Algorithm
Problem Nmae: 104. Maximum Depth of Binary Tree
Given the root
of a binary tree, return its maximum depth.
A binary tree's maximum depth is the number of nodes along the longest path from the root node down to the farthest leaf node.
Example 1:
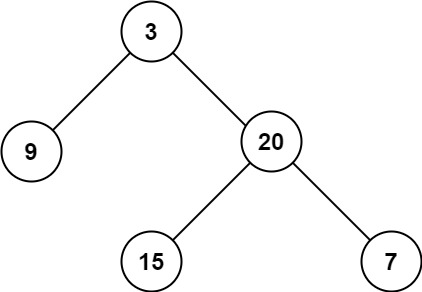
Input: root = [3,9,20,null,null,15,7] Output: 3
Example 2:
Input: root = [1,null,2] Output: 2
Constraints:
- The number of nodes in the tree is in the range
[0, 104]
. -100 <= Node.val <= 100
Code Examples
#1 Code Example with C Programming
Code -
C Programming
int maxDepth(struct TreeNode* root) {
int l, r;
if (!root) return 0;
l = maxDepth(root->left) + 1;
r = maxDepth(root->right) + 1;
return l > r ? l : r;
}
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with C++ Programming
Code -
C++ Programming
class Solution {
public:
int maxDepth(TreeNode* root) {
if (!root) return 0;
return max(maxDepth(root->left), maxDepth(root->right)) + 1;
}
};
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Java Programming
Code -
Java Programming
class Solution {
public int maxDepth(TreeNode root) {
if (root == null) {
return 0;
}
return 1 + Math.max(maxDepth(root.left), maxDepth(root.right));
}
}
Copy The Code &
Try With Live Editor
Input
Output
#4 Code Example with Javascript Programming
Code -
Javascript Programming
const maxDepth = function(root) {
if (!root) return 0;
const left = maxDepth(root.left);
const right = maxDepth(root.right);
let depth = left > right ? left : right;
return (depth += 1);
};
Copy The Code &
Try With Live Editor
Input
Output
#5 Code Example with Python Programming
Code -
Python Programming
class Solution:
def maxDepth(self, root: TreeNode, d = 0) -> int:
return max(self.maxDepth(root.left, d + 1), self.maxDepth(root.right, d + 1)) if root else d
Copy The Code &
Try With Live Editor
Input
Output
#6 Code Example with C# Programming
Code -
C# Programming
using System;
namespace LeetCode
{
public class _104_MaximumDepthOfBinaryTree
{
public int MaxDepth(TreeNode root)
{
if (root == null) return 0;
return Math.Max(1 + MaxDepth(root.left), 1 + MaxDepth(root.right));
}
}
}
Copy The Code &
Try With Live Editor
Input
Output