Algorithm
Problem Name: 500. Keyboard Row
Given an array of strings words
, return the words that can be typed using letters of the alphabet on only one row of American keyboard like the image below.
In the American keyboard:
- the first row consists of the characters
"qwertyuiop"
, - the second row consists of the characters
"asdfghjkl"
, and - the third row consists of the characters
"zxcvbnm"
.
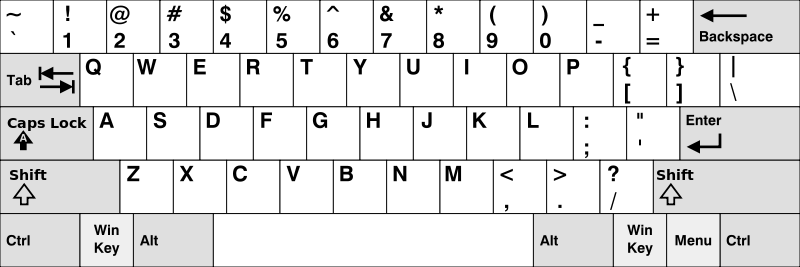
Example 1:
Input: words = ["Hello","Alaska","Dad","Peace"] Output: ["Alaska","Dad"]
Example 2:
Input: words = ["omk"] Output: []
Example 3:
Input: words = ["adsdf","sfd"] Output: ["adsdf","sfd"]
Constraints:
1 <= words.length <= 20
1 <= words[i].length <= 100
words[i]
consists of English letters (both lowercase and uppercase).
Code Examples
#1 Code Example with Java Programming
Code -
Java Programming
class Solution {
public String[] findWords(String[] words) {
Map map = new HashMap<>();
update(map, "qwertyuiop", 1);
update(map, "asdfghjkl", 2);
update(map, "zxcvbnm", 3);
List < Integer> list = new ArrayList<>();
for (int i = 0; i < words.length; i++) {
int rowIdx = -1;
for (char c : words[i].toCharArray()) {
if (rowIdx == -1) {
rowIdx = map.get(Character.toLowerCase(c));
}
if (map.get(Character.toLowerCase(c)) != rowIdx) {
rowIdx = -1;
break;
}
}
if (rowIdx != -1) {
list.add(i);
}
}
String[] ans = new String[list.size()];
for (int i = 0; i < list.size(); i++) {
ans[i] = words[list.get(i)];
}
return ans;
}
private void update(Map < Character, Integer> map, String s, int row) {
for (char c : s.toCharArray()) {
map.put(c, row);
}
}
}
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Javascript Programming
Code -
Javascript Programming
const findWords = function(words) {
const regex = /^[qwertyuiop]*$|^[asdfghjkl]*$|^[zxcvbnm]*$/;
return words.filter(
s => (s.toLowerCase().match(regex) === null ? false : true)
);
};
console.log(findWords(["Hello", "Alaska", "Dad", "Peace"]));
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Python Programming
Code -
Python Programming
class Solution:
def findWords(self, words):
return [w for w in words if any(not set(w.lower()) - row for row in (set("qwertyuiop"), set("asdfghjkl"), set("zxcvbnm")))]
Copy The Code &
Try With Live Editor
Input
Output
#4 Code Example with C# Programming
Code -
C# Programming
using System.Collections.Generic;
namespace LeetCode
{
public class _0500_KeyboardRow
{
public string[] FindWords(string[] words)
{
if (words.Length == 0) return words;
var map = new Dictionary < char, int>();
string r1 = "qwertyuiopQWERTYUIOP";
foreach (var ch in r1)
map[ch] = 1;
string r2 = "asdfghjklASDFGHJKL";
foreach (var ch in r2)
map[ch] = 2;
string r3 = "zxcvbnmZXCVBNM";
foreach (var ch in r3)
map[ch] = 3;
var result = new List < string>();
foreach (var word in words)
{
var flag = map[word[0]];
var valid = true;
foreach (var ch in word)
{
if (flag != map[ch])
{
valid = false;
break;
}
}
if (valid)
result.Add(word);
}
return result.ToArray();
}
}
}
Copy The Code &
Try With Live Editor
Input
Output