Algorithm
Problem Name: 700. Search in a Binary Search Tree
You are given the root
of a binary search tree (BST) and an integer val
.
Find the node in the BST that the node's value equals val
and return the subtree rooted with that node. If such a node does not exist, return null
.
Example 1:
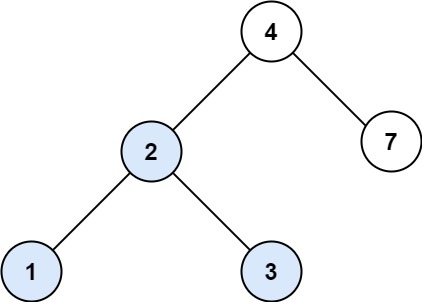
Input: root = [4,2,7,1,3], val = 2 Output: [2,1,3]
Example 2:
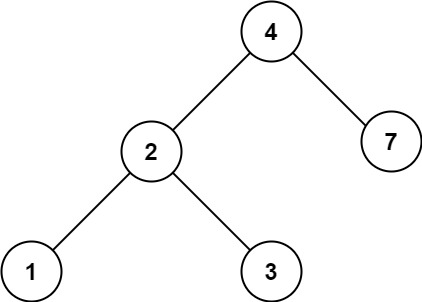
Input: root = [4,2,7,1,3], val = 5 Output: []
Constraints:
- The number of nodes in the tree is in the range
[1, 5000]
. 1 <= Node.val <= 107
root
is a binary search tree.1 <= val <= 107
Code Examples
#1 Code Example with Java Programming
Code -
Java Programming
class Solution {
public TreeNode searchBST(TreeNode root, int val) {
if (root == null) {
return root;
}
if (root.val == val) {
return root;
}
return root.val > val ? searchBST(root.left, val) : searchBST(root.right, val);
}
}
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Javascript Programming
Code -
Javascript Programming
const searchBST = function (root, val) {
if (!root || root.val === val) {
return root
}
return root.val < val ? searchBST(root.right, val) : searchBST(root.left, val)
}
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Python Programming
Code -
Python Programming
class Solution:
def searchBST(self, root, val):
if root and val < root.val: return self.searchBST(root.left, val)
elif root and val > root.val: return self.searchBST(root.right, val)
return root
Copy The Code &
Try With Live Editor
Input
Output
#4 Code Example with C# Programming
Code -
C# Programming
namespace LeetCode
{
public class _0700_SearchInABinarySearchTree
{
public TreeNode SearchBST(TreeNode root, int val)
{
if (root == null) return null;
if (root.val == val) return root;
else if (root.val < val) return SearchBST(root.right, val);
else return SearchBST(root.left, val);
}
}
}
Copy The Code &
Try With Live Editor
Input
Output