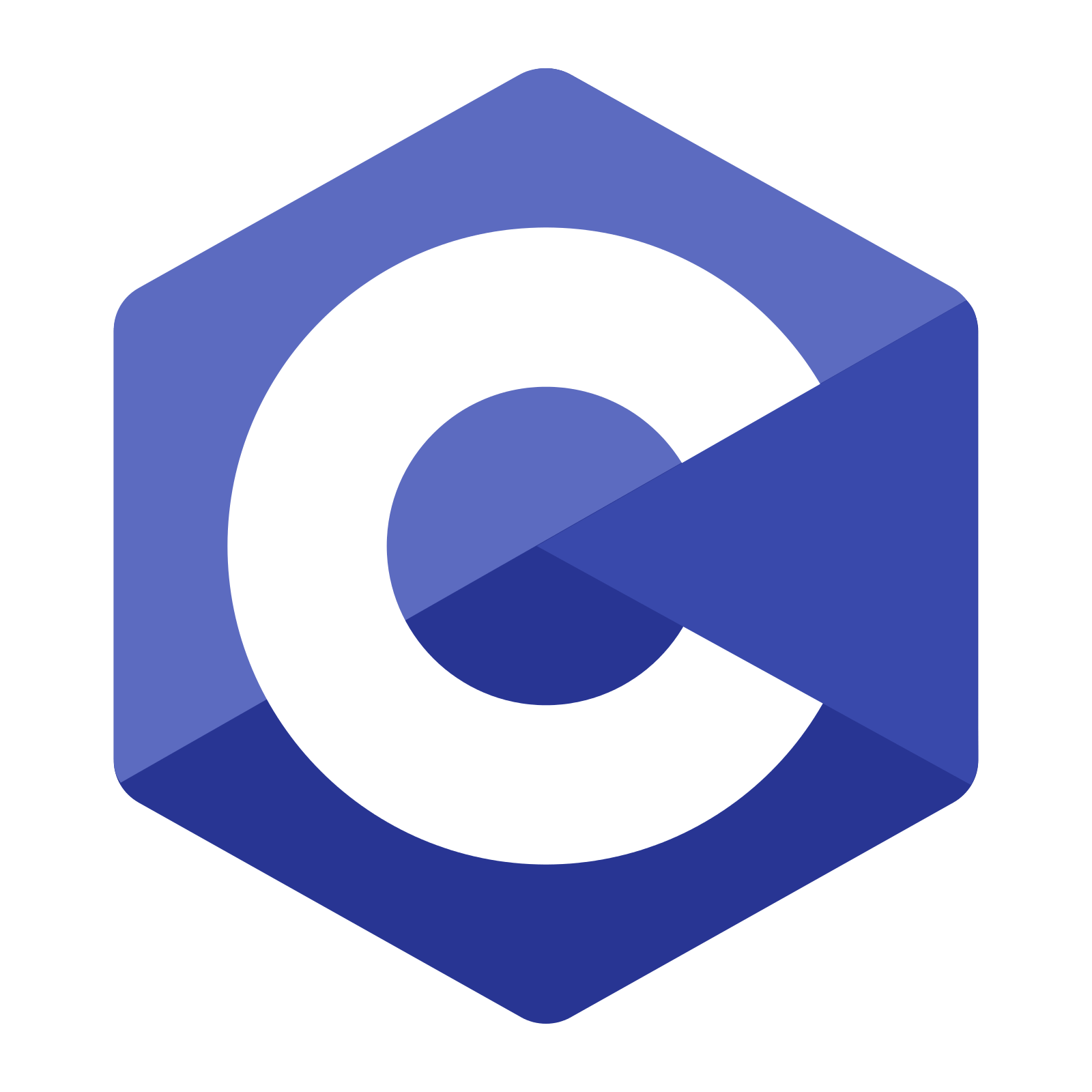
C Programming
File Handling in C Programming Language with Practical Examples
- Opening a FIle in C Programming
- File Accessing Modes
- Reading from a File
- Writing into a File
- Appending Data into a file
- Handling Binary File
- More Examples
In C programming, file handling enables us to retrieve, manipulate, and store data from and into files. Up to this point in the C programming tutorials, we have manually inputted data into our program through the terminal. Now, we will learn how to read, write, update, and store data permanently into a file, allowing us to access and utilize the information in the future.
¶Opening a FIle in C Programming
To manipulate file data in C programming, we need to open it first. In C, we use the fopen()
function to open a file and load it into memory for further operations. To close the file we use fclose()
for releasing the associated resources and removing it from memory.
Syntax of fopen()
:
fopen("file_name", "access_mode")
¶fopen()
Parameters
- file_name : A string containing the name of the file you want to open, create or update.
- access_mode : A string specifying the file access mode, indicating whether you intend to read, write, or perform other operations. If the file is not in the same directory as the program, the full path to the file must be inserted.
