Project
You could start with a fresh React Project. In my case, I've done this in Code-Sandbox.
npx create-react-app context-example
Now, Install only one package - Node Sass, as we're using Sass/Scss in our project. And rest of the are automatically included using the default CRA setup.
cd context-example
npm i node-sass
Before doing something great, let's check our file/folder structure for this React Context API Setup -
;
Add Store to Manage All Data
Inside src/store/Store.js file - Add this
import React, { createContext, useContext, useReducer } from "react";
// Create a context and make it in Store
const Store = createContext();
// Make Our StoreProvider which will actually used as HOC
export const StoreProvider = ({ children, initialState, reducer }) => {
const value = useReducer(reducer, initialState);
return {children};
};
// `useStore` A Custom Hook implementation to get context Store data's
export function useStore() {
return useContext(Store);
}
- We make a
Store
variable and put a React context instance there. - We make a
StoreProvider
which will be used as a wrapper of all of the child components. Inside StoreProvider, we'll actually handle the incoming reducers passed here. - And Next, we've made a Custom Hook -
useStore()
, so that we don't need to makeuseContext
in every page of our project.
Wait, The article will be continued soon. In that time, you can check the source of Code-Sandbox link.
Final Demo of React Context API
Form Submission and File Upload in PHP - Basic to Advance Level Form Submission in PHP
Fine-Tuning Flex-shrink and Flex-basis for Responsive CSS Layouts
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
14
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development