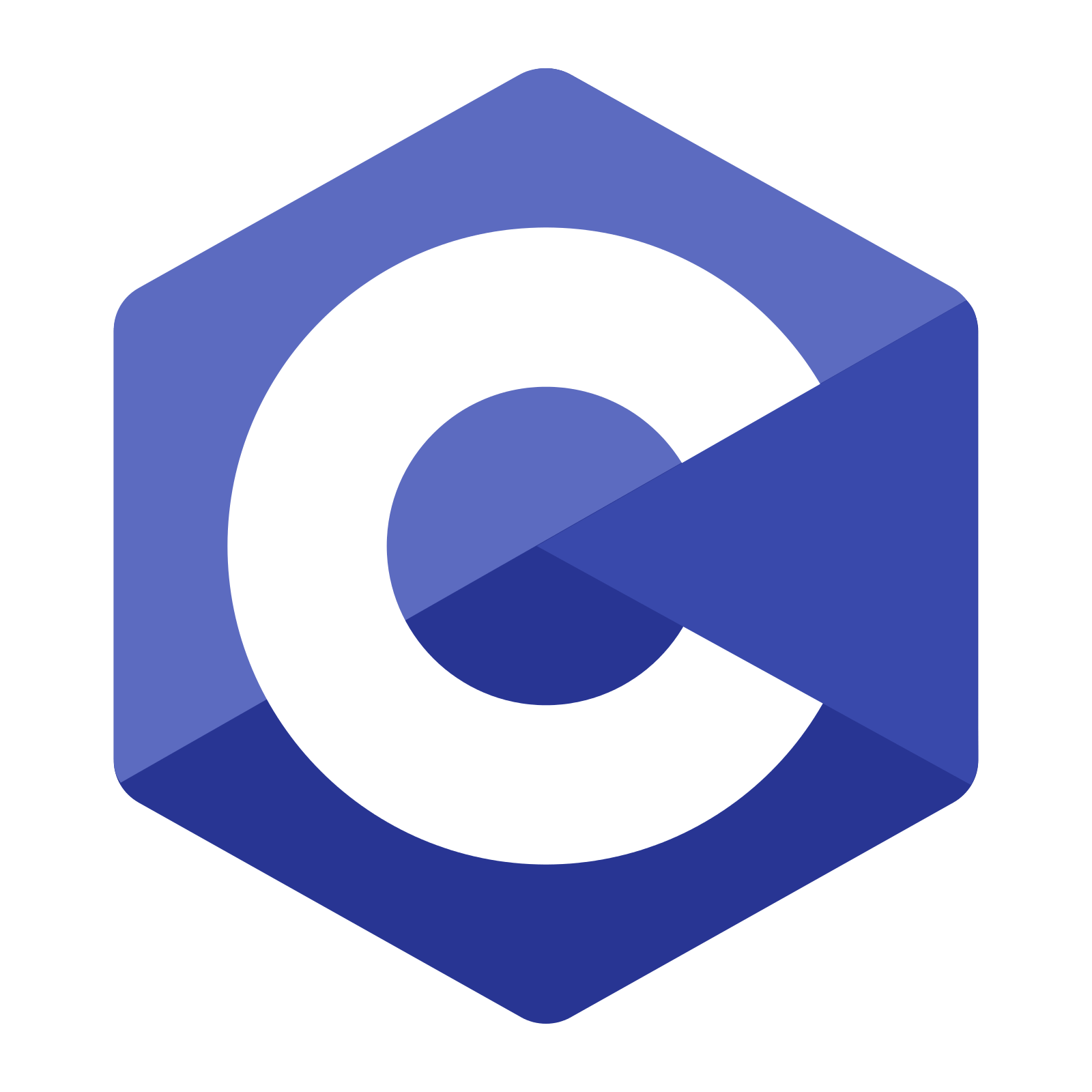
C Programming
Pointer in C Programming Language with Practical Examples
- Conceptual View of a Variable in Memory
- Declaration of Pointer
- Initialization of Pointer
- Dereferencing Pointer
- Types of Pointers in C Programming
- Pointer Arithmetic
- More Examples
In C programming, a Pointer variable stores memory address of another variable, pointing to where other variable resides in memory. Pointer variables Provide direct access and manipulation of memory, enhancing code flexibility and efficiency.
¶Conceptual View of a Variable in Memory
Every variable is assigned a specific memory location, allowing the program to store and retrieve data. This memory can be conceptualized as a contiguous sequence of bytes, each with a unique address. Let’s see how an variable is stored inside memory.

Here, an integer variable var
stores 13
at memory location 1001
and pointer variable ptr
stores the memory location 1001
of var
.
Though different data types require different amounts of memory to store a variable, all pointer variables require the same amount of memory for the memory address. The size of a memory address is architecture-dependent, with 64-bit
architectures requiring 8 bytes
, and 32-bit
architectures requiring 4 bytes
.
¶Declaration of Pointer
The declaration of a pointer involves specifying the data type it points to, followed by an asterisk (*) known as dereference operator. This asterisk indicates that the variable is a pointer.
data_type *var;
¶Initialization of Pointer
Pointers can be initialized by assigning the memory address of a variable to them. The address-of operator (&) is used for this purpose.
int var = 15;
int *ptr = &var;
Here, when we assign &var
to a pointer variable ptr
it stores the memory address (1001
) of the variable. Now, we can indirectly manipulate data in the address 1001
through ptr
.
¶Dereferencing Pointer
Dereferencing a pointer is accomplished using the dereference operator *. This operator, when applied to a pointer, retrieves the value stored at the memory address pointed to by that pointer.