¶fopen()
Return Type
Returns a pointer to the beginning of the file. If the file cannot be opened for any reason, returns a NULL pointer.
#include<stdio.h>
#include<string>
int main()
{
FILE *file_ptr; // Pointer to store a file pointer
file_ptr = fopen("input.txt", "r"); // openning input.txt file with access mode 'r'
if (file_ptr == NULL) printf("File could not be opened!\n"); // returned null ptr
else printf("File has been opened successfully!\n"); // returned Valid pointer
fclose(file_ptr); // close the file
return 0;
}
File has been opened successfully!
¶File Accessing Modes
File access modes determine how a file is opened and what actions can be done with it. By specifying the access mode, we instruct our program on how to interact with the file and define the types of operations that can be performed on it. There are mainly three types of accessing modes in C programming:
-
"r"
- Read Mode: Allows reading from a file. -
"w"
- Write Mode: Allows writing into a file. -
"a"
- Append Mode: Enables appending data into a file.
¶Reading from a File
We need to open the file with access mode 'r'
to read file data. Then we can use various functions to read from the file, including fscanf()
and fgets()
. They work similarly, but we need to specify a file pointer.fscanf()
is used to read formatted data, while fgets()
is used to read a whole line from a file.
¶Reading from a File Using fscanf()
.
#include<stdio.h>
#include<string>
int main()
{
FILE *file_ptr; // Pointer to store a file pointer
file_ptr = fopen("input.txt", "r"); // openning input.txt file with access mode 'r'
if (file_ptr == NULL)
{
printf("File could not be opened!\n"); // returned null ptr
return 0;
}
char line[1000];
while (fscanf(file_ptr, "%s", line) != EOF) // reading word by word till the end.
{
printf("%s ", line);
}
fclose(file_ptr); // close the file
return 0;
}
C programming tutorial on file handling! This is another line!
¶Reading from a File Using fgets()
.
#include<stdio.h>
#include<string>
int main()
{
FILE *file_ptr; // Pointer to store a file pointer
file_ptr = fopen("input.txt", "r"); // openning input.txt file with access mode 'r'
if (file_ptr == NULL)
{
printf("File could not be opened!\n"); // returned null ptr
return 0;
}
char line[1000];
while (fgets(line, 1000, file_ptr) != NULL) // reading line by line
{
printf("%s", line); // printing each line
}
fclose(file_ptr); // close the file
return 0;
}
C programming tutorial on file handling!
This is another line!
¶Writing into a File
To write into a file, we need to open the file with access mode 'w'
. Then, various functions can be used for writing, including fprintf()
and fputs()
. Although they work similarly, we need to specify a file pointer. fprintf()
is used to write formatted data, while fputs()
is used to write a whole line into a file. If the file we are trying to write into does not exist, a new file is created with the specified file name.
¶Writing into a file using fprintf()
FILE *file_ptr; // Pointer to store a file pointer
file_ptr = fopen("input.txt", "w"); // openning input.txt file with access mode 'w'
if (file_ptr == NULL)
{
printf("File could not be opened!\n"); // returned null ptr
return 0;
}
char new_line[] = "We have entered this line!";
fprintf(file_ptr, "%s\n", new_line); // writing into the file
fclose(file_ptr); // closing the file
¶Writing into a file using fputs()
FILE *file_ptr; // Pointer to store a file pointer
file_ptr = fopen("input.txt", "w"); // openning input.txt file with access mode 'w'
if (file_ptr == NULL)
{
printf("File could not be opened!\n"); // returned null ptr
return 0;
}
char new_line[] = "We have entered this line!";
fputs(new_line, file_ptr); // writing into the file
fclose(file_ptr); // closing the file
¶Appending Data into a file
To add new information at the end of an existing file without overwriting its current content, we use access mode "a"
in the fopen()
function.
FILE *file_ptr; // Pointer to store a file pointer
file_ptr = fopen("input.txt", "a"); // openning input.txt file with access mode 'a'
if (file_ptr == NULL)
{
printf("File could not be opened!\n"); // returned null ptr
return 0;
}
char new_line[] = "Add this line at the end of the file!";
fputs(new_line, file_ptr); // writing into the file
fclose(file_ptr); // closing the file
¶Handling Binary File
So far in this article, we have worked with text files, learning how to read, write, and manipulate them in detail. When handling binary files, the process is similar, but we need to adjust the access modes while opening the file. To denote operations on binary files, it’s a common practice to add 'b'
after the access modes. However, it’s important to note that on many systems, particularly Unix-based ones, the 'b'
modifier is ignored as there is no distinction between text and binary files in those environments.
¶Binary File Access Modes
When working with binary files in C, the 'b'
is added to the access modes.
-
"rb"
: Read mode for binary files. -
"wb"
: Write mode for binary files -
"ab"
: Append mode for binary files
¶More Examples
Structure in C Programming Language with practical examples
Pointer in C Programming Language with Practical Examples
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
14
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development