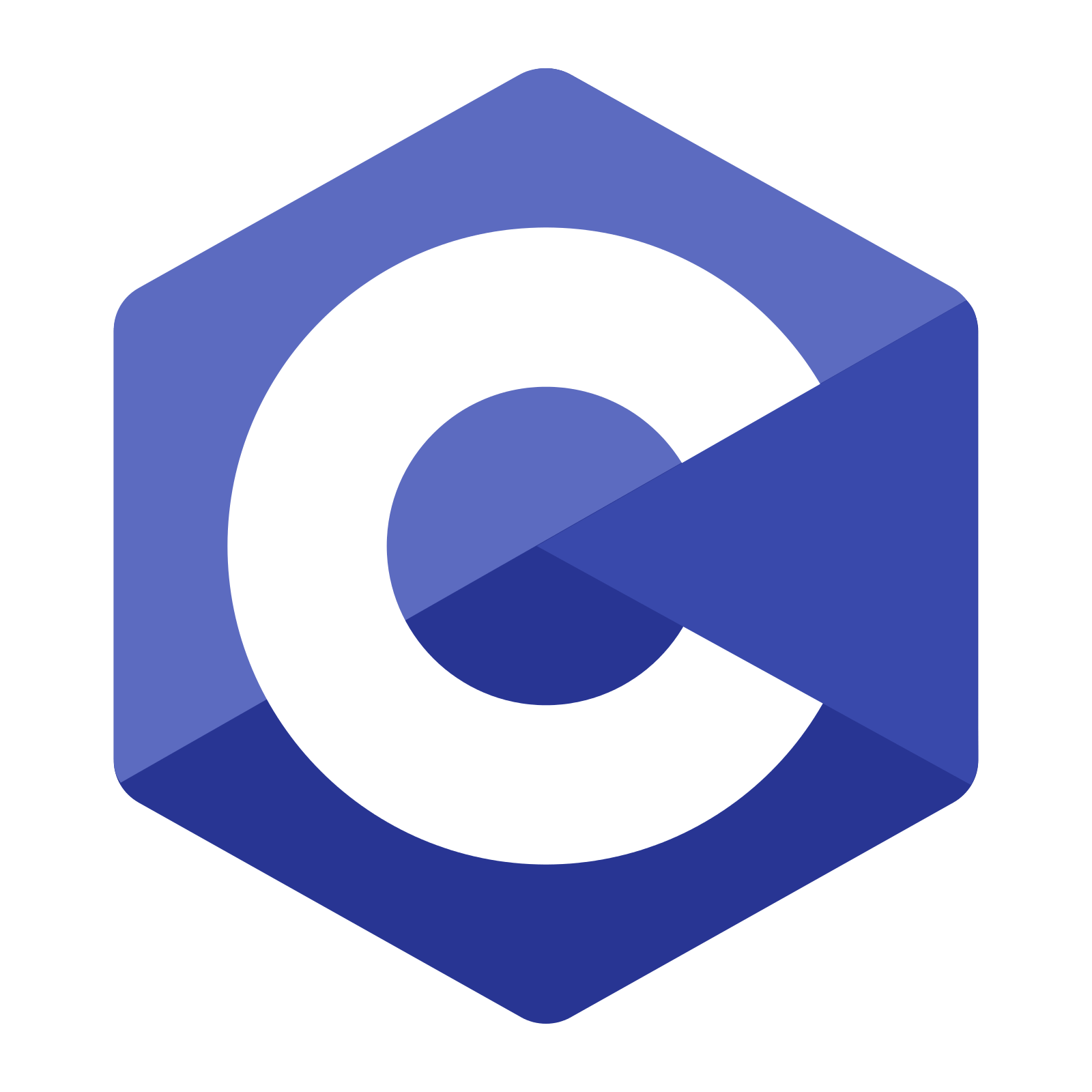
C Programming
Conditional in C Programming - if-else, elseif, switch-case
- What is if-else
- Example
- What is Switch
- Example
- Example 2 - Vowel check using if-else
- Example 3 - Check if a number is positive or not using c programming
C programming has a rich history, dating back to the early days of computer science. One of the key features of the C programming language is the ability to use conditional statements to control the flow of a program.
¶What is if-else
The “if-else” statement is a fundamental building block of C programming, allowing developers to execute different blocks of code based on the outcome of a Boolean expression.
