
React Js
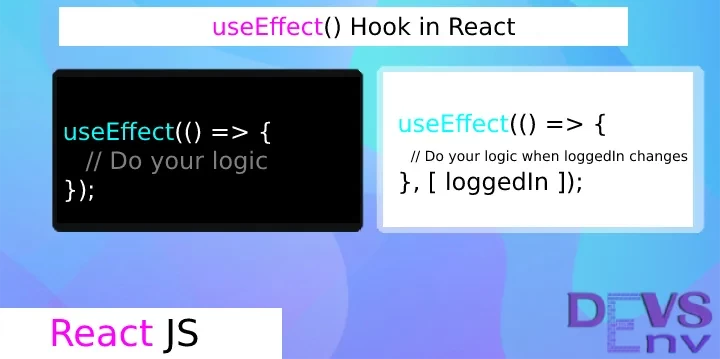
React Hooks - UseEffect() - Complete introduction about react useEffect hook
What is useEffect Hook:
If anything changes happend and needs side effect on your component then useEffect
hook will be called.
Means - when Component
- Mounts,
- Unmount,
- Variable change,
- State change,
- Props change or
- any value changes of component, then this effect hook will work.
UseEffect
hook expects a function to do something inside of it.
Motivation of React useEffect() Hook
When we used in class based component. Some things we need to repeat our code. Like think of a counter app, which counts value should implement in two sections like -
componentDidMount()
andcomponentDidUpdate()
componentWillUnmount()
Look the example that in class based component -
import React, { Component } from React;
// ...
class Score extends Component{
this.state = {
score: 0
}
componentDidMount() {
// Search from API or process here...
document.title = "Current Score: " + this.state.score;
}
componentDidUpdate() {
document.title = "Current Score: " + this.state.score;
}
render() {
return `Final Score: {this.state.score}`;
}
}
To mitigate these issues in class based component
- Remove redundant code and
- Make same functionality in one place,
useEffect arrived in react. And it has also many more benefits. Let's dive into React useEffect
().
How to create a basic useEffect Hook:
import { useEffect } from 'react';
useEffect(() => {
// Do your logic
});
Key points of useEffect Hook -
useEffect() Render Every time
It can accept additional n number of params in it's callback function's second argument.
Argument = []
- Empty array - Render only single time in its life lifecycle
- Used in - Select box Options data,
- Used in - Static data which will not change during render
Example Code View -
useEffect(() => {
// Do your logic for first render
}, []);
Example with database call and set data -
useEffect(() => {
fetch('https://jsonplaceholder.typicode.com/todos')
.then(response => response.json())
.then(json => setTodos(json)); // setTodos() is a function to set todos
}, [])
Any other argument = [loggedIn]
- Changes only when loggedIn change
- Single Data
Example Code view -
useEffect(() => {
// Do your logic for only when loggedIn changes
}, [loggedIn]);
Multiple data with comma
- [loggedIn, loggedMessage]
Example code view -
useEffect(() => {
// Do your logic for only when loggedIn and loggedInMessage changes
}, [loggedIn, loggedInMessage]);
When to use multiple useEffect Hook:
In a scenerio,
- When some side effect needs for one and some needs for other. Then it's important
- To segregate functions among component
Example code view of multiple useEffect() -
// Effect Hook 1
useEffect(() => {
// Do your first time logic to get api data
}, []);
// Effect Hook 2
useEffect(() => {
// Do your logic for only when loggedIn and loggedInMessage changes
}, [loggedIn, loggedInMessage]);
How and When needs to clean up useEffect Hook:
When, component would be unmounted, it's need to clean up something. Then we should do that inside useEffect with a return();
Example 1 of Cleanup useEffect:
- API Subscribe inside useEffect()
- Remove subscribe functionality after return
Example 2 of Cleanup useEffect:
- Event listener start on change something.
- Need to remove or stop event listener when component deleted.
Example code view -
const [count, setCount] = useState(window.innerWidth);
const calculateWindowSize = () => {
setCount(window.innerWidth)
}
useEffect(() => {
window.addEventListener('resize', calculateWindowSize);
return () => {
window.removeEventListener('resize', calculateWindowSize);
}
}, []);
Download Source Code From Github - https://github.com/ManiruzzamanAkash/ReactBasic2Pro/tree/day22
PHP If-else-elseif and Switch-case
PHP String Functions - All necessary String functions in PHP to manage strings better.
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
13
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development