Algorithm
Problem Nmae: 114. Flatten Binary Tree to Linked List
Given the root
of a binary tree, flatten the tree into a "linked list":
- The "linked list" should use the same
TreeNode
class where theright
child pointer points to the next node in the list and theleft
child pointer is alwaysnull
. - The "linked list" should be in the same order as a pre-order traversal of the binary tree.
Example 1:
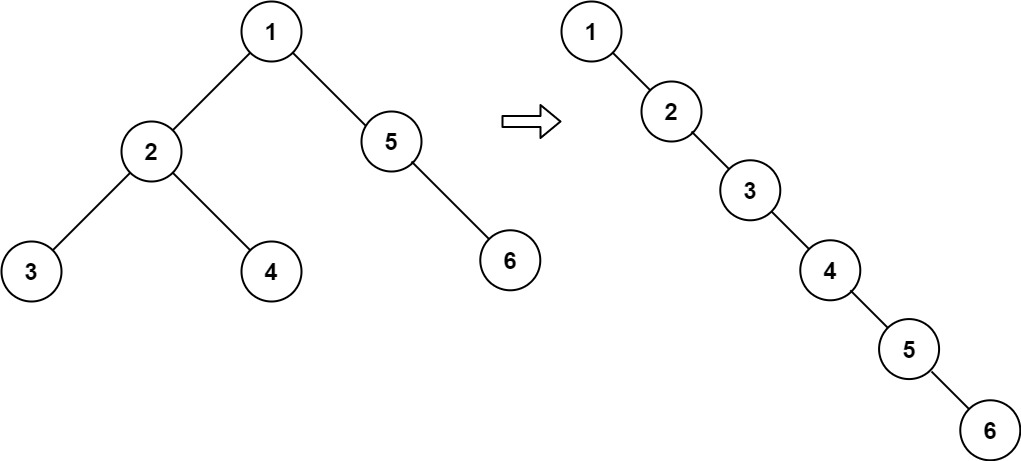
Input: root = [1,2,5,3,4,null,6] Output: [1,null,2,null,3,null,4,null,5,null,6]
Example 2:
Input: root = [] Output: []
Example 3:
Input: root = [0] Output: [0]
Constraints:
- The number of nodes in the tree is in the range
[0, 2000]
. -100 <= Node.val <= 100
Code Examples
#1 Code Example with C Programming
Code -
C Programming
struct TreeNode *preOrderTraversal(struct TreeNode *prev, struct TreeNode *node) {
struct TreeNode *left, *right;
if (!node) return prev;
left = node->left;
right = node->right;
node->left = node->right = NULL;
prev->right = node;
prev = node;
prev = preOrderTraversal(prev, left); // it's import to update prev
prev = preOrderTraversal(prev, right);
return prev;
}
void flatten(struct TreeNode* root) {
struct TreeNode head = { 0 };
preOrderTraversal(&head, root);
}
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with C++ Programming
Code -
C++ Programming
// Recursive
class Solution {
public:
void flatten(TreeNode* root) {
TreeNode* l, *r;
DFS(root, l, r);
}
TreeNode* DFS(TreeNode* root, TreeNode* l, TreeNode* r){
if(!root) return NULL;
l = DFS(root->left, l, r);
if(l){
l->right = root->right;
root->right = root->left;
root->left = NULL;
}
r = DFS(root->right, l, r);
return r ? r : l ? l : root;
}
};
// Iterative
class Solution {
public:
void flatten(TreeNode* root) {
TreeNode* p;
while(root){
if(root->left && root->right){
p = root->left;
while(p->right) p = p->right;
p->right = root->right;
}
if(root->left) root->right = root->left;
root->left = NULL;
root = root->right;
}
}
};
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Java Programming
Code -
Java Programming
class Solution {
public void flatten(TreeNode root) {
if (root == null) {
return;
}
Stack < TreeNode> stack = new Stack<>();
stack.push(root);
while (!stack.isEmpty()) {
TreeNode curr = stack.pop();
if (curr.right != null) {
stack.push(curr.right);
}
if (curr.left != null) {
stack.push(curr.left);
}
if (!stack.isEmpty()) {
curr.right = stack.peek();
}
curr.left = null;
}
}
}
Copy The Code &
Try With Live Editor
Input
Output
#4 Code Example with Javascript Programming
Code -
Javascript Programming
const flatten = function(root) {
let prev = null
function op(root) {
if (root == null) return;
op(root.right);
op(root.left);
root.right = prev;
root.left = null;
prev = root;
}
op(root)
};
Copy The Code &
Try With Live Editor
Input
Output
#5 Code Example with Python Programming
Code -
Python Programming
class Solution:
def flatten(self, root):
"""
:type root: TreeNode
:rtype: void Do not return anything, modify root in-place instead.
"""
def traverse(node):
if not node: return
left, right = traverse(node.left), traverse(node.right)
if node.left: left.right, node.right, node.left = node.right, node.left, None
return right if right else left if left else node
traverse(root)
Copy The Code &
Try With Live Editor
Input
Output
#6 Code Example with C# Programming
Code -
C# Programming
namespace LeetCode
{
public class _114_FlattenBinaryTreeToLinkedList
{
TreeNode prev = null;
public void Flatten(TreeNode root)
{
if (root == null) return;
Flatten(root.right);
Flatten(root.left);
root.right = prev;
root.left = null;
prev = root;
}
}
}
Copy The Code &
Try With Live Editor
Input
Output