Algorithm
Problem Name: 513. Find Bottom Left Tree Value
Given the root
of a binary tree, return the leftmost value in the last row of the tree.
Example 1:
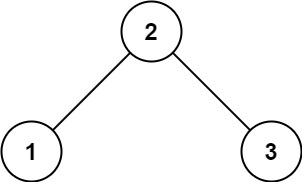
Input: root = [2,1,3] Output: 1
Example 2:
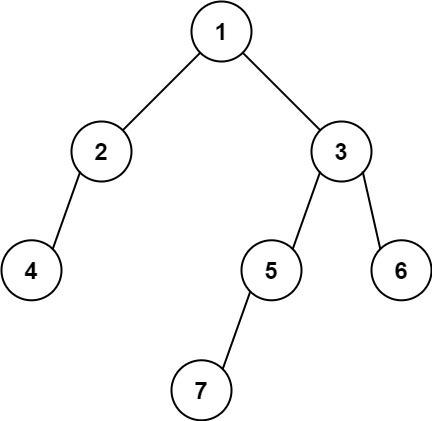
Input: root = [1,2,3,4,null,5,6,null,null,7] Output: 7
Constraints:
- The number of nodes in the tree is in the range
[1, 104]
. -231 <= Node.val <= 231 - 1
Code Examples
#1 Code Example with C++ Programming
Code -
C++ Programming
class Solution {
public:
int findBottomLeftValue(TreeNode* root) {
int res = 0, maxLevel = -1;
DFS(root, 0, maxLevel, res);
return res;
}
void DFS(TreeNode* root, int level, int& maxLevel, int& res){
if(!root) return;
DFS(root->left, level + 1, maxLevel, res);
DFS(root->right, level + 1, maxLevel, res);
if(level > maxLevel) res = root->val;
maxLevel = max(maxLevel, level);
}
};
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Java Programming
Code -
Java Programming
class Solution {
public int findBottomLeftValue(TreeNode root) {
int[] ans = {0, 0};
helper(root, 1, ans);
return ans[1];
}
private void helper(TreeNode root, int currLevel, int[] ans) {
if (root == null) {
return;
}
if (currLevel > ans[0]) {
ans[0] = currLevel;
ans[1] = root.val;
}
helper(root.left, currLevel + 1, ans);
helper(root.right, currLevel + 1, ans);
}
}
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with Javascript Programming
Code -
Javascript Programming
const findBottomLeftValue = function(root) {
const res = [];
single(root, 0, res);
return res[res.length - 1][0].val;
};
function single(node, row, arr) {
if (node == null) {
return null;
}
if (row < arr.length) {
arr[row].push(node);
} else {
arr[row] = [node];
}
single(node.left, row + 1, arr);
single(node.right, row + 1, arr);
}
Copy The Code &
Try With Live Editor
Input
Output
#4 Code Example with Python Programming
Code -
Python Programming
class Solution:
def findBottomLeftValue(self, root: TreeNode) -> int:
bfs = [root]
while bfs:
left = bfs[0].val
bfs = [child for node in bfs for child in (node.left, node.right) if child]
return left
Copy The Code &
Try With Live Editor
Input
Output
#5 Code Example with C# Programming
Code -
C# Programming
using System.Collections.Generic;
namespace LeetCode
{
public class _0513_FindBottomLeftTreeValue
{
public int FindBottomLeftValue(TreeNode root)
{
var queue = new Queue < TreeNode>();
queue.Enqueue(root);
var result = 0;
while (queue.Count > 0)
{
var size = queue.Count;
for (int i = 0; i < size; i++)
{
var node = queue.Dequeue();
if (i == 0) result = node.val;
if (node.left != null) queue.Enqueue(node.left);
if (node.right != null) queue.Enqueue(node.right);
}
}
return result;
}
}
}
Copy The Code &
Try With Live Editor
Input
Output