Algorithm
Problem Name: 661. Image Smoother
An image smoother is a filter of the size 3 x 3
that can be applied to each cell of an image by rounding down the average of the cell and the eight surrounding cells (i.e., the average of the nine cells in the blue smoother). If one or more of the surrounding cells of a cell is not present, we do not consider it in the average (i.e., the average of the four cells in the red smoother).
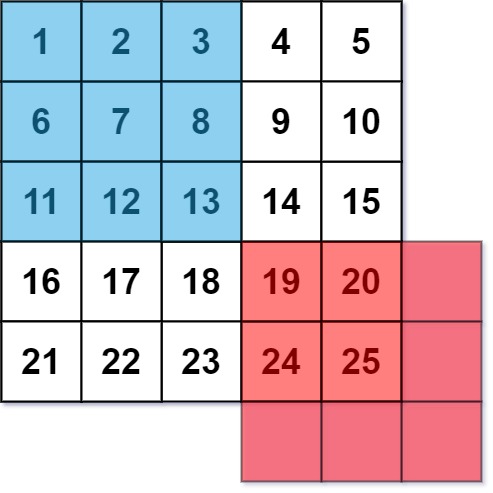
Given an m x n
integer matrix img
representing the grayscale of an image, return the image after applying the smoother on each cell of it.
Example 1:
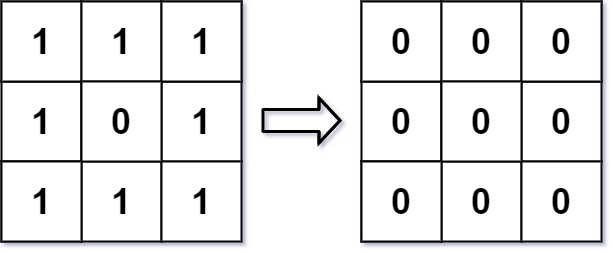
Input: img = [[1,1,1],[1,0,1],[1,1,1]] Output: [[0,0,0],[0,0,0],[0,0,0]] Explanation: For the points (0,0), (0,2), (2,0), (2,2): floor(3/4) = floor(0.75) = 0 For the points (0,1), (1,0), (1,2), (2,1): floor(5/6) = floor(0.83333333) = 0 For the point (1,1): floor(8/9) = floor(0.88888889) = 0
Example 2:
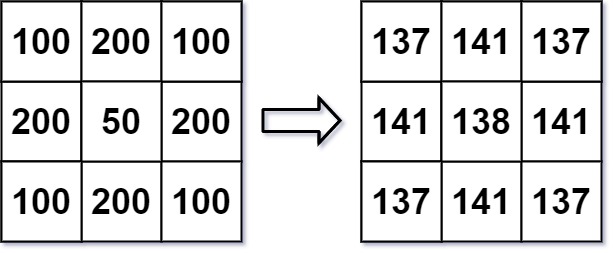
Input: img = [[100,200,100],[200,50,200],[100,200,100]] Output: [[137,141,137],[141,138,141],[137,141,137]] Explanation: For the points (0,0), (0,2), (2,0), (2,2): floor((100+200+200+50)/4) = floor(137.5) = 137 For the points (0,1), (1,0), (1,2), (2,1): floor((200+200+50+200+100+100)/6) = floor(141.666667) = 141 For the point (1,1): floor((50+200+200+200+200+100+100+100+100)/9) = floor(138.888889) = 138
Constraints:
m == img.length
n == img[i].length
1 <= m, n <= 200
0 <= img[i][j] <= 255
Code Examples
#1 Code Example with Java Programming
Code -
Java Programming
class Solution {
public int[][] imageSmoother(int[][] M) {
int[][] res = new int[M.length][M[0].length];
for (int i=0;i < M.length;i++) {
for (int j=0;j < M[0].length;j++) {
int count = 1;
int sum = M[i][j];
if(j-1 >= 0) {
count++;
sum += M[i][j-1];
}
if(j+1 < M[0].length> {
count++;
sum += M[i][j+1];
}
if(i-1 >= 0) {
count++;
sum += M[i-1][j];
}
if(i+1 < M.length) {
count++;
sum += M[i+1][j];
}
if(i+1 < M.length && j+1 < M[0].length> {
count++;
sum += M[i+1][j+1];
}
if(i+1 < M.length && j-1 >= 0) {
count++;
sum += M[i+1][j-1];
}
if(i-1 >= 0 && j-1 >= 0) {
count++;
sum += M[i-1][j-1];
}
if(i-1 >= 0 && j+1 < M[0].length) {
count++;
sum += M[i-1][j+1];
}
res[i][j] = (int)Math.floor(sum/count>;
}
}
return res;
}
}
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Javascript Programming
Code -
Javascript Programming
const imageSmoother = function (M) {
const r = M.length
if (r === 0) return 0
const c = M[0].length
if (c === 0) return 0
const res = Array.from({ length: r }, () => Array(c).fill(0))
for (let i = 0; i < r; i++) {
for (let j = 0; j < c; j++) {
res[i][j] = helper(M, i, j, res)
}
}
return res
}
function helper(M, i, j, res) {
let val = M[i][j]
let num = 1
const dirs = [
[-1, -1],
[-1, 0],
[-1, 1],
[0, -1],
[0, 1],
[1, -1],
[1, 0],
[1, 1],
]
for (let [dr, dc] of dirs) {
const ii = i + dr
const jj = j + dc
if (M[ii] != null && M[ii][jj] != null) {
val += M[ii][jj]
num++
}
}
return (val / num) >> 0
}
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Python Programming
Code -
Python Programming
class Solution:
def imageSmoother(self, M: List[List[int]]) -> List[List[int]]:
m, n = len(M), len(M[0])
grid = [[0] * n for _ in range(m)]
for i in range(m):
for j in range(n):
adj = [M[i + x][j + y] for x, y in ((0, 0), (-1, 0), (1, 0), (0, -1), (0, 1), (-1, -1), (-1, 1), (1, 1), (1, -1)) if 0 <= i + x < m and 0 <= j + y < n]
grid[i][j] = sum(adj) // len(adj)
return grid
Copy The Code &
Try With Live Editor
Input
#4 Code Example with C# Programming
Code -
C# Programming
namespace LeetCode
{
public class _0661_ImageSmoother
{
public int[][] ImageSmoother(int[][] M)
{
var rows = M.Length;
var cols = M[0].Length;
int[][] answer = new int[rows][];
for (var r = 0; r < rows; ++r)
{
answer[r] = new int[cols];
for (var c = 0; c < cols; ++c)
{
int count = 0;
for (var nr = r - 1; nr < = r + 1; nr++)
for (var nc = c - 1; nc < = c + 1; nc++)
{
if (0 <= nr && nr < rows && 0 <= nc && nc < cols)
{
answer[r][c] += M[nr][nc];
count++;
}
}
answer[r][c] /= count;
}
}
return answer;
}
}
}
Copy The Code &
Try With Live Editor
Input
Output