Algorithm
Problem Name: 766. Toeplitz Matrix
Given an m x n
matrix
, return true
if the matrix is Toeplitz. Otherwise, return false
.
A matrix is Toeplitz if every diagonal from top-left to bottom-right has the same elements.
Example 1:
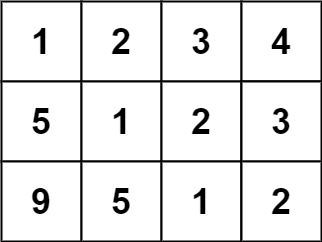
Input: matrix = [[1,2,3,4],[5,1,2,3],[9,5,1,2]] Output: true Explanation: In the above grid, the diagonals are: "[9]", "[5, 5]", "[1, 1, 1]", "[2, 2, 2]", "[3, 3]", "[4]". In each diagonal all elements are the same, so the answer is True.
Example 2:
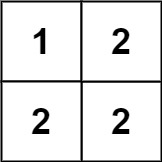
Input: matrix = [[1,2],[2,2]] Output: false Explanation: The diagonal "[1, 2]" has different elements.
Constraints:
m == matrix.length
n == matrix[i].length
1 <= m, n <= 20
0 <= matrix[i][j] <= 99
Code Examples
#1 Code Example with C++ Programming
Code -
C++ Programming
class Solution {
public:
bool isToeplitzMatrix(vector<vector<int>>& matrix) {
for(int i = 1; i < matrix.size(); i++)
for(int j = 1; j < matrix[0].size(); j++)
if(matrix[i][j] != matrix[i - 1][j - 1]) return false;
return true;
}
};
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Java Programming
Code -
Java Programming
class Solution {
public boolean isToeplitzMatrix(int[][] matrix) {
for (int i = 0; i < matrix.length - 1; i++) {
for (int j = 0; j < matrix[0].length - 1; j++) {
if (matrix[i][j] != matrix[i + 1][j + 1]) {
return false;
}
}
}
return true;
}
}
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Javascript Programming
Code -
Javascript Programming
const isToeplitzMatrix = function(matrix) {
for (let r = 0; r < matrix.length; r++) {
for (let c = 0; c < matrix[0].length; c++) {
if (r > 0 && c > 0 && matrix[r - 1][c - 1] !== matrix[r][c]) {
return false;
}
}
}
return true;
};
console.log(isToeplitzMatrix([[1, 2, 3, 4], [5, 1, 2, 3], [9, 5, 1, 2]]));
console.log(isToeplitzMatrix([[1, 2], [2, 2]]));
Copy The Code &
Try With Live Editor
Input
Output
#4 Code Example with Python Programming
Code -
Python Programming
class Solution:
def isToeplitzMatrix(self, matrix):
return all(matrix[i][j] == matrix[i - 1][j - 1] for i in range(1, len(matrix)) for j in range(1, len(matrix[0])))
Copy The Code &
Try With Live Editor
Input
Output
#5 Code Example with C# Programming
Code -
C# Programming
namespace LeetCode
{
public class _0766_ToeplitzMatrix
{
public bool IsToeplitzMatrix(int[][] matrix)
{
var row = matrix.Length;
var col = matrix[0].Length;
for (int i = 1; i < row; i++)
for (int j = 1; j < col; j++)
{
if (i > 0 && j > 0 && matrix[i - 1][j - 1] != matrix[i][j])
return false;
}
return true;
}
}
}
Copy The Code &
Try With Live Editor
Input
Output