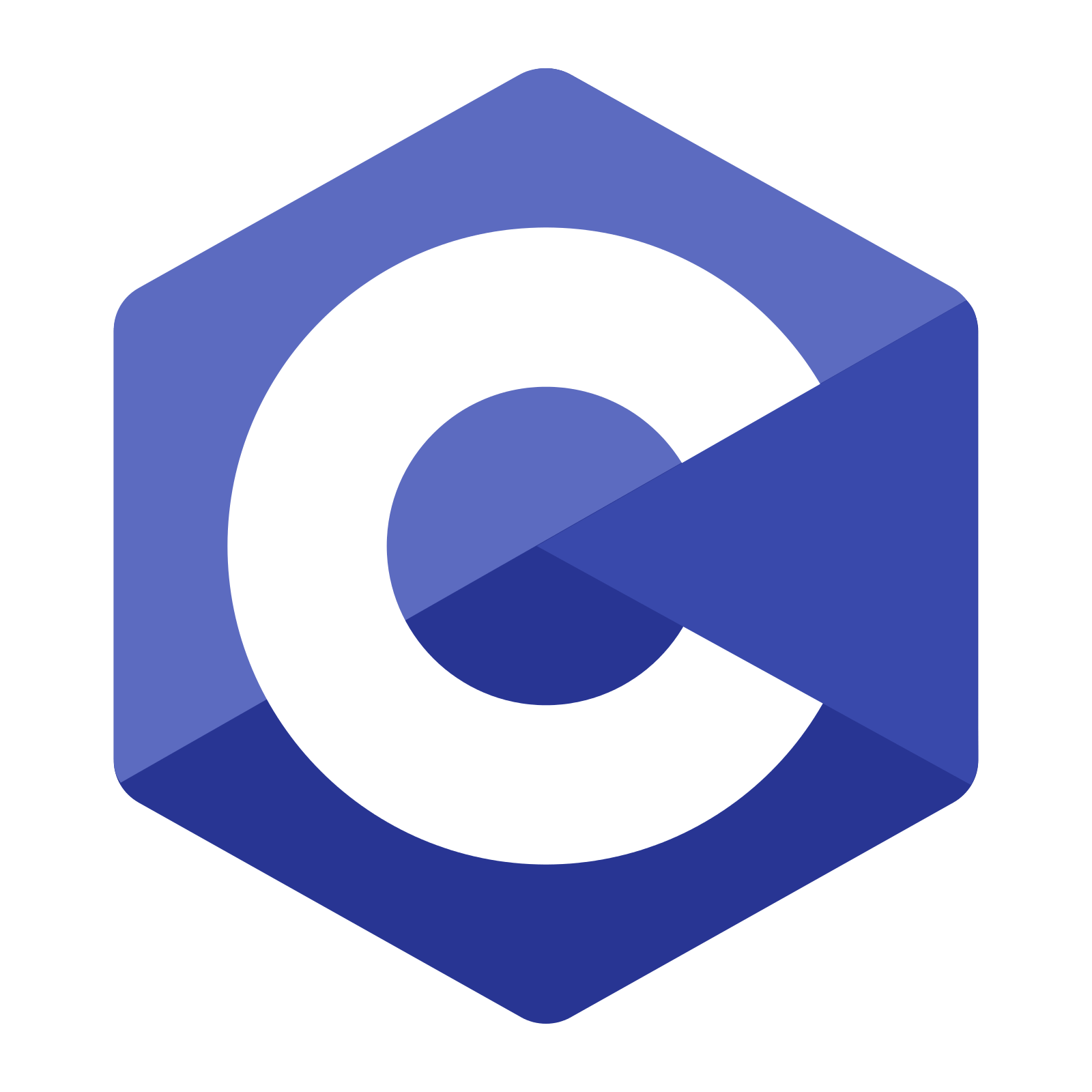
C Programming
Functions in C Programming - with Practical examples
- What is a Function in C Programming?
- How to Create a Function in C Programming
- Complete Example of a Function in C Programming
- Three Real-Life Coding Examples of Functions
Functions are an essential part of the C programming language. They allow programmers to break up their code into smaller, more manageable pieces and reuse them throughout their programs. In this article, we will discuss what functions are in C programming, how to create them, and provide a complete example of a function in C. We will also provide three real-life coding examples of functions in C to demonstrate their practical use.
