Algorithm
Problem Name: 941. Valid Mountain Array
Given an array of integers arr
, return true
if and only if it is a valid mountain array.
Recall that arr is a mountain array if and only if:
arr.length >= 3
- There exists some
i
with0 < i < arr.length - 1
such that:arr[0] < arr[1] < ... < arr[i - 1] < arr[i]
arr[i] > arr[i + 1] > ... > arr[arr.length - 1]
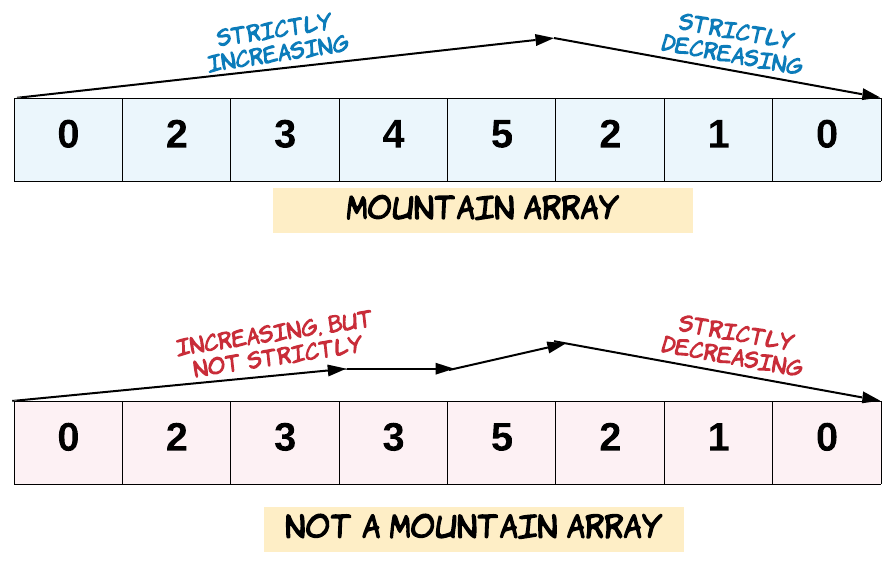
Example 1:
Input: arr = [2,1] Output: false
Example 2:
Input: arr = [3,5,5] Output: false
Example 3:
Input: arr = [0,3,2,1] Output: true
Constraints:
1 <= arr.length <= 104
0 <= arr[i] <= 104
Code Examples
#1 Code Example with C++ Programming
Code -
C++ Programming
class Solution {
public:
bool validMountainArray(vector<int>& A) {
int i = 0, n = A.size();
if (n < 3) {
return false;
}
while (i < n - 1 && A[i] < A[i + 1]) {
++i;
}
if (i == 0 || i == n - 1) {
return false;
}
while (i < n - 1 && A[i] > A[i + 1]) {
++i;
}
return i == n - 1;
}
};
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Java Programming
Code -
Java Programming
class Solution {
public boolean validMountainArray(int[] A) {
if (A.length < 3) {
return false;
}
int idx = 0;
int n = A.length;
while (idx + 1 < n && A[idx] < A[idx + 1]) {
idx++;
}
if (idx == 0 || idx == n - 1) {
return false;
}
while (idx + 1 < n && A[idx] > A[idx + 1]) {
idx++;
}
return idx == n - 1;
}
}
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Javascript Programming
Code -
Javascript Programming
const validMountainArray = function(A) {
if (A.length < 3) return false;
let start = 0;
let end = A.length - 1;
while (start < end) {
while (A[end - 1] > A[end]) {
end--;
}
while (A[start] < A[start + 1]) {
start++;
}
if (start !== end || start === 0 || end === A.length - 1) return false;
}
return true;
};
Copy The Code &
Try With Live Editor
Input
Output
#4 Code Example with Python Programming
Code -
Python Programming
class Solution:
def validMountainArray(self, A):
i = A and A.index(max(A))
return A and 0a3 for a2,a3 in zip(A[i:],A[i+1:])) or False
Copy The Code &
Try With Live Editor
Input
Output
#5 Code Example with C# Programming
Code -
C# Programming
namespace LeetCode
{
public class _0941_ValidMountainArray
{
public bool ValidMountainArray(int[] A)
{
int i = 0, N = A.Length;
while (i + 1 < N && A[i] < A[i + 1])
i++;
if (i == 0 || i == N - 1) return false;
while (i + 1 < N && A[i] > A[i + 1])
i++;
return i == N - 1;
}
}
}
Copy The Code &
Try With Live Editor
Input
Output