Algorithm
Problem Name: 203. Remove Linked List Elements
Given the head
of a linked list and an integer val
, remove all the nodes of the linked list that has Node.val == val
, and return the new head.
Example 1:
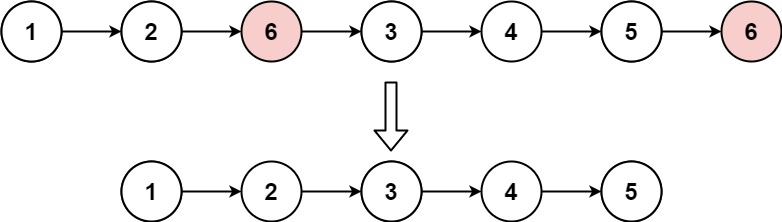
Input: head = [1,2,6,3,4,5,6], val = 6 Output: [1,2,3,4,5]
Example 2:
Input: head = [], val = 1 Output: []
Example 3:
Input: head = [7,7,7,7], val = 7 Output: []
Constraints:
- The number of nodes in the list is in the range
[0, 104]
. 1 <= Node.val <= 50
0 <= val <= 50
Code Examples
#1 Code Example with C Programming
Code -
C Programming
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* removeElements(struct ListNode* head, int val) {
struct ListNode *ret;
struct ListNode *prev, *p;
ret = p = head;
prev = head;
while (p) {
if (p->val == val) {
if (p == ret) { /* head */
ret = p->next;
prev = ret; /* maybe useless */
}
else { /* NOT head */
prev->next = p->next;
}
}
else {
prev = p;
}
p = p->next;
}
return ret;
}
int main() {
struct ListNode *l1 = (struct ListNode *)calloc(9, sizeof(struct ListNode));
struct ListNode *p = l1;
p->val = 6;
p->next = l1 + 1;
p = p->next;
p->val = 6;
p->next = l1 + 2;
p = p->next;
p->val = 1;
p->next = l1 + 3;
p = p->next;
p->val = 6;
p->next = l1 + 4;
p = p->next;
p->val = 6;
p->next = l1 + 5;
p = p->next;
p->val = 6;
p->next = l1 + 6;
p = p->next;
p->val = 2;
p->next = l1 + 7;
p = p->next;
p->val = 3;
p->next = l1 + 8;
p = p->next;
p->val = 6;
p->next = NULL;
/* 6 -> 6 -> 1 -> 6 -> 6 -> 6 -> 2 -> 3 -> 6 */
p = l1;
while (p) {
printf("%d ", p->val);
p = p->next;
}
printf("\n");
p = removeElements(l1, 6);
/* 1 -> 2 -> 3 */
while (p) {
printf("%d ", p->val);
p = p->next;
}
printf("\n");
return 0;
}
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Java Programming
Code -
Java Programming
class Solution {
public ListNode removeElements(ListNode head, int val) {
ListNode newHead = null;
ListNode curr = head;
while (curr != null && curr.val == val) {
curr = curr.next;
}
newHead = curr;
while (curr != null && curr.next != null) {
if (curr.next.val == val) {
curr.next = curr.next.next;
} else {
curr = curr.next;
}
}
return newHead;
}
}
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Javascript Programming
Code -
Javascript Programming
const removeElements = function(head, val) {
const dummy = new ListNode(Infinity)
if(head == null) return null
dummy.next = head
let cur = head
let prev = dummy
while(cur) {
if(cur.val === val) {
prev.next = cur.next
cur = cur.next
} else {
prev = cur
cur = cur.next
}
}
return dummy.next
};
Copy The Code &
Try With Live Editor
Input
Output
#5 Code Example with C# Programming
Code -
C# Programming
namespace LeetCode
{
public class _0203_RemoveLinkedListElements
{
public ListNode RemoveElements(ListNode head, int val)
{
var dummy = new ListNode(-1);
dummy.next = head;
ListNode prev = dummy, curr = head;
while (curr != null)
{
if (curr.val == val)
prev.next = curr.next;
else
prev = prev.next;
curr = curr.next;
}
return dummy.next;
}
}
}
Copy The Code &
Try With Live Editor
Input
Output