Algorithm
Problem Name: 671. Second Minimum Node In a Binary Tree
Given a non-empty special binary tree consisting of nodes with the non-negative value, where each node in this tree has exactly two
or zero
sub-node. If the node has two sub-nodes, then this node's value is the smaller value among its two sub-nodes. More formally, the property root.val = min(root.left.val, root.right.val)
always holds.
Given such a binary tree, you need to output the second minimum value in the set made of all the nodes' value in the whole tree.
If no such second minimum value exists, output -1 instead.
Example 1:
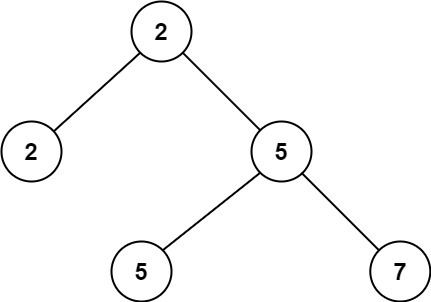
Input: root = [2,2,5,null,null,5,7] Output: 5 Explanation: The smallest value is 2, the second smallest value is 5.
Example 2:
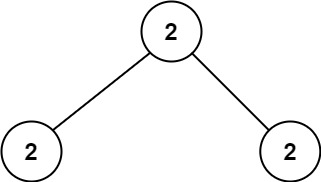
Input: root = [2,2,2] Output: -1 Explanation: The smallest value is 2, but there isn't any second smallest value.
Constraints:
- The number of nodes in the tree is in the range
[1, 25]
. 1 <= Node.val <= 231 - 1
root.val == min(root.left.val, root.right.val)
for each internal node of the tree.
Code Examples
#1 Code Example with C++ Programming
Code -
C++ Programming
class Solution {
public:
int findSecondMinimumValue(TreeNode* root) {
int res = INT_MAX;
DFS(root, root->val, res);
return res == INT_MAX ? -1 : res;
}
void DFS(TreeNode* root, int val, int& res){
if(!root) return;
if(root->val != val) res = min(res, root->val);
if(root->val == val) DFS(root->left, val, res), DFS(root->right, val, res);
}
};
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Java Programming
Code -
Java Programming
class Solution {
public int findSecondMinimumValue(TreeNode root) {
long[] topTwo = {root.val, Long.MAX_VALUE};
helper(root, topTwo);
return topTwo[1] == Long.MAX_VALUE ? -1 : (int) topTwo[1];
}
private void helper(TreeNode root, long[] topTwo) {
if (root == null) {
return;
}
if (topTwo[0] < root.val && root.val < topTwo[1]) {
topTwo[1] = root.val;
} else {
helper(root.left, topTwo);
helper(root.right, topTwo);
}
}
}
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Javascript Programming
Code -
Javascript Programming
const findSecondMinimumValue = function(root) {
if(root == null) return -1
const q = [root]
let min = Number.MAX_VALUE
let min2nd = Number.MAX_VALUE
while(q.length) {
const len = q.length
for(let i = 0; i < len; i++) {
const cur = q.shift()
if(cur.val < = min> {
min = cur.val
} else if(cur.val > min && cur.val < min2nd) {
min2nd = cur.val
}
if(cur.left) q.push(cur.left)
if(cur.right) q.push(cur.right>
}
}
return min2nd === Number.MAX_VALUE ? -1 : min2nd
};
Copy The Code &
Try With Live Editor
Input
Output
#4 Code Example with Python Programming
Code -
Python Programming
class Solution:
def findSecondMinimumValue(self, root: TreeNode) -> int:
self.sec = float('inf')
def dfs(node):
if not node: return
dfs(node.left)
dfs(node.right)
if root.val < node.val < self.sec:
self.sec = node.val
dfs(root)
return self.sec if self.sec < float('inf') else -1
Copy The Code &
Try With Live Editor
Input
Output
#5 Code Example with C# Programming
Code -
C# Programming
namespace LeetCode
{
public class _0671_SecondMinimumNodeInABinaryTree
{
private int min = int.MaxValue;
private long result = long.MaxValue;
public int FindSecondMinimumValue(TreeNode root)
{
min = root.val;
Traverse(root);
return (result == long.MaxValue) ? -1 : (int)result;
}
private void Traverse(TreeNode node)
{
if (node == null) return;
if (node.val > result) return;
if (min < node.val && result > node.val)
result = node.val;
else
{
Traverse(node.left);
Traverse(node.right);
}
}
}
}
Copy The Code &
Try With Live Editor
Input
Output