¶What is a Function in C Programming?
In C programming, a function is a block of code that performs a specific task. Functions are often used to perform repetitive tasks, such as calculating the sum of two numbers or printing a message to the screen. They are also used to break up complex programs into smaller, more manageable pieces, making them easier to write and debug.
Functions have three main components: a name, a list of parameters, and a return type. The name of the function is used to identify it, and the parameters are the variables that are passed to the function when it is called. The return type specifies the data type of the value that the function returns. For example, a function might have a return type of int
if it returns an integer value.
¶How to Create a Function in C Programming
To create a function in C, you will need to use the “function header” and the “function body.” The function header is the first line of the function and includes the function name, parameters, and return type. The function body is the block of code that contains the instructions that the function will execute when it is called.
Here is an example of a function in C:
int addNumbers(int x, int y) {
int result = x + y;
return result;
}
In this example, the function is named addNumbers()
and it takes two integer parameters (x
and y
). The function has a return type of int
and it returns the result of adding x
and y
together.
¶Complete Example of a Function in C Programming
Here is a complete example of a function in C that demonstrates how to create a function and call it from the main program:
#include <stdio.h>
int addNumbers(int x, int y) {
int result = x + y;
return result;
}
int main() {
int a = 10;
int b = 20;
int c = addNumbers(a, b); // call the function
printf("The sum of a and b is: %d", c);
return 0;
}
In this example, the function “addNumbers” is defined and called from the main program. The function takes two integer parameters (x and y) and returns the result of adding them together.
¶Three Real-Life Coding Examples of Functions
Here are three real-life examples of functions in C that demonstrate their practical use:
¶Calculating the Factorial of a Number:
In this example, we will create a function that calculates the factorial of a given number. The function will take an integer parameter (n) and return the factorial of that number.
#include <stdio.h>
long long calculateFactorial(int n); // function prototype
int main() {
int num;
long long fact;
printf("Enter an integer to find its factorial: ");
scanf("%", );
d", &num);
fact = calculateFactorial(num); // call the function
printf("The factorial of %d is: %lld", num, fact);
return 0;
}
long long calculateFactorial(int n) {
long long result = 1;
int i;
for (i = 1; i <= n; i++) {
result = result * i;
}
return result;
}
¶2. Converting Celsius to Fahrenheit:
In this example, we will create a function that converts a temperature from Celsius to Fahrenheit. The function will take a double parameter (celsius) and return the equivalent temperature in Fahrenheit.
#include <stdio.h>
double celsiusToFahrenheit(double celsius); // function prototype
int main() {
double celsius, fahrenheit;
printf("Enter a temperature in Celsius: ");
scanf("%lf", &celsius);
fahrenheit = celsiusToFahrenheit(celsius); // call the function
printf("%.2lf Celsius is equivalent to %.2lf Fahrenheit", celsius, fahrenheit);
return 0;
}
double celsiusToFahrenheit(double celsius) {
double fahrenheit = (celsius * 9.0 / 5.0) + 32.0;
return fahrenheit;
}
¶Generating a Random Number
In this example, we will create a function that generates a random number between a given range. The function will take two integer parameters (min and max) and return a random number within that range.
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int generateRandomNumber(int min, int max); // function prototype
int main() {
int min, max, random;
printf("Enter the minimum and maximum values for the random number: ");
scanf("%d%d", &min, &max);
random = generateRandomNumber(min, max); // call the function
printf("Your random number is: %d", random);
return 0;
}
int generateRandomNumber(int min, int max) {
srand(time(0)); // seed the random number generator
int random = rand() % (max - min + 1) + min;
return random;
}
In conclusion, functions are an important part of the C programming language. They allow programmers to break up their code into smaller, more manageable pieces and reuse them throughout their programs. By understanding how to create and use functions in C, you can write more efficient and effective code.
Loops in C Programming - for loop, while loop and do-while loop
Arrays in C Programming - Algorithm and Practical examples
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
18
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
SEO - Search Engine Optimization
1
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- SEO
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development