Algorithm
Problem Name: 328. Odd Even Linked List
Problem Link: https://leetcode.com/problems/odd-even-linked-list/
Given the head
of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return the reordered list.
The first node is considered odd, and the second node is even, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in O(1)
extra space complexity and O(n)
time complexity.
Example 1:
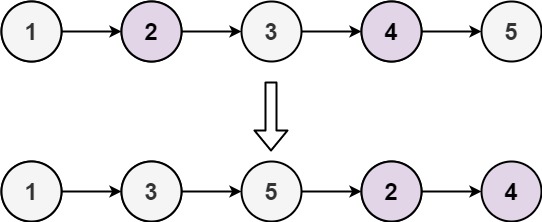
Input: head = [1,2,3,4,5] Output: [1,3,5,2,4]
Example 2:
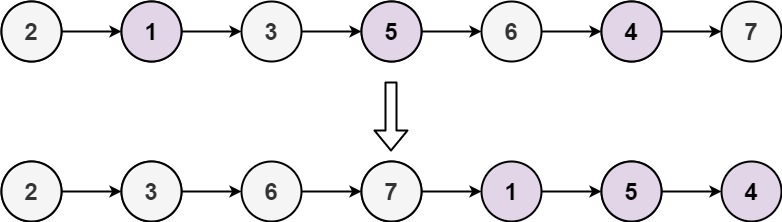
Input: head = [2,1,3,5,6,4,7] Output: [2,3,6,7,1,5,4]
Constraints:
- The number of nodes in the linked list is in the range
[0, 104]
. -106 <= Node.val <= 106
Code Examples
#1 Code Example with Java Programming
Code -
Java Programming
class Solution {
public ListNode oddEvenList(ListNode head) {
if (head == null || head.next == null) {
return head;
}
ListNode oddIdxNode = head;
ListNode evenIdxNodeStart = head.next;
ListNode evenIdxNode = evenIdxNodeStart;
while (evenIdxNode != null && evenIdxNode.next != null) {
oddIdxNode.next = evenIdxNode.next;
oddIdxNode = oddIdxNode.next;
evenIdxNode.next = oddIdxNode.next;
evenIdxNode = evenIdxNode.next;
}
oddIdxNode.next = evenIdxNodeStart;
return head;
}
}
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Javascript Programming
Code -
Javascript Programming
const oddEvenList = function(head) {
if (head === null) return null;
let odd = head,
even = head.next,
evenHead = even;
while (even !== null && even.next !== null) {
odd.next = even.next;
odd = odd.next;
even.next = odd.next;
even = even.next;
}
odd.next = evenHead;
return head;
};
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Python Programming
Code -
Python Programming
class Solution:
def oddEvenList(self, head):
"""
:type head: ListNode
:rtype: ListNode
"""
root, i, last, first = head, 1, None, None
if head and head.next: first = head.next
while head:
latter = head.next
if i%2 != 0: last = head
if head.next: head.next = head.next.next
head, i = latter, i+1
if last: last.next = first
return root
Copy The Code &
Try With Live Editor
Input
#4 Code Example with C# Programming
Code -
C# Programming
namespace LeetCode
{
public class _0328_OddEvenLinkedList
{
public ListNode OddEvenList(ListNode head)
{
if (head == null) return null;
ListNode odd = head, even = head.next, evenHead = even;
while (even != null && even.next != null)
{
odd.next = even.next;
odd = odd.next;
even.next = odd.next;
even = even.next;
}
odd.next = evenHead;
return head;
}
}
}
Copy The Code &
Try With Live Editor
Input
Output