Algorithm
Problem Name: 590. N-ary Tree Postorder Traversal
Given the root
of an n-ary tree, return the postorder traversal of its nodes' values.
Nary-Tree input serialization is represented in their level order traversal. Each group of children is separated by the null value (See examples)
Example 1:
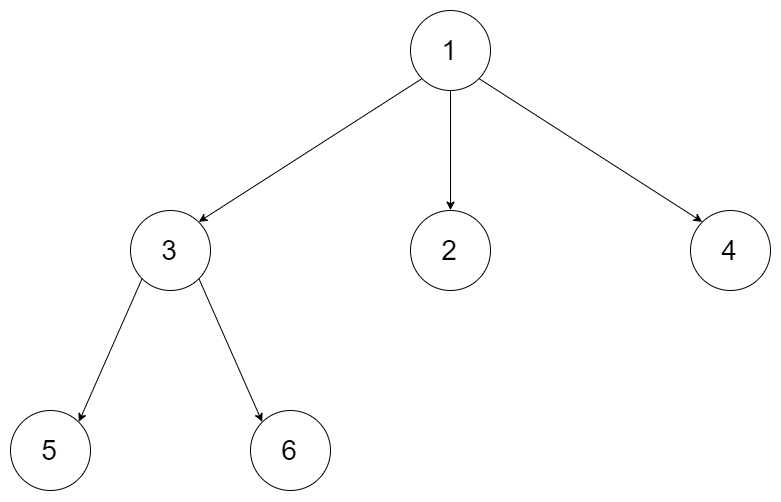
Input: root = [1,null,3,2,4,null,5,6] Output: [5,6,3,2,4,1]
Example 2:
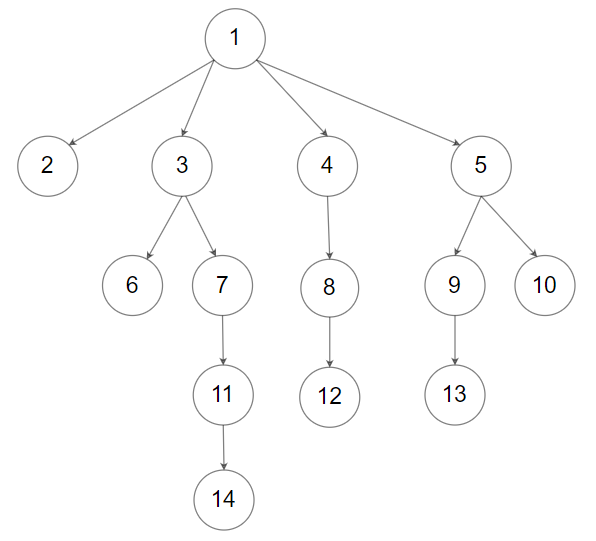
Input: root = [1,null,2,3,4,5,null,null,6,7,null,8,null,9,10,null,null,11,null,12,null,13,null,null,14] Output: [2,6,14,11,7,3,12,8,4,13,9,10,5,1]
Constraints:
- The number of nodes in the tree is in the range
[0, 104]
. 0 <= Node.val <= 104
- The height of the n-ary tree is less than or equal to
1000
.
Code Examples
#1 Code Example with Java Programming
Code -
Java Programming
class Solution {
public List postorder(Node root) {
List result = new ArrayList<>();
helper(root, result);
return result;
}
private void helper(Node root, List < Integer> result) {
if (root == null) {
return;
}
for (Node child : root.children) {
helper(child, result);
}
result.add(root.val);
}
}
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Javascript Programming
Code -
Javascript Programming
const postorder = function(root) {
const res = []
traverse(root, res)
return res
};
function traverse(node, res) {
if(node == null) return
for(let i = 0; i < node.children.length; i++) {
traverse(node.children[i], res)
}
res.push(node.val>
}
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with C# Programming
Code -
C# Programming
using System.Collections.Generic;
namespace LeetCode
{
public class _0590_NAryTreePostorderTraversal
{
public IList < int> Postorder(Node root)
{
var result = new List<int>();
if (root == null) return result;
var stack = new Stack < Node>();
stack.Push(root);
while (stack.Count > 0)
{
var node = stack.Pop();
result.Add(node.val);
if (node.children != null)
foreach (var child in node.children)
stack.Push(child);
}
result.Reverse();
return result;
}
public class Node
{
public int val;
public IList < Node> children;
public Node() { }
public Node(int _val)
{
val = _val;
}
public Node(int _val, IList < Node> _children)
{
val = _val;
children = _children;
}
}
}
}
Copy The Code &
Try With Live Editor
Input
Output