#include <stdio.h>
int main() {
int var = 13;
int *ptr; // declaring pointer variable
ptr = &var; // assigning pointer variable
printf("Value at memory location %p = %d\n",ptr, *ptr); // retrieving data from memory location pointed by ptr
return 0;
}
Value at memory location 0x7fffffffd99c = 13
When we print value in ptr
it prints a memory location in hexadecimal as it stores a memory location. But when we dereference it with *
it prints the value in the memory location stored in it.
¶Types of Pointers in C Programming
Depending on the types of data that pointer variables point to, they are mainly classified as follows,
¶Null Pointers
A null pointer is a pointer that does not point to any memory location. It is often used to indicate the absence of a valid memory address. In C, a null pointer is represented by assigning the value NULL to a pointer.
int *ptr = NULL; // ptr is a null pointer
¶Void Pointers
Void pointers, or generic pointers, are pointers that can point to objects of any data type. They can be casted to different types according to need. Thus, providing a way to achieve flexibility in handling different data types without explicitly specifying the type. But they can not be dereferenced.
#include <stdio.h>
int main() {
float var = 13.0;
void *ptr; // declaring pointer variable
ptr = &var; // assigning pointer variable
float *float_ptr = (float *)ptr; // type casting void pointer
printf("Memory location %p\n", ptr); // retrieving data from memory location pointed by ptr
printf("Value at Memory location %p = %.5f\n", float_ptr, *float_ptr); // retrieving data from memory location pointed by ptr
return 0;
}
Memory location 0x7fffffffd994
Value at Memory location 0x7fffffffd994 = 13.00000
¶Regular Pointers
They are the pointers that point to specific data types like int, char, or float. These pointers are essential for efficiently manipulating and accessing individual elements of basic data types. Examples we have seen so far are mostly of this type.
int var = 13;
int *ptr; // declaring pointer variable
ptr = &var; // assigning pointer variable
¶Function Pointers
Function pointers point to functions instead of data. They are particularly useful in scenarios where different functions need to be called based on certain conditions. They actually point to the code segment in the memory of the function it is pointing to, allowing the execution of the function through the pointer.
#include <stdio.h>
int add(int a, int b) {
return a + b;
}
int main() {
int (*fnc) (int, int);
fnc = add;
int res = fnc(5, 7);
printf("sum = %d\n", res);
return 0;
}
sum = 12
¶Pointer to Pointer
A pointer to a pointer, also known as a multilevel pointer, stores the address of another pointer. This type of pointer is especially useful in dynamic memory allocation and multidimensional arrays.
#include <stdio.h>
int main() {
int var = 13;
int *ptr1 = &var;
int **ptr2 = &ptr1;
printf("Memory address stored in ptr2 = %p\nMemory address stored in ptr1 = %p\nValue stored in var = %d\n",
ptr2, *ptr2, **ptr2);
return 0;
}
Memory address stored in ptr2 = 0x7fffffffd998
Memory address stored in ptr1 = 0x7fffffffd994
Value stored in var = 13
¶Pointer Arithmetic
Pointer arithmetic is different from other variables. Pointer arithmetic supports following operations,
¶Addition and Subtraction
Adding or subtracting an integer value to a pointer results in moving in memory by a distance calculated based on the size of the data type the pointer points to. For integers, pointers move 4 bytes.
#include <stdio.h>
int main() {
int arr[5] = {10, 20, 30, 40, 50};
int *ptr = arr;
long long x = (long long)ptr, y;
++ptr;
y = (long long)ptr;
printf("Number of bytes added = %lld", y - x);
return 0;
}
Number of bytes added = 4
¶Comparison of Pointers of Same Types
We cam compare two pointers of the same type using relational operators. When comparing pointers, you are comparing the memory addresses they hold.
int arr[5] = {1, 2, 3, 4, 5};
int *p1 = &arr[2];
int *p2 = &arr[2];
if (p1 == p2) {
printf("Pointers are equal.\n");
} else {
printf("Pointers are not equal.\n");
}
¶More Examples
File Handling in C Programming Language with Practical Examples
Student Management Complete Application Using C Programming
All Tutorials in this playlist
Popular Tutorials
Categories
-
Artificial Intelligence (AI)
11
-
Bash Scripting
1
-
Bootstrap CSS
0
-
C Programming
14
-
C#
0
-
ChatGPT
1
-
Code Editor
2
-
Computer Engineering
3
-
CSS
28
-
Data Structure and Algorithm
13
-
Design Pattern in PHP
2
-
Design Patterns - Clean Code
1
-
E-Book
1
-
Git Commands
1
-
HTML
19
-
Interview Prepration
2
-
Java Programming
0
-
JavaScript
12
-
Laravel PHP Framework
37
-
Mysql
1
-
Node JS
1
-
Online Business
0
-
PHP
28
-
Programming
8
-
Python
12
-
React Js
19
-
React Native
1
-
Redux
2
-
Rust Programming
15
-
Tailwind CSS
1
-
Typescript
10
-
Uncategorized
0
-
Vue JS
1
-
Windows Operating system
1
-
Woocommerce
1
-
WordPress Development
2
Tags
- Artificial Intelligence (AI)
- Bash Scripting
- Business
- C
- C Programming
- C-sharp programming
- C++
- Code Editor
- Computer Engineering
- CSS
- Data Structure and Algorithm
- Database
- Design pattern
- Express JS
- git
- Git Commands
- github
- HTML
- Java
- JavaScript
- Laravel
- Mathematics
- MongoDB
- Mysql
- Node JS
- PHP
- Programming
- Python
- React Js
- Redux
- Rust Programming Language
- TypeScript
- Vue JS
- Windows terminal
- Woocommerce
- WordPress
- WordPress Plugin Development