Algorithm
Problem Name: 1260. Shift 2D Grid
Given a 2D grid
of size m x n
and an integer k
. You need to shift the grid
k
times.
In one shift operation:
- Element at
grid[i][j]
moves togrid[i][j + 1]
. - Element at
grid[i][n - 1]
moves togrid[i + 1][0]
. - Element at
grid[m - 1][n - 1]
moves togrid[0][0]
.
Return the 2D grid after applying shift operation k
times.
Example 1:
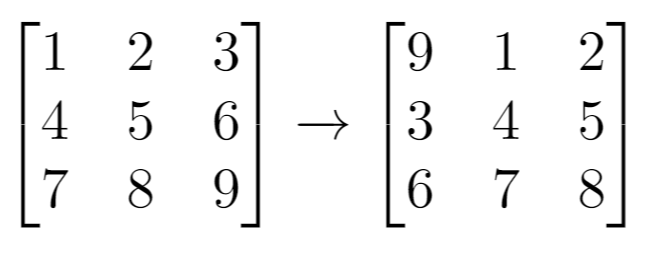
Input: grid
= [[1,2,3],[4,5,6],[7,8,9]], k = 1 Output: [[9,1,2],[3,4,5],[6,7,8]]
Example 2:
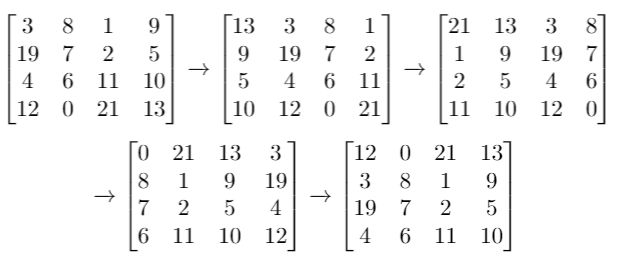
Input: grid
= [[3,8,1,9],[19,7,2,5],[4,6,11,10],[12,0,21,13]], k = 4 Output: [[12,0,21,13],[3,8,1,9],[19,7,2,5],[4,6,11,10]]
Example 3:
Input: grid
= [[1,2,3],[4,5,6],[7,8,9]], k = 9 Output: [[1,2,3],[4,5,6],[7,8,9]]
Constraints:
m == grid.length
n == grid[i].length
1 <= m <= 50
1 <= n <= 50
-1000 <= grid[i][j] <= 1000
0 <= k <= 100
Code Examples
#1 Code Example with Javascript Programming
Code -
Javascript Programming
const shiftGrid = function(grid, k) {
for(let i = 0; i < k; i++) once(grid)
return grid
};
function once(grid) {
const m = grid.length, n = grid[0].length
let last = grid[m - 1][n - 1]
for(let i = 0; i < m; i++) {
let pre = grid[i][0]
for(let j = 1; j < n; j++) {
let cur = grid[i][j]
grid[i][j] = pre
pre = cur
}
grid[i][0] = last
last = pre
}
}
Copy The Code &
Try With Live Editor
Input
Output
#2 Code Example with Python Programming
Code -
Python Programming
class Solution:
def shiftGrid(self, grid: List[List[int]], k: int) -> List[List[int]]:
chain = [r for row in grid for r in row]
k %= len(chain)
chain = chain[-k:] + chain[:-k]
return [chain[i : i + len(grid[0])] for i in range(0, len(chain), len(grid[0]))]
Copy The Code &
Try With Live Editor
Input
Output
#3 Code Example with C# Programming
Code -
C# Programming
using System.Collections.Generic;
namespace LeetCode
{
public class _1260_Shift2DGrid
{
public IList < IList<int>> ShiftGrid(int[][] grid, int k)
{
var row = grid.Length;
var col = grid[0].Length;
if (k % (row * col) == 0)
return grid;
var result = new int[row][];
for (int i = 0; i < row; i++)
result[i] = new int[col];
for (int i = 0; i < row; i++)
for (int j = 0; j < col; j++)
{
var newCol = (j + k) % col;
var wrapRows = (j + k) / col;
var newRow = (i + wrapRows) % row;
result[newRow][newCol] = grid[i][j];
}
return result;
}
}
}
Copy The Code &
Try With Live Editor
Input
Output