Algorithm
You are given an integer array height
of length n
. There are n
vertical lines drawn such that the two endpoints of the ith
line are (i, 0)
and (i, height[i])
.
Find two lines that together with the x-axis form a container, such that the container contains the most water.
Return the maximum amount of water a container can store.
Notice that you may not slant the container.
Example 1:
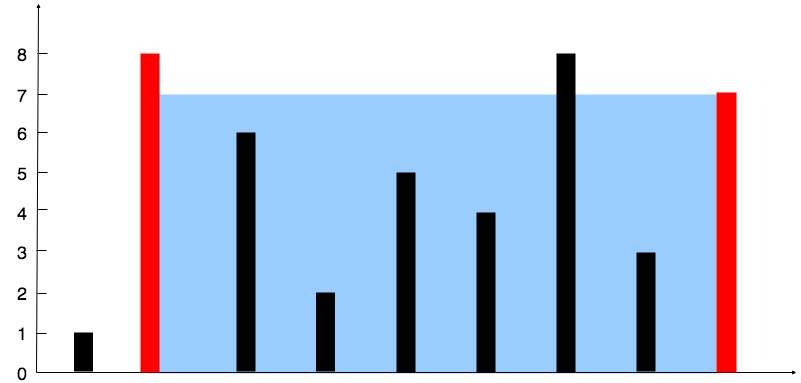
Input: height = [1,8,6,2,5,4,8,3,7] Output: 49 Explanation: The above vertical lines are represented by array [1,8,6,2,5,4,8,3,7]. In this case, the max area of water (blue section) the container can contain is 49.
Example 2:
Input: height = [1,1] Output: 1
Constraints:
n == height.length
2 <= n <= 105
0 <= height[i] <= 104
Code Examples
#1 Code Example with C Programming
Code -
C Programming
int maxArea(int* height, int heightSize) {
int l, r, x, water, max = 0;
int i, j;
#if 0
for (i = 0; i < heightSize - 1; i ++) {
if (i == 0 || height[i] > l) {
l = height[i];
for (j = i + 1; j < heightSize; j ++) {
r = height[j];
x = l < r ? l : r;
water = x * (j - i);
if (max < water) max = water;
}
}
}
#else
i = 0;
j = heightSize - 1;
while (i < j) {
l = height[i];
r = height[j];
x = l < r ? l : r;
water = x * (j - i);
if (max < water) max = water;
if (l < r) i ++;
else j --;
}
#endif
return max;
}
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with C++ Programming
Code -
C++ Programming
class Solution {
public:
int maxArea(vector<int>& height) {
int maxArea = 0, minLeft = 0, minRight = 0;
for(int i = height.size() - 1; i >= 0; i--){
if(height[i] < minRight) continue;
minLeft = 0;
for(int j = 0; j < i; j++){
if(height[j] < minLeft) continue;
maxArea = max(maxArea, min(height[i], height[j]) * (i - j));
minLeft = max(minLeft, height[j]);
}
minRight = max(minRight, height[i]>;
}
return maxArea;
}
};
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Java Programming
Code -
Java Programming
class Solution {
public int maxArea(int[] height) {
int maximumArea = 0;
int leftIdx = 0;
int rightIdx = height.length - 1;
while (leftIdx < rightIdx) {
int maxHeight = Math.min(height[leftIdx], height[rightIdx]);
int currArea = maxHeight * (rightIdx - leftIdx);
maximumArea = Math.max(currArea, maximumArea);
if (maxHeight == height[leftIdx]) {
leftIdx++;
} else {
rightIdx--;
}
}
return maximumArea;
}
}
Copy The Code &
Try With Live Editor
Input
Output
#4 Code Example with Javascript Programming
Code -
Javascript Programming
const maxArea = function(height) {
let res = 0, l = 0, r = height.length - 1
while(l < r) {
const tmp = (r - l) * Math.min(height[l], height[r])
if(tmp > res) res = tmp
if(height[l] < height[r]> l++
else r--
}
return res
};
Copy The Code &
Try With Live Editor
Input
Output