The syntax for an “if-else” statement in C is as follows:
if (expression)
{
// code to be executed if expression is true
}
else
{
// code to be executed if expression is false
}
¶Example
For example, consider a program that checks the value of a variable “x” and prints a message depending on whether “x” is greater than, less than, or equal to 10:
if (x > 10)
{
printf("x is greater than 10\n");
}
else if (x < 10)
{
printf("x is less than 10\n");
}
else
{
printf("x is equal to 10\n");
}
Complete Program of this greater and calculation -
#include <stdio.h>
int main()
{
int x = 5;
// check the value of x and print a message
if (x > 10)
{
printf("x is greater than 10\n");
}
else if (x < 10)
{
printf("x is less than 10\n");
}
else
{
printf("x is equal to 10\n");
}
return 0;
}
In this program, the “if-else” statement checks the value of the variable “x” and prints a message depending on whether “x” is greater than, less than, or equal to 10. The output of this program would be “x is less than 10”.
This is a simple example that demonstrates the basic syntax and usage of an “if-else” statement in C. In a more complex program, the “if-else” statement could be used in combination with other control flow statements and nested within other “if-else” statements to implement more sophisticated logic.
¶What is Switch
The switch
statement is a control structure in C that allows handling multiple conditions. It is useful when dealing with a series of constant values of a variable.
switch (var) {
case constant1:
// Code to be executed if expression equals constant1
break;
case constant2:
// Code to be executed if expression equals constant2
break;
// Additional cases as needed
default:
// Code to be executed if none of the cases match
}
Here, variable var
is evaluated, and its value is compared against each case. If a match is found, the block of code is executed.
The break statement is crucial to exit the switch block, otherwise it will continue executing subsequent cases. if none of the cases match, the default case is executed.
¶Example
#include <stdio.h>
int main() {
char ch;
scanf("%c", &ch);
switch (ch)
{
case 'a':
printf("It's a vowel\n");
break;
case 'e':
printf("It's a vowel\n");
break;
case 'i':
printf("It's a vowel\n");
break;
case 'o':
printf("It's a vowel\n");
break;
case 'u':
printf("It's a vowel\n");
break;
default:
printf("It's a consonant\n");
break;
}
return 0;
}
Here, we take a lowercase letter as input and check whether it’s a vowel or consonant.
¶Example 2 - Vowel check using if-else
Here is a simple C program that uses an “if-else” statement to check whether a given character is a vowel:
#include <stdio.h>
int main()
{
char ch = 'a';
// check if ch is a vowel
if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u')
{
printf("%c is a vowel\n", ch);
}
else
{
printf("%c is not a vowel\n", ch);
}
return 0;
}
In this program, the “if-else” statement checks whether the value of the variable “ch” is equal to any of the vowels ‘a’, ‘e’, ‘i’, ‘o’, or ‘u’. If the condition is true, the program prints a message saying that the character is a vowel. Otherwise, it prints a message saying that the character is not a vowel.
The output of this program would be “a is a vowel”. This is a simple example that demonstrates the use of an “if-else” statement to implement a basic conditional check in C programming.
¶Example 3 - Check if a number is positive or not using c programming
Here is a simple C program that uses an “if-else” statement to check whether a given number is positive:
#include <stdio.h>
int main()
{
int num = 10;
// check if num is a positive number
if (num > 0)
{
printf("%d is a positive number\n", num);
}
else
{
printf("%d is not a positive number\n", num);
}
return 0;
}
In this program, the “if-else” statement checks whether the value of the variable “num” is greater than 0. If the condition is true, the program prints a message saying that the number is positive. Otherwise, it prints a message saying that the number is not positive.
The output of this program would be “10 is a positive number”. This is a simple example that demonstrates the use of an “if-else” statement to implement a basic conditional check in C programming.
In conclusion, the “if-else” statement is a fundamental and essential feature of the C programming language. It allows developers to control the flow of a program based on the outcome of a Boolean expression, enabling them to execute different blocks of code depending on whether a certain condition is true or false. The “if-else” statement is versatile, powerful, and widely used in C programming, making it an indispensable tool for any C programmer.
Input and Output in C Programming
Loops in C Programming - for loop, while loop and do-while loop
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development